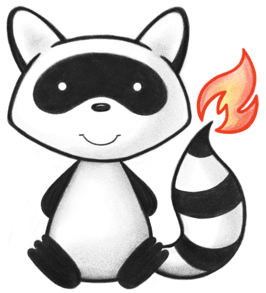
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.provider; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.jpa.api.dao.IFhirResourceDaoStructureDefinition; 024import ca.uhn.fhir.jpa.model.util.JpaConstants; 025import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 026import ca.uhn.fhir.rest.annotation.IdParam; 027import ca.uhn.fhir.rest.annotation.Operation; 028import ca.uhn.fhir.rest.annotation.OperationParam; 029import ca.uhn.fhir.rest.api.server.IBundleProvider; 030import ca.uhn.fhir.rest.api.server.RequestDetails; 031import ca.uhn.fhir.rest.param.UriParam; 032import ca.uhn.fhir.rest.server.exceptions.ResourceNotFoundException; 033import ca.uhn.fhir.util.ValidateUtil; 034import org.hl7.fhir.instance.model.api.IBaseResource; 035import org.hl7.fhir.instance.model.api.IIdType; 036import org.hl7.fhir.instance.model.api.IPrimitiveType; 037 038public abstract class BaseJpaResourceProviderStructureDefinition<T extends IBaseResource> 039 extends BaseJpaResourceProvider<T> { 040 041 /** 042 * <code>$snapshot</code> operation 043 */ 044 @Operation(name = JpaConstants.OPERATION_SNAPSHOT, idempotent = true) 045 public IBaseResource snapshot( 046 @IdParam(optional = true) IIdType theId, 047 @OperationParam(name = "definition", typeName = "StructureDefinition") IBaseResource theStructureDefinition, 048 @OperationParam(name = "url", typeName = "string") IPrimitiveType<String> theUrl, 049 RequestDetails theRequestDetails) { 050 051 ValidateUtil.exactlyOneNotNullOrThrowInvalidRequestException( 052 new Object[] {theId, theStructureDefinition, theUrl}, 053 "Must supply either an ID or a StructureDefinition or a URL (but not more than one of these things)"); 054 055 IBaseResource sd; 056 IFhirResourceDaoStructureDefinition dao = getDao(); 057 if (theId == null && theStructureDefinition != null && theUrl == null) { 058 sd = theStructureDefinition; 059 } else if (theId != null && theStructureDefinition == null) { 060 sd = dao.read(theId, theRequestDetails); 061 } else { 062 SearchParameterMap map = new SearchParameterMap(); 063 map.setLoadSynchronousUpTo(2); 064 map.add(org.hl7.fhir.r4.model.StructureDefinition.SP_URL, new UriParam(theUrl.getValue())); 065 IBundleProvider outcome = dao.search(map, theRequestDetails); 066 Integer numResults = outcome.size(); 067 assert numResults != null; 068 if (numResults == 0) { 069 throw new ResourceNotFoundException( 070 Msg.code(1162) + "No StructureDefiniton found with url = '" + theUrl.getValue() + "'"); 071 } 072 sd = outcome.getResources(0, 1).get(0); 073 } 074 075 return dao.generateSnapshot(sd, null, null, null); 076 } 077 078 @Override 079 public IFhirResourceDaoStructureDefinition<T> getDao() { 080 return (IFhirResourceDaoStructureDefinition<T>) super.getDao(); 081 } 082}