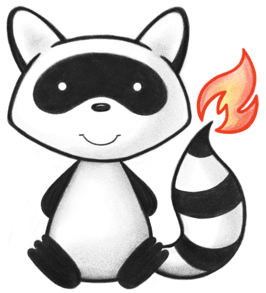
001 002package ca.uhn.fhir.jpa.rp.dstu2; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import ca.uhn.fhir.model.dstu2.composite.*; 012import ca.uhn.fhir.model.dstu2.resource.*; // 013import ca.uhn.fhir.rest.annotation.*; 014import ca.uhn.fhir.rest.param.*; 015import ca.uhn.fhir.rest.api.SortSpec; 016import ca.uhn.fhir.rest.api.SummaryEnum; 017import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 018import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 019 020public class DocumentReferenceResourceProvider extends 021 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<DocumentReference> 022 { 023 024 @Override 025 public Class<DocumentReference> getResourceType() { 026 return DocumentReference.class; 027 } 028 029 @Search(allowUnknownParams=true) 030 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 031 jakarta.servlet.http.HttpServletRequest theServletRequest, 032 jakarta.servlet.http.HttpServletResponse theServletResponse, 033 034 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 035 036 @Description(shortDefinition="Search the contents of the resource's data using a filter") 037 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 038 StringAndListParam theFtFilter, 039 040 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 041 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 042 StringAndListParam theFtContent, 043 044 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 045 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 046 StringAndListParam theFtText, 047 048 @Description(shortDefinition="Search for resources which have the given tag") 049 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 050 TokenAndListParam theSearchForTag, 051 052 @Description(shortDefinition="Search for resources which have the given security labels") 053 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 054 TokenAndListParam theSearchForSecurity, 055 056 @Description(shortDefinition="Search for resources which have the given profile") 057 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 058 UriAndListParam theSearchForProfile, 059 060 @Description(shortDefinition="Search the contents of the resource's data using a list") 061 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 062 StringAndListParam theList, 063 064 @Description(shortDefinition="The language of the resource") 065 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 066 TokenAndListParam theResourceLanguage, 067 068 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 069 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 070 UriAndListParam theSearchForSource, 071 072 @Description(shortDefinition="Return resources linked to by the given target") 073 @OptionalParam(name="_has") 074 HasAndListParam theHas, 075 076 077 078 @Description(shortDefinition="The ID of the resource") 079 @OptionalParam(name="_id") 080 StringAndListParam the_id, 081 082 083 @Description(shortDefinition="") 084 @OptionalParam(name="authenticator", targetTypes={ } ) 085 ReferenceAndListParam theAuthenticator, 086 087 088 @Description(shortDefinition="") 089 @OptionalParam(name="author", targetTypes={ } ) 090 ReferenceAndListParam theAuthor, 091 092 093 @Description(shortDefinition="") 094 @OptionalParam(name="class") 095 TokenAndListParam theClass, 096 097 098 @Description(shortDefinition="") 099 @OptionalParam(name="created") 100 DateRangeParam theCreated, 101 102 103 @Description(shortDefinition="") 104 @OptionalParam(name="custodian", targetTypes={ } ) 105 ReferenceAndListParam theCustodian, 106 107 108 @Description(shortDefinition="") 109 @OptionalParam(name="description") 110 StringAndListParam theDescription, 111 112 113 @Description(shortDefinition="") 114 @OptionalParam(name="encounter", targetTypes={ } ) 115 ReferenceAndListParam theEncounter, 116 117 118 @Description(shortDefinition="") 119 @OptionalParam(name="event") 120 TokenAndListParam theEvent, 121 122 123 @Description(shortDefinition="") 124 @OptionalParam(name="facility") 125 TokenAndListParam theFacility, 126 127 128 @Description(shortDefinition="") 129 @OptionalParam(name="format") 130 TokenAndListParam theFormat, 131 132 133 @Description(shortDefinition="") 134 @OptionalParam(name="identifier") 135 TokenAndListParam theIdentifier, 136 137 138 @Description(shortDefinition="") 139 @OptionalParam(name="indexed") 140 DateRangeParam theIndexed, 141 142 143 @Description(shortDefinition="") 144 @OptionalParam(name="language") 145 TokenAndListParam theLanguage, 146 147 148 @Description(shortDefinition="") 149 @OptionalParam(name="location") 150 UriAndListParam theLocation, 151 152 153 @Description(shortDefinition="") 