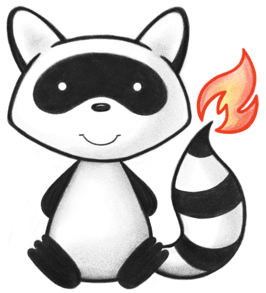
001 002package ca.uhn.fhir.jpa.rp.dstu3; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.dstu3.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class ObservationResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProviderObservation<Observation> 021 { 022 023 @Override 024 public Class<Observation> getResourceType() { 025 return Observation.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 048 @Description(shortDefinition="Search the contents of the resource's data using a list") 049 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 050 StringAndListParam theList, 051 052 @Description(shortDefinition="The language of the resource") 053 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 054 TokenAndListParam theResourceLanguage, 055 056 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 057 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 058 UriAndListParam theSearchForSource, 059 060 @Description(shortDefinition="Return resources linked to by the given target") 061 @OptionalParam(name="_has") 062 HasAndListParam theHas, 063 064 065 066 @Description(shortDefinition="The ID of the resource") 067 @OptionalParam(name="_id") 068 TokenAndListParam the_id, 069 070 071 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 072 @OptionalParam(name="_lastUpdated") 073 DateRangeParam the_lastUpdated, 074 075 076 @Description(shortDefinition="The profile of the resource") 077 @OptionalParam(name="_profile") 078 UriAndListParam the_profile, 079 080 081 @Description(shortDefinition="The security of the resource") 082 @OptionalParam(name="_security") 083 TokenAndListParam the_security, 084 085 086 @Description(shortDefinition="The tag of the resource") 087 @OptionalParam(name="_tag") 088 TokenAndListParam the_tag, 089 090 091 @Description(shortDefinition="Reference to the test or procedure request.") 092 @OptionalParam(name="based-on", targetTypes={ } ) 093 ReferenceAndListParam theBased_on, 094 095 096 @Description(shortDefinition="The classification of the type of observation") 097 @OptionalParam(name="category") 098 TokenAndListParam theCategory, 099 100 101 @Description(shortDefinition="The code of the observation type") 102 @OptionalParam(name="code") 103 TokenAndListParam theCode, 104 105 106 @Description(shortDefinition="Code and coded value parameter pair") 107 @OptionalParam(name="code-value-concept", compositeTypes= { TokenParam.class, TokenParam.class }) 108 CompositeAndListParam<TokenParam, TokenParam> theCode_value_concept, 109 110 111 @Description(shortDefinition="Code and date/time value parameter pair") 112 @OptionalParam(name="code-value-date", compositeTypes= { TokenParam.class, DateParam.class }) 113 CompositeAndListParam<TokenParam, DateParam> theCode_value_date, 114 115 116 @Description(shortDefinition="Code and quantity value parameter pair") 117 @OptionalParam(name="code-value-quantity", compositeTypes= { TokenParam.class, QuantityParam.class }) 118 CompositeAndListParam<TokenParam, QuantityParam> theCode_value_quantity, 119 120 121 @Description(shortDefinition="Code and string value parameter pair") 122 @OptionalParam(name="code-value-string", compositeTypes= { TokenParam.class, StringParam.class }) 123 CompositeAndListParam<TokenParam, StringParam> theCode_value_string, 124 125 126 @Description(shortDefinition="The code of the observation type or component type") 127 @OptionalParam(name="combo-code") 128 TokenAndListParam theCombo_code, 129 130 131 @Description(shortDefinition="Code and coded value parameter pair, including in components") 132 @OptionalParam(name="combo-code-value-concept", compositeTypes= { TokenParam.class, TokenParam.class }) 133 CompositeAndListParam<TokenParam, TokenParam> theCombo_code_value_concept, 134 135 136 @Description(shortDefinition="Code and quantity value parameter pair, including in components") 137 @OptionalParam(name="combo-code-value-quantity", compositeTypes= { TokenParam.class, QuantityParam.class }) 138 CompositeAndListParam<TokenParam, QuantityParam> theCombo_code_value_quantity, 139 140 141 @Description(shortDefinition="The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.") 