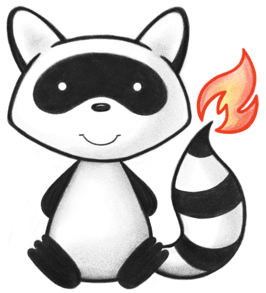
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.provider; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.jpa.api.dao.DaoRegistry; 025import ca.uhn.fhir.jpa.api.dao.IFhirResourceDao; 026import ca.uhn.fhir.jpa.patch.FhirPatch; 027import ca.uhn.fhir.model.api.annotation.Description; 028import ca.uhn.fhir.rest.annotation.IdParam; 029import ca.uhn.fhir.rest.annotation.Operation; 030import ca.uhn.fhir.rest.annotation.OperationParam; 031import ca.uhn.fhir.rest.api.server.RequestDetails; 032import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 033import ca.uhn.fhir.rest.server.exceptions.ResourceNotFoundException; 034import ca.uhn.fhir.rest.server.provider.ProviderConstants; 035import com.google.common.base.Objects; 036import jakarta.annotation.Nonnull; 037import org.hl7.fhir.instance.model.api.IBaseParameters; 038import org.hl7.fhir.instance.model.api.IBaseResource; 039import org.hl7.fhir.instance.model.api.IIdType; 040import org.hl7.fhir.instance.model.api.IPrimitiveType; 041import org.slf4j.Logger; 042import org.slf4j.LoggerFactory; 043import org.springframework.beans.factory.annotation.Autowired; 044 045public class DiffProvider { 046 private static final Logger ourLog = LoggerFactory.getLogger(DiffProvider.class); 047 048 @Autowired 049 private FhirContext myContext; 050 051 @Autowired 052 private DaoRegistry myDaoRegistry; 053 054 @Description( 055 value = 056 "This operation examines two resource versions (can be two versions of the same resource, or two different resources) and generates a FHIR Patch document showing the differences.", 057 shortDefinition = "Comparte two resources or two versions of a single resource") 058 @Operation(name = ProviderConstants.DIFF_OPERATION_NAME, global = true, idempotent = true) 059 public IBaseParameters diff( 060 @IdParam IIdType theResourceId, 061 @Description(value = "The resource ID and version to diff from", example = "Patient/example/version/1") 062 @OperationParam( 063 name = ProviderConstants.DIFF_FROM_VERSION_PARAMETER, 064 typeName = "string", 065 min = 0, 066 max = 1) 067 IPrimitiveType<?> theFromVersion, 068 @Description( 069 value = "Should differences in the Resource.meta element be included in the diff", 070 example = "false") 071 @OperationParam( 072 name = ProviderConstants.DIFF_INCLUDE_META_PARAMETER, 073 typeName = "boolean", 074 min = 0, 075 max = 1) 076 IPrimitiveType<Boolean> theIncludeMeta, 077 RequestDetails theRequestDetails) { 078 079 IFhirResourceDao<?> dao = myDaoRegistry.getResourceDao(theResourceId.getResourceType()); 080 IBaseResource targetResource = dao.read(theResourceId, theRequestDetails); 081 IBaseResource sourceResource = null; 082 083 Long versionId = targetResource.getIdElement().getVersionIdPartAsLong(); 084 085 if (theFromVersion == null || theFromVersion.getValueAsString() == null) { 086 087 // If no explicit from version is specified, find the next previous existing version 088 while (--versionId > 0L && sourceResource == null) { 089 IIdType nextVersionedId = theResourceId.withVersion(Long.toString(versionId)); 090 try { 091 sourceResource = dao.read(nextVersionedId, theRequestDetails); 092 } catch (ResourceNotFoundException e) { 093 ourLog.trace("Resource version {} can not be found, most likely it was expunged", nextVersionedId); 094 } 095 } 096 097 } else { 098 099 long fromVersion = Long.parseLong(theFromVersion.getValueAsString()); 100 sourceResource = dao.read(theResourceId.withVersion(Long.toString(fromVersion)), theRequestDetails); 101 } 102 103 FhirPatch fhirPatch = newPatch(theIncludeMeta); 104 return fhirPatch.diff(sourceResource, targetResource); 105 } 106 107 @Description( 108 "This operation examines two resource versions (can be two versions of the same resource, or two different resources) and generates a FHIR Patch document showing the differences.") 109 @Operation(name = ProviderConstants.DIFF_OPERATION_NAME, idempotent = true) 110 public IBaseParameters diff( 111 @Description(value = "The resource ID and version to diff from", example = "Patient/example/version/1") 112 @OperationParam(name = ProviderConstants.DIFF_FROM_PARAMETER, typeName = "id", min = 1, max = 1) 113 IIdType theFromVersion, 114 @Description(value = "The resource ID and version to diff to", example = "Patient/example/version/2") 115 @OperationParam(name = ProviderConstants.DIFF_TO_PARAMETER, typeName = "id", min = 1, max = 1) 116 IIdType theToVersion, 117 @Description( 118 value = "Should differences in the Resource.meta element be included in the diff", 119 example = "false") 120 @OperationParam( 121 name = ProviderConstants.DIFF_INCLUDE_META_PARAMETER, 122 typeName = "boolean", 123 min = 0, 124 max = 1) 125 IPrimitiveType<Boolean> theIncludeMeta, 126 RequestDetails theRequestDetails) { 127 128 if (!Objects.equal(theFromVersion.getResourceType(), theToVersion.getResourceType())) { 129 String msg = myContext.getLocalizer().getMessage(DiffProvider.class, "cantDiffDifferentTypes"); 130 throw new InvalidRequestException(Msg.code(1129) + msg); 131 } 132 133 IFhirResourceDao<?> dao = myDaoRegistry.getResourceDao(theFromVersion.getResourceType()); 134 IBaseResource sourceResource = dao.read(theFromVersion, theRequestDetails); 135 IBaseResource targetResource = dao.read(theToVersion, theRequestDetails); 136 137 FhirPatch fhirPatch = newPatch(theIncludeMeta); 138 return fhirPatch.diff(sourceResource, targetResource); 139 } 140 141 @Nonnull 142 public FhirPatch newPatch(IPrimitiveType<Boolean> theIncludeMeta) { 143 FhirPatch fhirPatch = new FhirPatch(myContext); 144 fhirPatch.setIncludePreviousValueInDiff(true); 145 146 if (theIncludeMeta != null && theIncludeMeta.getValue()) { 147 ourLog.trace("Including resource metadata in patch"); 148 } else { 149 fhirPatch.addIgnorePath("*.meta"); 150 } 151 152 return fhirPatch; 153 } 154}