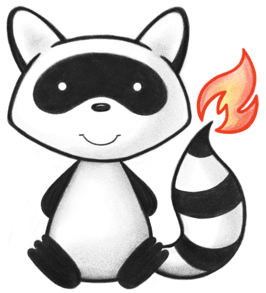
001 002package ca.uhn.fhir.jpa.rp.dstu2; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import ca.uhn.fhir.model.dstu2.composite.*; 012import ca.uhn.fhir.model.dstu2.resource.*; // 013import ca.uhn.fhir.rest.annotation.*; 014import ca.uhn.fhir.rest.param.*; 015import ca.uhn.fhir.rest.api.SortSpec; 016import ca.uhn.fhir.rest.api.SummaryEnum; 017import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 018import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 019 020public class PractitionerResourceProvider extends 021 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<Practitioner> 022 { 023 024 @Override 025 public Class<Practitioner> getResourceType() { 026 return Practitioner.class; 027 } 028 029 @Search(allowUnknownParams=true) 030 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 031 jakarta.servlet.http.HttpServletRequest theServletRequest, 032 jakarta.servlet.http.HttpServletResponse theServletResponse, 033 034 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 035 036 @Description(shortDefinition="Search the contents of the resource's data using a filter") 037 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 038 StringAndListParam theFtFilter, 039 040 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 041 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 042 StringAndListParam theFtContent, 043 044 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 045 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 046 StringAndListParam theFtText, 047 048 @Description(shortDefinition="Search for resources which have the given tag") 049 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 050 TokenAndListParam theSearchForTag, 051 052 @Description(shortDefinition="Search for resources which have the given security labels") 053 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 054 TokenAndListParam theSearchForSecurity, 055 056 @Description(shortDefinition="Search for resources which have the given profile") 057 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 058 UriAndListParam theSearchForProfile, 059 060 @Description(shortDefinition="Search the contents of the resource's data using a list") 061 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 062 StringAndListParam theList, 063 064 @Description(shortDefinition="The language of the resource") 065 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 066 TokenAndListParam theResourceLanguage, 067 068 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 069 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 070 UriAndListParam theSearchForSource, 071 072 @Description(shortDefinition="Return resources linked to by the given target") 073 @OptionalParam(name="_has") 074 HasAndListParam theHas, 075 076 077 078 @Description(shortDefinition="The ID of the resource") 079 @OptionalParam(name="_id") 080 StringAndListParam the_id, 081 082 083 @Description(shortDefinition="An address in any kind of address/part") 084 @OptionalParam(name="address") 085 StringAndListParam theAddress, 086 087 088 @Description(shortDefinition="A city specified in an address") 089 @OptionalParam(name="address-city") 090 StringAndListParam theAddress_city, 091 092 093 @Description(shortDefinition="A country specified in an address") 094 @OptionalParam(name="address-country") 095 StringAndListParam theAddress_country, 096 097 098 @Description(shortDefinition="A postalCode specified in an address") 099 @OptionalParam(name="address-postalcode") 100 StringAndListParam theAddress_postalcode, 101 102 103 @Description(shortDefinition="A state specified in an address") 104 @OptionalParam(name="address-state") 105 StringAndListParam theAddress_state, 106 107 108 @Description(shortDefinition="A use code specified in an address") 109 @OptionalParam(name="address-use") 110 TokenAndListParam theAddress_use, 111 112 113 @Description(shortDefinition="One of the languages that the practitioner can communicate with") 114 @OptionalParam(name="communication") 115 TokenAndListParam theCommunication, 116 117 118 @Description(shortDefinition="A value in an email contact") 119 @OptionalParam(name="email") 120 TokenAndListParam theEmail, 121 122 123 @Description(shortDefinition="A portion of the family name") 124 @OptionalParam(name="family") 125 StringAndListParam theFamily, 126 127 128 @Description(shortDefinition="Gender of the practitioner") 129 @OptionalParam(name="gender") 130 TokenAndListParam theGender, 131 132 133 @Description(shortDefinition="A portion of the given name") 134 @OptionalParam(name="given") 135 StringAndListParam theGiven, 136 137 138 @Description(shortDefinition="A practitioner's Identifier") 139 @OptionalParam(name="identifier") 140 TokenAndListParam theIdentifier, 141 142 143 @Description(shortDefinition="One of the locations at which this practitioner provides care") 144 @OptionalParam(name="location", targetTypes={ } ) 145 ReferenceAndListParam theLocation, 146 147 148 @Description(shortDefinition="A portion of either family or given name") 149 @OptionalParam(name="name") 150 StringAndListParam theName, 151 152 153 @Description(shortDefinition="The identity of the organization the practitioner represents / acts on behalf of") 154 @OptionalParam(name="organization", targetTypes={ } ) 155 ReferenceAndListParam theOrganization, 156 157 158 @Description(shortDefinition="A value in a phone contact") 159 @OptionalParam(name="phone") 160 TokenAndListParam thePhone, 161 162 163 @Description(shortDefinition="A portion of either family or given name using some kind of phonetic matching algorithm") 164 @OptionalParam(name="phonetic") 165 StringAndListParam thePhonetic, 166 167 168 @Description(shortDefinition="The practitioner can perform this role at for the organization") 169 @OptionalParam(name="role") 170 TokenAndListParam theRole, 171 172 173 @Description(shortDefinition="The practitioner has this specialty at an organization") 174 @OptionalParam(name="specialty") 175 TokenAndListParam theSpecialty, 176 177 178 @Description(shortDefinition="The value in any kind of contact") 179 @OptionalParam(name="telecom") 180 TokenAndListParam theTelecom, 181 182 @RawParam 183 Map<String, List<String>> theAdditionalRawParams, 184 185 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 186 @OptionalParam(name="_lastUpdated") 187 DateRangeParam the_lastUpdated, 188 189 @IncludeParam 190 Set<Include> theIncludes, 191 192 @IncludeParam(reverse=true) 193 Set<Include> theRevIncludes, 194 195 @Sort 196 SortSpec theSort, 197 198 @ca.uhn.fhir.rest.annotation.Count 199 Integer theCount, 200 201 @ca.uhn.fhir.rest.annotation.Offset 202 Integer theOffset, 203 204 SummaryEnum theSummaryMode, 205 206 SearchTotalModeEnum theSearchTotalMode, 207 208 SearchContainedModeEnum theSearchContainedMode 209 210 ) { 211 startRequest(theServletRequest); 212 try { 213 SearchParameterMap paramMap = new SearchParameterMap(); 214 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 215 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 216 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 217 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 218 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 219 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 220 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 221 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 222 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 223 224 paramMap.add("_has", theHas); 225 paramMap.add("_id", the_id); 226 paramMap.add("address", theAddress); 227 paramMap.add("address-city", theAddress_city); 228 paramMap.add("address-country", theAddress_country); 229 paramMap.add("address-postalcode", theAddress_postalcode); 230 paramMap.add("address-state", theAddress_state); 231 paramMap.add("address-use", theAddress_use); 232 paramMap.add("communication", theCommunication); 233 paramMap.add("email", theEmail); 234 paramMap.add("family", theFamily); 235 paramMap.add("gender", theGender); 236 paramMap.add("given", theGiven); 237 paramMap.add("identifier", theIdentifier); 238 paramMap.add("location", theLocation); 239 paramMap.add("name", theName); 240 paramMap.add("organization", theOrganization); 241 paramMap.add("phone", thePhone); 242 paramMap.add("phonetic", thePhonetic); 243 paramMap.add("role", theRole); 244 paramMap.add("specialty", theSpecialty); 245 paramMap.add("telecom", theTelecom); 246paramMap.setRevIncludes(theRevIncludes); 247 paramMap.setLastUpdated(the_lastUpdated); 248 paramMap.setIncludes(theIncludes); 249 paramMap.setSort(theSort); 250 paramMap.setCount(theCount); 251 paramMap.setOffset(theOffset); 252 paramMap.setSummaryMode(theSummaryMode); 253 paramMap.setSearchTotalMode(theSearchTotalMode); 254 paramMap.setSearchContainedMode(theSearchContainedMode); 255 256 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 257 258 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 259 return retVal; 260 } finally { 261 endRequest(theServletRequest); 262 } 263 } 264 265}