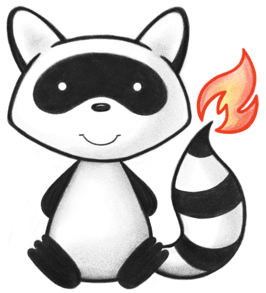
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.provider; 021 022import ca.uhn.fhir.context.support.IValidationSupport; 023import ca.uhn.fhir.jpa.dao.JpaResourceDaoCodeSystem; 024import ca.uhn.fhir.jpa.model.util.JpaConstants; 025import ca.uhn.fhir.rest.annotation.IdParam; 026import ca.uhn.fhir.rest.annotation.Operation; 027import ca.uhn.fhir.rest.annotation.OperationParam; 028import ca.uhn.fhir.rest.api.server.RequestDetails; 029import ca.uhn.fhir.util.FhirTerser; 030import jakarta.servlet.http.HttpServletRequest; 031import org.hl7.fhir.instance.model.api.IBaseCoding; 032import org.hl7.fhir.instance.model.api.IBaseParameters; 033import org.hl7.fhir.instance.model.api.IBaseResource; 034import org.hl7.fhir.instance.model.api.IIdType; 035import org.hl7.fhir.instance.model.api.IPrimitiveType; 036import org.springframework.beans.factory.annotation.Autowired; 037 038import java.util.List; 039 040import static ca.uhn.fhir.jpa.provider.BaseJpaResourceProviderCodeSystem.applyVersionToSystem; 041 042public class ValueSetOperationProviderDstu2 extends ValueSetOperationProvider { 043 044 @Autowired 045 private IValidationSupport myValidationSupport; 046 047 /** 048 * Alternate expand implementation since DSTU2 uses "identifier" instead of "url" as a parameter. 049 */ 050 @Operation(name = JpaConstants.OPERATION_EXPAND, idempotent = true, typeName = "ValueSet") 051 public IBaseResource expand( 052 HttpServletRequest theServletRequest, 053 @IdParam(optional = true) IIdType theId, 054 @OperationParam(name = "valueSet", min = 0, max = 1) IBaseResource theValueSet, 055 @OperationParam(name = "url", min = 0, max = 1, typeName = "uri") IPrimitiveType<String> theUrl, 056 @OperationParam(name = "identifier", min = 0, max = 1, typeName = "uri") 057 IPrimitiveType<String> theIdentifier, 058 @OperationParam(name = "valueSetVersion", min = 0, max = 1, typeName = "string") 059 IPrimitiveType<String> theValueSetVersion, 060 @OperationParam(name = "filter", min = 0, max = 1, typeName = "string") IPrimitiveType<String> theFilter, 061 @OperationParam(name = "context", min = 0, max = 1, typeName = "string") IPrimitiveType<String> theContext, 062 @OperationParam(name = "contextDirection", min = 0, max = 1, typeName = "string") 063 IPrimitiveType<String> theContextDirection, 064 @OperationParam(name = "offset", min = 0, max = 1, typeName = "integer") IPrimitiveType<Integer> theOffset, 065 @OperationParam(name = "count", min = 0, max = 1, typeName = "integer") IPrimitiveType<Integer> theCount, 066 @OperationParam( 067 name = JpaConstants.OPERATION_EXPAND_PARAM_DISPLAY_LANGUAGE, 068 min = 0, 069 max = 1, 070 typeName = "code") 071 IPrimitiveType<String> theDisplayLanguage, 072 @OperationParam( 073 name = JpaConstants.OPERATION_EXPAND_PARAM_INCLUDE_HIERARCHY, 074 min = 0, 075 max = 1, 076 typeName = "boolean") 077 IPrimitiveType<Boolean> theIncludeHierarchy, 078 RequestDetails theRequestDetails) { 079 080 IPrimitiveType<String> url = theUrl; 081 if (theIdentifier != null) { 082 url = theIdentifier; 083 } 084 085 startRequest(theServletRequest); 086 try { 087 088 return getDao().expand( 089 theId, 090 theValueSet, 091 url, 092 theValueSetVersion, 093 theFilter, 094 theContext, 095 theContextDirection, 096 theOffset, 097 theCount, 098 theDisplayLanguage, 099 theIncludeHierarchy, 100 theRequestDetails); 101 102 } finally { 103 endRequest(theServletRequest); 104 } 105 } 106 107 /** 108 * $lookup operation - This is on CodeSystem after DSTU2 but on ValueSet in DSTU2 109 */ 110 @SuppressWarnings("unchecked") 111 @Operation( 112 name = JpaConstants.OPERATION_LOOKUP, 113 idempotent = true, 114 typeName = "ValueSet", 115 returnParameters = { 116 @OperationParam(name = "name", typeName = "string", min = 1), 117 @OperationParam(name = "version", typeName = "string", min = 0), 118 @OperationParam(name = "display", typeName = "string", min = 1), 119 @OperationParam(name = "abstract", typeName = "boolean", min = 1), 120 }) 121 public IBaseParameters lookup( 122 HttpServletRequest theServletRequest, 123 @OperationParam(name = "code", min = 0, max = 1, typeName = "code") IPrimitiveType<String> theCode, 124 @OperationParam(name = "system", min = 0, max = 1, typeName = "uri") IPrimitiveType<String> theSystem, 125 @OperationParam(name = "coding", min = 0, max = 1, typeName = "Coding") IBaseCoding theCoding, 126 @OperationParam(name = "version", min = 0, max = 1, typeName = "string") IPrimitiveType<String> theVersion, 127 @OperationParam(name = "displayLanguage", min = 0, max = 1, typeName = "code") 128 IPrimitiveType<String> theDisplayLanguage, 129 @OperationParam(name = "property", min = 0, max = OperationParam.MAX_UNLIMITED, typeName = "code") 130 List<IPrimitiveType<String>> thePropertyNames, 131 RequestDetails theRequestDetails) { 132 133 startRequest(theServletRequest); 134 try { 135 IValidationSupport.LookupCodeResult result; 136 applyVersionToSystem(theSystem, theVersion); 137 138 FhirTerser terser = getContext().newTerser(); 139 result = JpaResourceDaoCodeSystem.doLookupCode( 140 getContext(), 141 terser, 142 myValidationSupport, 143 theCode, 144 theSystem, 145 theCoding, 146 theDisplayLanguage, 147 thePropertyNames); 148 result.throwNotFoundIfAppropriate(); 149 return result.toParameters(theRequestDetails.getFhirContext(), thePropertyNames); 150 } finally { 151 endRequest(theServletRequest); 152 } 153 } 154}