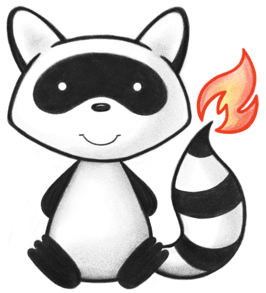
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.provider; 021 022import ca.uhn.fhir.jpa.api.dao.IFhirResourceDaoComposition; 023import ca.uhn.fhir.jpa.model.util.JpaConstants; 024import ca.uhn.fhir.model.api.annotation.Description; 025import ca.uhn.fhir.model.valueset.BundleTypeEnum; 026import ca.uhn.fhir.rest.annotation.IdParam; 027import ca.uhn.fhir.rest.annotation.Operation; 028import ca.uhn.fhir.rest.annotation.OperationParam; 029import ca.uhn.fhir.rest.annotation.Sort; 030import ca.uhn.fhir.rest.api.Constants; 031import ca.uhn.fhir.rest.api.SortSpec; 032import ca.uhn.fhir.rest.api.server.IBundleProvider; 033import ca.uhn.fhir.rest.api.server.RequestDetails; 034import ca.uhn.fhir.rest.param.DateRangeParam; 035import org.hl7.fhir.instance.model.api.IBaseResource; 036import org.hl7.fhir.instance.model.api.IIdType; 037import org.hl7.fhir.instance.model.api.IPrimitiveType; 038import org.hl7.fhir.r5.model.Composition; 039 040public abstract class BaseJpaResourceProviderComposition<T extends IBaseResource> extends BaseJpaResourceProvider<T> { 041 042 /** 043 * Composition/123/$document 044 */ 045 @Operation(name = JpaConstants.OPERATION_DOCUMENT, idempotent = true, bundleType = BundleTypeEnum.DOCUMENT) 046 public IBundleProvider getDocumentForComposition( 047 jakarta.servlet.http.HttpServletRequest theServletRequest, 048 @IdParam IIdType theId, 049 @Description( 050 formalDefinition = 051 "Results from this method are returned across multiple pages. This parameter controls the size of those pages.") 052 @OperationParam(name = Constants.PARAM_COUNT, typeName = "unsignedInt") 053 IPrimitiveType<Integer> theCount, 054 @Description( 055 formalDefinition = 056 "Results from this method are returned across multiple pages. This parameter controls the offset when fetching a page.") 057 @OperationParam(name = Constants.PARAM_OFFSET, typeName = "unsignedInt") 058 IPrimitiveType<Integer> theOffset, 059 @Description( 060 shortDefinition = 061 "Only return resources which were last updated as specified by the given range") 062 @OperationParam(name = Constants.PARAM_LASTUPDATED, min = 0, max = 1) 063 DateRangeParam theLastUpdated, 064 @Sort SortSpec theSortSpec, 065 RequestDetails theRequestDetails) { 066 067 startRequest(theServletRequest); 068 try { 069 IBundleProvider bundleProvider = ((IFhirResourceDaoComposition<Composition>) getDao()) 070 .getDocumentForComposition( 071 theServletRequest, 072 theId, 073 theCount, 074 theOffset, 075 theLastUpdated, 076 theSortSpec, 077 theRequestDetails); 078 return bundleProvider; 079 } finally { 080 endRequest(theServletRequest); 081 } 082 } 083}