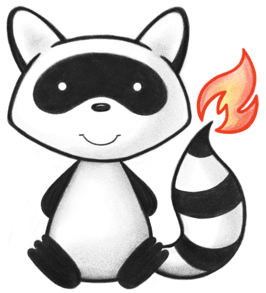
001 002package ca.uhn.fhir.jpa.rp.dstu2; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import ca.uhn.fhir.model.dstu2.composite.*; 012import ca.uhn.fhir.model.dstu2.resource.*; // 013import ca.uhn.fhir.rest.annotation.*; 014import ca.uhn.fhir.rest.param.*; 015import ca.uhn.fhir.rest.api.SortSpec; 016import ca.uhn.fhir.rest.api.SummaryEnum; 017import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 018import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 019 020public class SlotResourceProvider extends 021 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<Slot> 022 { 023 024 @Override 025 public Class<Slot> getResourceType() { 026 return Slot.class; 027 } 028 029 @Search(allowUnknownParams=true) 030 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 031 jakarta.servlet.http.HttpServletRequest theServletRequest, 032 jakarta.servlet.http.HttpServletResponse theServletResponse, 033 034 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 035 036 @Description(shortDefinition="Search the contents of the resource's data using a filter") 037 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 038 StringAndListParam theFtFilter, 039 040 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 041 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 042 StringAndListParam theFtContent, 043 044 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 045 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 046 StringAndListParam theFtText, 047 048 @Description(shortDefinition="Search for resources which have the given tag") 049 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 050 TokenAndListParam theSearchForTag, 051 052 @Description(shortDefinition="Search for resources which have the given security labels") 053 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 054 TokenAndListParam theSearchForSecurity, 055 056 @Description(shortDefinition="Search for resources which have the given profile") 057 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 058 UriAndListParam theSearchForProfile, 059 060 @Description(shortDefinition="Search the contents of the resource's data using a list") 061 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 062 StringAndListParam theList, 063 064 @Description(shortDefinition="The language of the resource") 065 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 066 TokenAndListParam theResourceLanguage, 067 068 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 069 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 070 UriAndListParam theSearchForSource, 071 072 @Description(shortDefinition="Return resources linked to by the given target") 073 @OptionalParam(name="_has") 074 HasAndListParam theHas, 075 076 077 078 @Description(shortDefinition="The ID of the resource") 079 @OptionalParam(name="_id") 080 StringAndListParam the_id, 081 082 083 @Description(shortDefinition="The free/busy status of the appointment") 084 @OptionalParam(name="fb-type") 085 TokenAndListParam theFb_type, 086 087 088 @Description(shortDefinition="A Slot Identifier") 089 @OptionalParam(name="identifier") 090 TokenAndListParam theIdentifier, 091 092 093 @Description(shortDefinition="The Schedule Resource that we are seeking a slot within") 094 @OptionalParam(name="schedule", targetTypes={ } ) 095 ReferenceAndListParam theSchedule, 096 097 098 @Description(shortDefinition="The type of appointments that can be booked into the slot") 099 @OptionalParam(name="slot-type") 100 TokenAndListParam theSlot_type, 101 102 103 @Description(shortDefinition="Appointment date/time.") 104 @OptionalParam(name="start") 105 DateRangeParam theStart, 106 107 @RawParam 108 Map<String, List<String>> theAdditionalRawParams, 109 110 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 111 @OptionalParam(name="_lastUpdated") 112 DateRangeParam the_lastUpdated, 113 114 @IncludeParam 115 Set<Include> theIncludes, 116 117 @IncludeParam(reverse=true) 118 Set<Include> theRevIncludes, 119 120 @Sort 121 SortSpec theSort, 122 123 @ca.uhn.fhir.rest.annotation.Count 124 Integer theCount, 125 126 @ca.uhn.fhir.rest.annotation.Offset 127 Integer theOffset, 128 129 SummaryEnum theSummaryMode, 130 131 SearchTotalModeEnum theSearchTotalMode, 132 133 SearchContainedModeEnum theSearchContainedMode 134 135 ) { 136 startRequest(theServletRequest); 137 try { 138 SearchParameterMap paramMap = new SearchParameterMap(); 139 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 140 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 141 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 142 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 143 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 144 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 145 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 146 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 147 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 148 149 paramMap.add("_has", theHas); 150 paramMap.add("_id", the_id); 151 paramMap.add("fb-type", theFb_type); 152 paramMap.add("identifier", theIdentifier); 153 paramMap.add("schedule", theSchedule); 154 paramMap.add("slot-type", theSlot_type); 155 paramMap.add("start", theStart); 156paramMap.setRevIncludes(theRevIncludes); 157 paramMap.setLastUpdated(the_lastUpdated); 158 paramMap.setIncludes(theIncludes); 159 paramMap.setSort(theSort); 160 paramMap.setCount(theCount); 161 paramMap.setOffset(theOffset); 162 paramMap.setSummaryMode(theSummaryMode); 163 paramMap.setSearchTotalMode(theSearchTotalMode); 164 paramMap.setSearchContainedMode(theSearchContainedMode); 165 166 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 167 168 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 169 return retVal; 170 } finally { 171 endRequest(theServletRequest); 172 } 173 } 174 175}