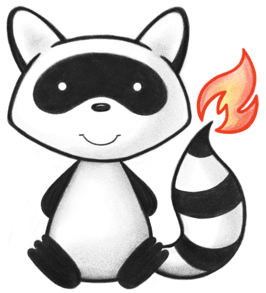
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.provider; 021 022import ca.uhn.fhir.jpa.api.dao.IFhirResourceDaoObservation; 023import ca.uhn.fhir.jpa.model.util.JpaConstants; 024import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 025import ca.uhn.fhir.model.api.annotation.Description; 026import ca.uhn.fhir.model.valueset.BundleTypeEnum; 027import ca.uhn.fhir.rest.annotation.Operation; 028import ca.uhn.fhir.rest.annotation.OperationParam; 029import ca.uhn.fhir.rest.annotation.RawParam; 030import ca.uhn.fhir.rest.api.Constants; 031import ca.uhn.fhir.rest.api.server.IBundleProvider; 032import ca.uhn.fhir.rest.param.DateAndListParam; 033import ca.uhn.fhir.rest.param.ReferenceAndListParam; 034import ca.uhn.fhir.rest.param.TokenAndListParam; 035import org.hl7.fhir.instance.model.api.IBaseResource; 036import org.hl7.fhir.instance.model.api.IPrimitiveType; 037 038import java.util.List; 039import java.util.Map; 040 041public abstract class BaseJpaResourceProviderObservation<T extends IBaseResource> extends BaseJpaResourceProvider<T> { 042 043 /** 044 * Observation/$lastn 045 */ 046 @Operation(name = JpaConstants.OPERATION_LASTN, idempotent = true, bundleType = BundleTypeEnum.SEARCHSET) 047 public IBundleProvider observationLastN( 048 jakarta.servlet.http.HttpServletRequest theServletRequest, 049 jakarta.servlet.http.HttpServletResponse theServletResponse, 050 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 051 @Description( 052 formalDefinition = 053 "Results from this method are returned across multiple pages. This parameter controls the size of those pages.") 054 @OperationParam(name = Constants.PARAM_COUNT, typeName = "unsignedInt") 055 IPrimitiveType<Integer> theCount, 056 @Description(shortDefinition = "The classification of the type of observation") 057 @OperationParam(name = "category") 058 TokenAndListParam theCategory, 059 @Description(shortDefinition = "The code of the observation type") @OperationParam(name = "code") 060 TokenAndListParam theCode, 061 @Description(shortDefinition = "The effective date of the observation") @OperationParam(name = "date") 062 DateAndListParam theDate, 063 @Description(shortDefinition = "The subject that the observation is about (if patient)") 064 @OperationParam(name = "patient") 065 ReferenceAndListParam thePatient, 066 @Description(shortDefinition = "The subject that the observation is about") 067 @OperationParam(name = "subject") 068 ReferenceAndListParam theSubject, 069 @Description(shortDefinition = "The maximum number of observations to return for each observation code") 070 @OperationParam(name = "max", typeName = "integer", min = 0, max = 1) 071 IPrimitiveType<Integer> theMax, 072 @RawParam Map<String, List<String>> theAdditionalRawParams) { 073 startRequest(theServletRequest); 074 try { 075 SearchParameterMap paramMap = new SearchParameterMap(); 076 paramMap.add(org.hl7.fhir.r4.model.Observation.SP_CATEGORY, theCategory); 077 paramMap.add(org.hl7.fhir.r4.model.Observation.SP_CODE, theCode); 078 paramMap.add(org.hl7.fhir.r4.model.Observation.SP_DATE, theDate); 079 if (thePatient != null) { 080 paramMap.add(org.hl7.fhir.r4.model.Observation.SP_PATIENT, thePatient); 081 } 082 if (theSubject != null) { 083 paramMap.add(org.hl7.fhir.r4.model.Observation.SP_SUBJECT, theSubject); 084 } 085 if (theMax != null) { 086 paramMap.setLastNMax(theMax.getValue()); 087 088 /** 089 * The removal of the original raw parameter is required as every implementing class 090 * has the "Observation" resource class defined. For this resource, the max parameter 091 * is not supported and thus has to be removed before the use of "translateRawParameters". 092 */ 093 theAdditionalRawParams.remove("max"); 094 } 095 if (theCount != null) { 096 paramMap.setCount(theCount.getValue()); 097 } 098 099 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 100 101 return ((IFhirResourceDaoObservation<?>) getDao()) 102 .observationsLastN(paramMap, theRequestDetails, theServletResponse); 103 } finally { 104 endRequest(theServletRequest); 105 } 106 } 107}