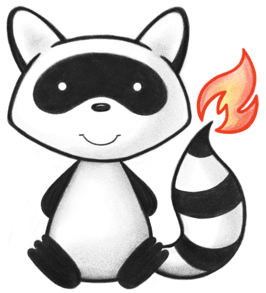
001 002package ca.uhn.fhir.jpa.rp.dstu3; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.dstu3.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class NamingSystemResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<NamingSystem> 021 { 022 023 @Override 024 public Class<NamingSystem> getResourceType() { 025 return NamingSystem.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Name of an individual to contact") 083 @OptionalParam(name="contact") 084 StringAndListParam theContact, 085 086 087 @Description(shortDefinition="The naming system publication date") 088 @OptionalParam(name="date") 089 DateRangeParam theDate, 090 091 092 @Description(shortDefinition="The description of the naming system") 093 @OptionalParam(name="description") 094 StringAndListParam theDescription, 095 096 097 @Description(shortDefinition="oid | uuid | uri | other") 098 @OptionalParam(name="id-type") 099 TokenAndListParam theId_type, 100 101 102 @Description(shortDefinition="Intended jurisdiction for the naming system") 103 @OptionalParam(name="jurisdiction") 104 TokenAndListParam theJurisdiction, 105 106 107 @Description(shortDefinition="codesystem | identifier | root") 108 @OptionalParam(name="kind") 109 TokenAndListParam theKind, 110 111 112 @Description(shortDefinition="Computationally friendly name of the naming system") 113 @OptionalParam(name="name") 114 StringAndListParam theName, 115 116 117 @Description(shortDefinition="When is identifier valid?") 118 @OptionalParam(name="period") 119 DateRangeParam thePeriod, 120 121 122 @Description(shortDefinition="Name of the publisher of the naming system") 123 @OptionalParam(name="publisher") 124 StringAndListParam thePublisher, 125 126 127 @Description(shortDefinition="Use this instead") 128 @OptionalParam(name="replaced-by", targetTypes={ } ) 129 ReferenceAndListParam theReplaced_by, 130 131 132 @Description(shortDefinition="Who maintains system namespace?") 133 @OptionalParam(name="responsible") 134 StringAndListParam theResponsible, 135 136 137 @Description(shortDefinition="The current status of the naming system") 138 @OptionalParam(name="status") 139 TokenAndListParam theStatus, 140 141 142 @Description(shortDefinition="Contact details for individual or organization") 143 @OptionalParam(name="telecom") 144 TokenAndListParam theTelecom, 145 146 147 @Description(shortDefinition="e.g. driver, provider, patient, bank etc.") 148 @OptionalParam(name="type") 149 TokenAndListParam theType, 150 151 152 @Description(shortDefinition="The unique identifier") 153 @OptionalParam(name="value") 154 StringAndListParam theValue, 155 156 @RawParam 157 Map<String, List<String>> theAdditionalRawParams, 158 159 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 160 @OptionalParam(name="_lastUpdated") 161 DateRangeParam theLastUpdated, 162 163 @IncludeParam 164 Set<Include> theIncludes, 165 166 @IncludeParam(reverse=true) 167 Set<Include> theRevIncludes, 168 169 @Sort 170 SortSpec theSort, 171 172 @ca.uhn.fhir.rest.annotation.Count 173 Integer theCount, 174 175 @ca.uhn.fhir.rest.annotation.Offset 176 Integer theOffset, 177 178 SummaryEnum theSummaryMode, 179 180 SearchTotalModeEnum theSearchTotalMode, 181 182 SearchContainedModeEnum theSearchContainedMode 183 184 ) { 185 startRequest(theServletRequest); 186 try { 187 SearchParameterMap paramMap = new SearchParameterMap(); 188 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 189 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 190 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 191 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 192 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 193 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 194 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 195 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 196 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 197 198 paramMap.add("_has", theHas); 199 paramMap.add("_id", the_id); 200 paramMap.add("contact", theContact); 201 paramMap.add("date", theDate); 202 paramMap.add("description", theDescription); 203 paramMap.add("id-type", theId_type); 204 paramMap.add("jurisdiction", theJurisdiction); 205 paramMap.add("kind", theKind); 206 paramMap.add("name", theName); 207 paramMap.add("period", thePeriod); 208 paramMap.add("publisher", thePublisher); 209 paramMap.add("replaced-by", theReplaced_by); 210 paramMap.add("responsible", theResponsible); 211 paramMap.add("status", theStatus); 212 paramMap.add("telecom", theTelecom); 213 paramMap.add("type", theType); 214 paramMap.add("value", theValue); 215 paramMap.setRevIncludes(theRevIncludes); 216 paramMap.setLastUpdated(theLastUpdated); 217 paramMap.setIncludes(theIncludes); 218 paramMap.setSort(theSort); 219 paramMap.setCount(theCount); 220 paramMap.setOffset(theOffset); 221 paramMap.setSummaryMode(theSummaryMode); 222 paramMap.setSearchTotalMode(theSearchTotalMode); 223 paramMap.setSearchContainedMode(theSearchContainedMode); 224 225 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 226 227 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 228 return retVal; 229 } finally { 230 endRequest(theServletRequest); 231 } 232 } 233 234}