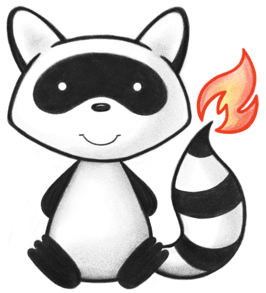
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.provider; 021 022import ca.uhn.fhir.jpa.api.dao.IFhirSystemDao; 023import ca.uhn.fhir.jpa.model.util.JpaConstants; 024import ca.uhn.fhir.model.api.annotation.Description; 025import ca.uhn.fhir.rest.annotation.Operation; 026import ca.uhn.fhir.rest.annotation.OperationParam; 027import ca.uhn.fhir.rest.api.server.RequestDetails; 028import jakarta.servlet.http.HttpServletRequest; 029import org.hl7.fhir.instance.model.api.IBaseBundle; 030import org.springframework.beans.factory.annotation.Autowired; 031 032import static ca.uhn.fhir.jpa.provider.BaseJpaProvider.endRequest; 033import static ca.uhn.fhir.jpa.provider.BaseJpaProvider.startRequest; 034 035public class ProcessMessageProvider { 036 037 @Autowired 038 private IFhirSystemDao<?, ?> mySystemDao; 039 040 /** 041 * $process-message 042 */ 043 @Description("Accept a FHIR Message Bundle for processing") 044 @Operation(name = JpaConstants.OPERATION_PROCESS_MESSAGE, idempotent = false) 045 public IBaseBundle processMessage( 046 HttpServletRequest theServletRequest, 047 RequestDetails theRequestDetails, 048 @OperationParam(name = "content", min = 1, max = 1, typeName = "Bundle") 049 @Description( 050 shortDefinition = 051 "The message to process (or, if using asynchronous messaging, it may be a response message to accept)") 052 IBaseBundle theMessageToProcess) { 053 054 startRequest(theServletRequest); 055 try { 056 return mySystemDao.processMessage(theRequestDetails, theMessageToProcess); 057 } finally { 058 endRequest(theServletRequest); 059 } 060 } 061}