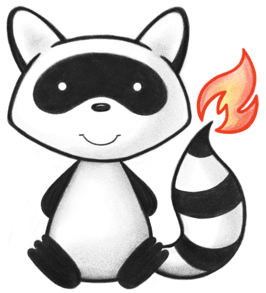
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.provider; 021 022import ca.uhn.fhir.jpa.api.dao.IFhirResourceDaoEncounter; 023import ca.uhn.fhir.jpa.model.util.JpaConstants; 024import ca.uhn.fhir.model.api.annotation.Description; 025import ca.uhn.fhir.model.dstu2.resource.Encounter; 026import ca.uhn.fhir.model.valueset.BundleTypeEnum; 027import ca.uhn.fhir.rest.annotation.IdParam; 028import ca.uhn.fhir.rest.annotation.Operation; 029import ca.uhn.fhir.rest.annotation.OperationParam; 030import ca.uhn.fhir.rest.annotation.Sort; 031import ca.uhn.fhir.rest.api.Constants; 032import ca.uhn.fhir.rest.api.SortSpec; 033import ca.uhn.fhir.rest.api.server.IBundleProvider; 034import ca.uhn.fhir.rest.param.DateRangeParam; 035 036public abstract class BaseJpaResourceProviderEncounterDstu2 extends BaseJpaResourceProvider<Encounter> { 037 038 /** 039 * Encounter/123/$everything 040 */ 041 @Operation(name = JpaConstants.OPERATION_EVERYTHING, idempotent = true, bundleType = BundleTypeEnum.SEARCHSET) 042 public IBundleProvider EncounterInstanceEverything( 043 jakarta.servlet.http.HttpServletRequest theServletRequest, 044 @IdParam ca.uhn.fhir.model.primitive.IdDt theId, 045 @Description( 046 formalDefinition = 047 "Results from this method are returned across multiple pages. This parameter controls the size of those pages.") 048 @OperationParam(name = Constants.PARAM_COUNT) 049 ca.uhn.fhir.model.primitive.UnsignedIntDt theCount, 050 @Description( 051 formalDefinition = 052 "Results from this method are returned across multiple pages. This parameter controls the offset when fetching a page.") 053 @OperationParam(name = Constants.PARAM_OFFSET) 054 ca.uhn.fhir.model.primitive.UnsignedIntDt theOffset, 055 @Description( 056 shortDefinition = 057 "Only return resources which were last updated as specified by the given range") 058 @OperationParam(name = Constants.PARAM_LASTUPDATED, min = 0, max = 1) 059 DateRangeParam theLastUpdated, 060 @Sort SortSpec theSortSpec) { 061 062 startRequest(theServletRequest); 063 try { 064 return ((IFhirResourceDaoEncounter<Encounter>) getDao()) 065 .encounterInstanceEverything( 066 theServletRequest, theId, theCount, theOffset, theLastUpdated, theSortSpec); 067 } finally { 068 endRequest(theServletRequest); 069 } 070 } 071 072 /** 073 * /Encounter/$everything 074 */ 075 @Operation(name = JpaConstants.OPERATION_EVERYTHING, idempotent = true, bundleType = BundleTypeEnum.SEARCHSET) 076 public IBundleProvider EncounterTypeEverything( 077 jakarta.servlet.http.HttpServletRequest theServletRequest, 078 @Description( 079 formalDefinition = 080 "Results from this method are returned across multiple pages. This parameter controls the size of those pages.") 081 @OperationParam(name = Constants.PARAM_COUNT) 082 ca.uhn.fhir.model.primitive.UnsignedIntDt theCount, 083 @Description( 084 formalDefinition = 085 "Results from this method are returned across multiple pages. This parameter controls the offset when fetching a page.") 086 @OperationParam(name = Constants.PARAM_OFFSET) 087 ca.uhn.fhir.model.primitive.UnsignedIntDt theOffset, 088 @Description( 089 shortDefinition = 090 "Only return resources which were last updated as specified by the given range") 091 @OperationParam(name = Constants.PARAM_LASTUPDATED, min = 0, max = 1) 092 DateRangeParam theLastUpdated, 093 @Sort SortSpec theSortSpec) { 094 095 startRequest(theServletRequest); 096 try { 097 return ((IFhirResourceDaoEncounter<Encounter>) getDao()) 098 .encounterTypeEverything(theServletRequest, theCount, theOffset, theLastUpdated, theSortSpec); 099 } finally { 100 endRequest(theServletRequest); 101 } 102 } 103}