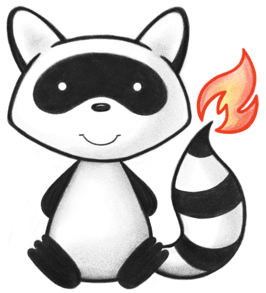
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.provider.merge; 021 022import ca.uhn.fhir.batch2.jobs.chunk.FhirIdJson; 023import ca.uhn.fhir.batch2.jobs.merge.MergeJobParameters; 024import ca.uhn.fhir.context.FhirContext; 025import ca.uhn.fhir.interceptor.model.RequestPartitionId; 026import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 027import ca.uhn.fhir.util.CanonicalIdentifier; 028import org.hl7.fhir.instance.model.api.IBaseReference; 029import org.hl7.fhir.instance.model.api.IBaseResource; 030import org.hl7.fhir.r4.model.Patient; 031 032import java.util.List; 033 034/** 035 * See <a href="https://build.fhir.org/patient-operation-merge.html">Patient $merge spec</a> 036 */ 037public abstract class BaseMergeOperationInputParameters { 038 039 private List<CanonicalIdentifier> mySourceResourceIdentifiers; 040 private List<CanonicalIdentifier> myTargetResourceIdentifiers; 041 private IBaseReference mySourceResource; 042 private IBaseReference myTargetResource; 043 private boolean myPreview; 044 private boolean myDeleteSource; 045 private IBaseResource myResultResource; 046 private final int myResourceLimit; 047 048 protected BaseMergeOperationInputParameters(int theResourceLimit) { 049 myResourceLimit = theResourceLimit; 050 } 051 052 public abstract String getSourceResourceParameterName(); 053 054 public abstract String getTargetResourceParameterName(); 055 056 public abstract String getSourceIdentifiersParameterName(); 057 058 public abstract String getTargetIdentifiersParameterName(); 059 060 public abstract String getResultResourceParameterName(); 061 062 public List<CanonicalIdentifier> getSourceIdentifiers() { 063 return mySourceResourceIdentifiers; 064 } 065 066 public boolean hasAtLeastOneSourceIdentifier() { 067 return mySourceResourceIdentifiers != null && !mySourceResourceIdentifiers.isEmpty(); 068 } 069 070 public void setSourceResourceIdentifiers(List<CanonicalIdentifier> theSourceIdentifiers) { 071 this.mySourceResourceIdentifiers = theSourceIdentifiers; 072 } 073 074 public List<CanonicalIdentifier> getTargetIdentifiers() { 075 return myTargetResourceIdentifiers; 076 } 077 078 public boolean hasAtLeastOneTargetIdentifier() { 079 return myTargetResourceIdentifiers != null && !myTargetResourceIdentifiers.isEmpty(); 080 } 081 082 public void setTargetResourceIdentifiers(List<CanonicalIdentifier> theTargetIdentifiers) { 083 this.myTargetResourceIdentifiers = theTargetIdentifiers; 084 } 085 086 public boolean getPreview() { 087 return myPreview; 088 } 089 090 public void setPreview(boolean thePreview) { 091 this.myPreview = thePreview; 092 } 093 094 public boolean getDeleteSource() { 095 return myDeleteSource; 096 } 097 098 public void setDeleteSource(boolean theDeleteSource) { 099 this.myDeleteSource = theDeleteSource; 100 } 101 102 public IBaseResource getResultResource() { 103 return myResultResource; 104 } 105 106 public void setResultResource(IBaseResource theResultResource) { 107 this.myResultResource = theResultResource; 108 } 109 110 public IBaseReference getSourceResource() { 111 return mySourceResource; 112 } 113 114 public void setSourceResource(IBaseReference theSourceResource) { 115 this.mySourceResource = theSourceResource; 116 } 117 118 public IBaseReference getTargetResource() { 119 return myTargetResource; 120 } 121 122 public void setTargetResource(IBaseReference theTargetResource) { 123 this.myTargetResource = theTargetResource; 124 } 125 126 public int getResourceLimit() { 127 return myResourceLimit; 128 } 129 130 public MergeJobParameters asMergeJobParameters( 131 FhirContext theFhirContext, 132 JpaStorageSettings theStorageSettings, 133 Patient theSourceResource, 134 Patient theTargetResource, 135 RequestPartitionId thePartitionId) { 136 MergeJobParameters retval = new MergeJobParameters(); 137 if (getResultResource() != null) { 138 retval.setResultResource(theFhirContext.newJsonParser().encodeResourceToString(getResultResource())); 139 } 140 retval.setDeleteSource(getDeleteSource()); 141 retval.setBatchSize(theStorageSettings.getDefaultTransactionEntriesForWrite()); 142 retval.setSourceId(new FhirIdJson(theSourceResource.getIdElement().toVersionless())); 143 retval.setTargetId(new FhirIdJson(theTargetResource.getIdElement().toVersionless())); 144 retval.setPartitionId(thePartitionId); 145 return retval; 146 } 147}