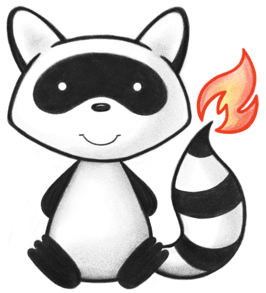
001 002package ca.uhn.fhir.jpa.rp.dstu2; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import ca.uhn.fhir.model.dstu2.composite.*; 012import ca.uhn.fhir.model.dstu2.resource.*; // 013import ca.uhn.fhir.rest.annotation.*; 014import ca.uhn.fhir.rest.param.*; 015import ca.uhn.fhir.rest.api.SortSpec; 016import ca.uhn.fhir.rest.api.SummaryEnum; 017import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 018import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 019 020public class AccountResourceProvider extends 021 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<Account> 022 { 023 024 @Override 025 public Class<Account> getResourceType() { 026 return Account.class; 027 } 028 029 @Search(allowUnknownParams=true) 030 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 031 jakarta.servlet.http.HttpServletRequest theServletRequest, 032 jakarta.servlet.http.HttpServletResponse theServletResponse, 033 034 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 035 036 @Description(shortDefinition="Search the contents of the resource's data using a filter") 037 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 038 StringAndListParam theFtFilter, 039 040 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 041 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 042 StringAndListParam theFtContent, 043 044 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 045 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 046 StringAndListParam theFtText, 047 048 @Description(shortDefinition="Search for resources which have the given tag") 049 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 050 TokenAndListParam theSearchForTag, 051 052 @Description(shortDefinition="Search for resources which have the given security labels") 053 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 054 TokenAndListParam theSearchForSecurity, 055 056 @Description(shortDefinition="Search for resources which have the given profile") 057 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 058 UriAndListParam theSearchForProfile, 059 060 @Description(shortDefinition="Search the contents of the resource's data using a list") 061 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 062 StringAndListParam theList, 063 064 @Description(shortDefinition="The language of the resource") 065 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 066 TokenAndListParam theResourceLanguage, 067 068 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 069 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 070 UriAndListParam theSearchForSource, 071 072 @Description(shortDefinition="Return resources linked to by the given target") 073 @OptionalParam(name="_has") 074 HasAndListParam theHas, 075 076 077 078 @Description(shortDefinition="The ID of the resource") 079 @OptionalParam(name="_id") 080 StringAndListParam the_id, 081 082 083 @Description(shortDefinition="") 084 @OptionalParam(name="balance") 085 QuantityAndListParam theBalance, 086 087 088 @Description(shortDefinition="") 089 @OptionalParam(name="identifier") 090 TokenAndListParam theIdentifier, 091 092 093 @Description(shortDefinition="") 094 @OptionalParam(name="name") 095 StringAndListParam theName, 096 097 098 @Description(shortDefinition="") 099 @OptionalParam(name="owner", targetTypes={ } ) 100 ReferenceAndListParam theOwner, 101 102 103 @Description(shortDefinition="") 104 @OptionalParam(name="patient", targetTypes={ } ) 105 ReferenceAndListParam thePatient, 106 107 108 @Description(shortDefinition="") 109 @OptionalParam(name="period") 110 DateRangeParam thePeriod, 111 112 113 @Description(shortDefinition="") 114 @OptionalParam(name="status") 115 TokenAndListParam theStatus, 116 117 118 @Description(shortDefinition="") 119 @OptionalParam(name="subject", targetTypes={ } ) 120 ReferenceAndListParam theSubject, 121 122 123 @Description(shortDefinition="") 124 @OptionalParam(name="type") 125 TokenAndListParam theType, 126 127 @RawParam 128 Map<String, List<String>> theAdditionalRawParams, 129 130 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 131 @OptionalParam(name="_lastUpdated") 132 DateRangeParam the_lastUpdated, 133 134 @IncludeParam 135 Set<Include> theIncludes, 136 137 @IncludeParam(reverse=true) 138 Set<Include> theRevIncludes, 139 140 @Sort 141 SortSpec theSort, 142 143 @ca.uhn.fhir.rest.annotation.Count 144 Integer theCount, 145 146 @ca.uhn.fhir.rest.annotation.Offset 147 Integer theOffset, 148 149 SummaryEnum theSummaryMode, 150 151 SearchTotalModeEnum theSearchTotalMode, 152 153 SearchContainedModeEnum theSearchContainedMode 154 155 ) { 156 startRequest(theServletRequest); 157 try { 158 SearchParameterMap paramMap = new SearchParameterMap(); 159 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 160 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 161 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 162 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 163 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 164 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 165 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 166 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 167 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 168 169 paramMap.add("_has", theHas); 170 paramMap.add("_id", the_id); 171 paramMap.add("balance", theBalance); 172 paramMap.add("identifier", theIdentifier); 173 paramMap.add("name", theName); 174 paramMap.add("owner", theOwner); 175 paramMap.add("patient", thePatient); 176 paramMap.add("period", thePeriod); 177 paramMap.add("status", theStatus); 178 paramMap.add("subject", theSubject); 179 paramMap.add("type", theType); 180paramMap.setRevIncludes(theRevIncludes); 181 paramMap.setLastUpdated(the_lastUpdated); 182 paramMap.setIncludes(theIncludes); 183 paramMap.setSort(theSort); 184 paramMap.setCount(theCount); 185 paramMap.setOffset(theOffset); 186 paramMap.setSummaryMode(theSummaryMode); 187 paramMap.setSearchTotalMode(theSearchTotalMode); 188 paramMap.setSearchContainedMode(theSearchContainedMode); 189 190 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 191 192 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 193 return retVal; 194 } finally { 195 endRequest(theServletRequest); 196 } 197 } 198 199}