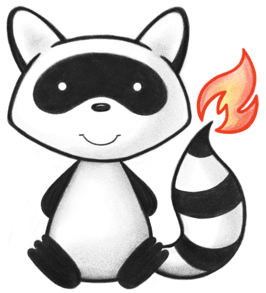
001 002package ca.uhn.fhir.jpa.rp.dstu3; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.dstu3.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class AuditEventResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<AuditEvent> 021 { 022 023 @Override 024 public Class<AuditEvent> getResourceType() { 025 return AuditEvent.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 083 @OptionalParam(name="_lastUpdated") 084 DateRangeParam the_lastUpdated, 085 086 087 @Description(shortDefinition="The profile of the resource") 088 @OptionalParam(name="_profile") 089 UriAndListParam the_profile, 090 091 092 @Description(shortDefinition="The security of the resource") 093 @OptionalParam(name="_security") 094 TokenAndListParam the_security, 095 096 097 @Description(shortDefinition="The tag of the resource") 098 @OptionalParam(name="_tag") 099 TokenAndListParam the_tag, 100 101 102 @Description(shortDefinition="Type of action performed during the event") 103 @OptionalParam(name="action") 104 TokenAndListParam theAction, 105 106 107 @Description(shortDefinition="Identifier for the network access point of the user device") 108 @OptionalParam(name="address") 109 StringAndListParam theAddress, 110 111 112 @Description(shortDefinition="Direct reference to resource") 113 @OptionalParam(name="agent", targetTypes={ } ) 114 ReferenceAndListParam theAgent, 115 116 117 @Description(shortDefinition="Human-meaningful name for the agent") 118 @OptionalParam(name="agent-name") 119 StringAndListParam theAgent_name, 120 121 122 @Description(shortDefinition="Agent role in the event") 123 @OptionalParam(name="agent-role") 124 TokenAndListParam theAgent_role, 125 126 127 @Description(shortDefinition="Alternative User id e.g. authentication") 128 @OptionalParam(name="altid") 129 TokenAndListParam theAltid, 130 131 132 @Description(shortDefinition="Time when the event occurred on source") 133 @OptionalParam(name="date") 134 DateRangeParam theDate, 135 136 137 @Description(shortDefinition="Specific instance of resource") 138 @OptionalParam(name="entity", targetTypes={ } ) 139 ReferenceAndListParam theEntity, 140 141 142 @Description(shortDefinition="Specific instance of object") 143 @OptionalParam(name="entity-id") 144 TokenAndListParam theEntity_id, 145 146 147 @Description(shortDefinition="Descriptor for entity") 148 @OptionalParam(name="entity-name") 149 StringAndListParam theEntity_name, 150 151 152 @Description(shortDefinition="What role the entity played") 153 @OptionalParam(name="entity-role") 154 TokenAndListParam theEntity_role, 155 156 157 @Description(shortDefinition="Type of entity involved") 158 @OptionalParam(name="entity-type") 159 TokenAndListParam theEntity_type, 160 161 162 @Description(shortDefinition="Whether the event succeeded or failed") 163 @OptionalParam(name="outcome") 164 TokenAndListParam theOutcome, 165 166 167 @Description(shortDefinition="Direct reference to resource") 168 @OptionalParam(name="patient", targetTypes={ } ) 169 ReferenceAndListParam thePatient, 170 171 172 @Description(shortDefinition="Policy that authorized event") 173 @OptionalParam(name="policy") 174 UriAndListParam thePolicy, 175 176 177 @Description(shortDefinition="Logical source location within the enterprise") 178 @OptionalParam(name="site") 179 TokenAndListParam theSite, 180 181 182 @Description(shortDefinition="The identity of source detecting the event") 183 @OptionalParam(name="source") 184 TokenAndListParam theSource, 185 186 187 @Description(shortDefinition="More specific type/id for the event") 188 @OptionalParam(name="subtype") 189 TokenAndListParam theSubtype, 190 191 192 @Description(shortDefinition="Type/identifier of event") 193 @OptionalParam(name="type") 194 TokenAndListParam theType, 195 196 197 @Description(shortDefinition="Unique identifier for the user") 198 @OptionalParam(name="user") 199 TokenAndListParam theUser, 200 201 @RawParam 202 Map<String, List<String>> theAdditionalRawParams, 203 204 205 @IncludeParam 206 Set<Include> theIncludes, 207 208 @IncludeParam(reverse=true) 209 Set<Include> theRevIncludes, 210 211 @Sort 212 SortSpec theSort, 213 214 @ca.uhn.fhir.rest.annotation.Count 215 Integer theCount, 216 217 @ca.uhn.fhir.rest.annotation.Offset 218 Integer theOffset, 219 220 SummaryEnum theSummaryMode, 221 222 SearchTotalModeEnum theSearchTotalMode, 223 224 SearchContainedModeEnum theSearchContainedMode 225 226 ) { 227 startRequest(theServletRequest); 228 try { 229 SearchParameterMap paramMap = new SearchParameterMap(); 230 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 231 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 232 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 233 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 234 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 235 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 236 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 237 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 238 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 239 240 paramMap.add("_has", theHas); 241 paramMap.add("_id", the_id); 242 paramMap.add("_profile", the_profile); 243 paramMap.add("_security", the_security); 244 paramMap.add("_tag", the_tag); 245 paramMap.add("action", theAction); 246 paramMap.add("address", theAddress); 247 paramMap.add("agent", theAgent); 248 paramMap.add("agent-name", theAgent_name); 249 paramMap.add("agent-role", theAgent_role); 250 paramMap.add("altid", theAltid); 251 paramMap.add("date", theDate); 252 paramMap.add("entity", theEntity); 253 paramMap.add("entity-id", theEntity_id); 254 paramMap.add("entity-name", theEntity_name); 255 paramMap.add("entity-role", theEntity_role); 256 paramMap.add("entity-type", theEntity_type); 257 paramMap.add("outcome", theOutcome); 258 paramMap.add("patient", thePatient); 259 paramMap.add("policy", thePolicy); 260 paramMap.add("site", theSite); 261 paramMap.add("source", theSource); 262 paramMap.add("subtype", theSubtype); 263 paramMap.add("type", theType); 264 paramMap.add("user", theUser); 265paramMap.setRevIncludes(theRevIncludes); 266 paramMap.setLastUpdated(the_lastUpdated); 267 paramMap.setIncludes(theIncludes); 268 paramMap.setSort(theSort); 269 paramMap.setCount(theCount); 270 paramMap.setOffset(theOffset); 271 paramMap.setSummaryMode(theSummaryMode); 272 paramMap.setSearchTotalMode(theSearchTotalMode); 273 paramMap.setSearchContainedMode(theSearchContainedMode); 274 275 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 276 277 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 278 return retVal; 279 } finally { 280 endRequest(theServletRequest); 281 } 282 } 283 284}