154 @OptionalParam(name="patient", targetTypes={ } ) 155 ReferenceAndListParam thePatient, 156 157 158 @Description(shortDefinition="") 159 @OptionalParam(name="period") 160 DateRangeParam thePeriod, 161 162 163 @Description(shortDefinition="") 164 @OptionalParam(name="related-id") 165 TokenAndListParam theRelated_id, 166 167 168 @Description(shortDefinition="") 169 @OptionalParam(name="related-ref", targetTypes={ } ) 170 ReferenceAndListParam theRelated_ref, 171 172 173 @Description(shortDefinition="") 174 @OptionalParam(name="relatesto", targetTypes={ } ) 175 ReferenceAndListParam theRelatesto, 176 177 178 @Description(shortDefinition="Combination of relation and relatesTo") 179 @OptionalParam(name="relatesto-relation", compositeTypes= { ReferenceParam.class, TokenParam.class }) 180 CompositeAndListParam<ReferenceParam, TokenParam> theRelatesto_relation, 181 182 183 @Description(shortDefinition="") 184 @OptionalParam(name="relation") 185 TokenAndListParam theRelation, 186 187 188 @Description(shortDefinition="") 189 @OptionalParam(name="securitylabel") 190 TokenAndListParam theSecuritylabel, 191 192 193 @Description(shortDefinition="") 194 @OptionalParam(name="setting") 195 TokenAndListParam theSetting, 196 197 198 @Description(shortDefinition="") 199 @OptionalParam(name="status") 200 TokenAndListParam theStatus, 201 202 203 @Description(shortDefinition="") 204 @OptionalParam(name="subject", targetTypes={ } ) 205 ReferenceAndListParam theSubject, 206 207 208 @Description(shortDefinition="") 209 @OptionalParam(name="type") 210 TokenAndListParam theType, 211 212 @RawParam 213 Map<String, List<String>> theAdditionalRawParams, 214 215 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 216 @OptionalParam(name="_lastUpdated") 217 DateRangeParam the_lastUpdated, 218 219 @IncludeParam 220 Set<Include> theIncludes, 221 222 @IncludeParam(reverse=true) 223 Set<Include> theRevIncludes, 224 225 @Sort 226 SortSpec theSort, 227 228 @ca.uhn.fhir.rest.annotation.Count 229 Integer theCount, 230 231 @ca.uhn.fhir.rest.annotation.Offset 232 Integer theOffset, 233 234 SummaryEnum theSummaryMode, 235 236 SearchTotalModeEnum theSearchTotalMode, 237 238 SearchContainedModeEnum theSearchContainedMode 239 240 ) { 241 startRequest(theServletRequest); 242 try { 243 SearchParameterMap paramMap = new SearchParameterMap(); 244 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 245 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 246 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 247 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 248 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 249 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 250 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 251 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 252 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 253 254 paramMap.add("_has", theHas); 255 paramMap.add("_id", the_id); 256 paramMap.add("authenticator", theAuthenticator); 257 paramMap.add("author", theAuthor); 258 paramMap.add("class", theClass); 259 paramMap.add("created", theCreated); 260 paramMap.add("custodian", theCustodian); 261 paramMap.add("description", theDescription); 262 paramMap.add("encounter", theEncounter); 263 paramMap.add("event", theEvent); 264 paramMap.add("facility", theFacility); 265 paramMap.add("format", theFormat); 266 paramMap.add("identifier", theIdentifier); 267 paramMap.add("indexed", theIndexed); 268 paramMap.add("language", theLanguage); 269 paramMap.add("location", theLocation); 270 paramMap.add("patient", thePatient); 271 paramMap.add("period", thePeriod); 272 paramMap.add("related-id", theRelated_id); 273 paramMap.add("related-ref", theRelated_ref); 274 paramMap.add("relatesto", theRelatesto); 275 paramMap.add("relatesto-relation", theRelatesto_relation); 276 paramMap.add("relation", theRelation); 277 paramMap.add("securitylabel", theSecuritylabel); 278 paramMap.add("setting", theSetting); 279 paramMap.add("status", theStatus); 280 paramMap.add("subject", theSubject); 281 paramMap.add("type", theType); 282paramMap.setRevIncludes(theRevIncludes); 283 paramMap.setLastUpdated(the_lastUpdated); 284 paramMap.setIncludes(theIncludes); 285 paramMap.setSort(theSort); 286 paramMap.setCount(theCount); 287 paramMap.setOffset(theOffset); 288 paramMap.setSummaryMode(theSummaryMode); 289 paramMap.setSearchTotalMode(theSearchTotalMode); 290 paramMap.setSearchContainedMode(theSearchContainedMode); 291 292 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 293 294 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 295 return retVal; 296 } finally { 297 endRequest(theServletRequest); 298 } 299 } 300 301}