142 @OptionalParam(name="combo-data-absent-reason") 143 TokenAndListParam theCombo_data_absent_reason, 144 145 146 @Description(shortDefinition="The value or component value of the observation, if the value is a CodeableConcept") 147 @OptionalParam(name="combo-value-concept") 148 TokenAndListParam theCombo_value_concept, 149 150 151 @Description(shortDefinition="The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)") 152 @OptionalParam(name="combo-value-quantity") 153 QuantityAndListParam theCombo_value_quantity, 154 155 156 @Description(shortDefinition="The component code of the observation type") 157 @OptionalParam(name="component-code") 158 TokenAndListParam theComponent_code, 159 160 161 @Description(shortDefinition="Component code and component coded value parameter pair") 162 @OptionalParam(name="component-code-value-concept", compositeTypes= { TokenParam.class, TokenParam.class }) 163 CompositeAndListParam<TokenParam, TokenParam> theComponent_code_value_concept, 164 165 166 @Description(shortDefinition="Component code and component quantity value parameter pair") 167 @OptionalParam(name="component-code-value-quantity", compositeTypes= { TokenParam.class, QuantityParam.class }) 168 CompositeAndListParam<TokenParam, QuantityParam> theComponent_code_value_quantity, 169 170 171 @Description(shortDefinition="The reason why the expected value in the element Observation.component.value[x] is missing.") 172 @OptionalParam(name="component-data-absent-reason") 173 TokenAndListParam theComponent_data_absent_reason, 174 175 176 @Description(shortDefinition="The value of the component observation, if the value is a CodeableConcept") 177 @OptionalParam(name="component-value-concept") 178 TokenAndListParam theComponent_value_concept, 179 180 181 @Description(shortDefinition="The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)") 182 @OptionalParam(name="component-value-quantity") 183 QuantityAndListParam theComponent_value_quantity, 184 185 186 @Description(shortDefinition="Healthcare event (Episode-of-care or Encounter) related to the observation") 187 @OptionalParam(name="context", targetTypes={ } ) 188 ReferenceAndListParam theContext, 189 190 191 @Description(shortDefinition="The reason why the expected value in the element Observation.value[x] is missing.") 192 @OptionalParam(name="data-absent-reason") 193 TokenAndListParam theData_absent_reason, 194 195 196 @Description(shortDefinition="Obtained date/time. If the obtained element is a period, a date that falls in the period") 197 @OptionalParam(name="date") 198 DateRangeParam theDate, 199 200 201 @Description(shortDefinition="The Device that generated the observation data.") 202 @OptionalParam(name="device", targetTypes={ } ) 203 ReferenceAndListParam theDevice, 204 205 206 @Description(shortDefinition="Encounter related to the observation") 207 @OptionalParam(name="encounter", targetTypes={ } ) 208 ReferenceAndListParam theEncounter, 209 210 211 @Description(shortDefinition="The unique id for a particular observation") 212 @OptionalParam(name="identifier") 213 TokenAndListParam theIdentifier, 214 215 216 @Description(shortDefinition="The method used for the observation") 217 @OptionalParam(name="method") 218 TokenAndListParam theMethod, 219 220 221 @Description(shortDefinition="The subject that the observation is about (if patient)") 222 @OptionalParam(name="patient", targetTypes={ } ) 223 ReferenceAndListParam thePatient, 224 225 226 @Description(shortDefinition="Who performed the observation") 227 @OptionalParam(name="performer", targetTypes={ } ) 228 ReferenceAndListParam thePerformer, 229 230 231 @Description(shortDefinition="Related Observations - search on related-type and related-target together") 232 @OptionalParam(name="related", compositeTypes= { ReferenceParam.class, TokenParam.class }) 233 CompositeAndListParam<ReferenceParam, TokenParam> theRelated, 234 235 236 @Description(shortDefinition="Resource that is related to this one") 237 @OptionalParam(name="related-target", targetTypes={ } ) 238 ReferenceAndListParam theRelated_target, 239 240 241 @Description(shortDefinition="has-member | derived-from | sequel-to | replaces | qualified-by | interfered-by") 242 @OptionalParam(name="related-type") 243 TokenAndListParam theRelated_type, 244 245 246 @Description(shortDefinition="Specimen used for this observation") 247 @OptionalParam(name="specimen", targetTypes={ } ) 248 ReferenceAndListParam theSpecimen, 249 250 251 @Description(shortDefinition="The status of the observation") 252 @OptionalParam(name="status") 253 TokenAndListParam theStatus, 254 255 256 @Description(shortDefinition="The subject that the observation is about") 257 @OptionalParam(name="subject", targetTypes={ } ) 258 ReferenceAndListParam theSubject, 259 260 261 @Description(shortDefinition="The value of the observation, if the value is a CodeableConcept") 262 @OptionalParam(name="value-concept") 263 TokenAndListParam theValue_concept, 264 265 266 @Description(shortDefinition="The value of the observation, if the value is a date or period of time") 267 @OptionalParam(name="value-date") 268 DateRangeParam theValue_date, 269 270 271 @Description(shortDefinition="The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)") 272 @OptionalParam(name="value-quantity") 273 QuantityAndListParam theValue_quantity, 274 275 276 @Description(shortDefinition="The value of the observation, if the value is a string, and also searches in CodeableConcept.text") 277 @OptionalParam(name="value-string") 278 StringAndListParam theValue_string, 279 280 @RawParam 281 Map<String, List<String>> theAdditionalRawParams, 282 283 284 @IncludeParam 285 Set<Include> theIncludes, 286 287 @IncludeParam(reverse=true) 288 Set<Include> theRevIncludes, 289 290 @Sort 291 SortSpec theSort, 292 293 @ca.uhn.fhir.rest.annotation.Count 294 Integer theCount, 295 296 @ca.uhn.fhir.rest.annotation.Offset 297 Integer theOffset, 298 299 SummaryEnum theSummaryMode, 300 301 SearchTotalModeEnum theSearchTotalMode, 302 303 SearchContainedModeEnum theSearchContainedMode 304 305 ) { 306 startRequest(theServletRequest); 307 try { 308 SearchParameterMap paramMap = new SearchParameterMap(); 309 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 310 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 311 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 312 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 313 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 314 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 315 316 paramMap.add("_has", theHas); 317 paramMap.add("_id", the_id); 318 paramMap.add("_profile", the_profile); 319 paramMap.add("_security", the_security); 320 paramMap.add("_tag", the_tag); 321 paramMap.add("based-on", theBased_on); 322 paramMap.add("category", theCategory); 323 paramMap.add("code", theCode); 324 paramMap.add("code-value-concept", theCode_value_concept); 325 paramMap.add("code-value-date", theCode_value_date); 326 paramMap.add("code-value-quantity", theCode_value_quantity); 327 paramMap.add("code-value-string", theCode_value_string); 328 paramMap.add("combo-code", theCombo_code); 329 paramMap.add("combo-code-value-concept", theCombo_code_value_concept); 330 paramMap.add("combo-code-value-quantity", theCombo_code_value_quantity); 331 paramMap.add("combo-data-absent-reason", theCombo_data_absent_reason); 332 paramMap.add("combo-value-concept", theCombo_value_concept); 333 paramMap.add("combo-value-quantity", theCombo_value_quantity); 334 paramMap.add("component-code", theComponent_code); 335 paramMap.add("component-code-value-concept", theComponent_code_value_concept); 336 paramMap.add("component-code-value-quantity", theComponent_code_value_quantity); 337 paramMap.add("component-data-absent-reason", theComponent_data_absent_reason); 338 paramMap.add("component-value-concept", theComponent_value_concept); 339 paramMap.add("component-value-quantity", theComponent_value_quantity); 340 paramMap.add("context", theContext); 341 paramMap.add("data-absent-reason", theData_absent_reason); 342 paramMap.add("date", theDate); 343 paramMap.add("device", theDevice); 344 paramMap.add("encounter", theEncounter); 345 paramMap.add("identifier", theIdentifier); 346 paramMap.add("method", theMethod); 347 paramMap.add("patient", thePatient); 348 paramMap.add("performer", thePerformer); 349 paramMap.add("related", theRelated); 350 paramMap.add("related-target", theRelated_target); 351 paramMap.add("related-type", theRelated_type); 352 paramMap.add("specimen", theSpecimen); 353 paramMap.add("status", theStatus); 354 paramMap.add("subject", theSubject); 355 paramMap.add("value-concept", theValue_concept); 356 paramMap.add("value-date", theValue_date); 357 paramMap.add("value-quantity", theValue_quantity); 358 paramMap.add("value-string", theValue_string); 359paramMap.setRevIncludes(theRevIncludes); 360 paramMap.setLastUpdated(the_lastUpdated); 361 paramMap.setIncludes(theIncludes); 362 paramMap.setSort(theSort); 363 paramMap.setCount(theCount); 364 paramMap.setOffset(theOffset); 365 paramMap.setSummaryMode(theSummaryMode); 366 paramMap.setSearchTotalMode(theSearchTotalMode); 367 paramMap.setSearchContainedMode(theSearchContainedMode); 368 369 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 370 371 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 372 return retVal; 373 } finally { 374 endRequest(theServletRequest); 375 } 376 } 377 378}