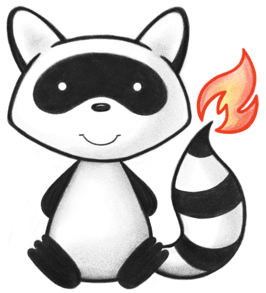
001 002package ca.uhn.fhir.jpa.rp.r4; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r4.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class BinaryResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<Binary> 021 { 022 023 @Override 024 public Class<Binary> getResourceType() { 025 return Binary.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 048 @Description(shortDefinition="Search the contents of the resource's data using a list") 049 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 050 StringAndListParam theList, 051 052 @Description(shortDefinition="The language of the resource") 053 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 054 TokenAndListParam theResourceLanguage, 055 056 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 057 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 058 UriAndListParam theSearchForSource, 059 060 @Description(shortDefinition="Return resources linked to by the given target") 061 @OptionalParam(name="_has") 062 HasAndListParam theHas, 063 064 065 066 @Description(shortDefinition="The ID of the resource") 067 @OptionalParam(name="_id") 068 TokenAndListParam the_id, 069 070 071 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 072 @OptionalParam(name="_lastUpdated") 073 DateRangeParam the_lastUpdated, 074 075 076 @Description(shortDefinition="The profile of the resource") 077 @OptionalParam(name="_profile") 078 UriAndListParam the_profile, 079 080 081 @Description(shortDefinition="The security of the resource") 082 @OptionalParam(name="_security") 083 TokenAndListParam the_security, 084 085 086 @Description(shortDefinition="The tag of the resource") 087 @OptionalParam(name="_tag") 088 TokenAndListParam the_tag, 089 090 @RawParam 091 Map<String, List<String>> theAdditionalRawParams, 092 093 094 @IncludeParam 095 Set<Include> theIncludes, 096 097 @IncludeParam(reverse=true) 098 Set<Include> theRevIncludes, 099 100 @Sort 101 SortSpec theSort, 102 103 @ca.uhn.fhir.rest.annotation.Count 104 Integer theCount, 105 106 @ca.uhn.fhir.rest.annotation.Offset 107 Integer theOffset, 108 109 SummaryEnum theSummaryMode, 110 111 SearchTotalModeEnum theSearchTotalMode, 112 113 SearchContainedModeEnum theSearchContainedMode 114 115 ) { 116 startRequest(theServletRequest); 117 try { 118 SearchParameterMap paramMap = new SearchParameterMap(); 119 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 120 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 121 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 122 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 123 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 124 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 125 126 paramMap.add("_has", theHas); 127 paramMap.add("_id", the_id); 128 paramMap.add("_profile", the_profile); 129 paramMap.add("_security", the_security); 130 paramMap.add("_tag", the_tag); 131paramMap.setRevIncludes(theRevIncludes); 132 paramMap.setLastUpdated(the_lastUpdated); 133 paramMap.setIncludes(theIncludes); 134 paramMap.setSort(theSort); 135 paramMap.setCount(theCount); 136 paramMap.setOffset(theOffset); 137 paramMap.setSummaryMode(theSummaryMode); 138 paramMap.setSearchTotalMode(theSearchTotalMode); 139 paramMap.setSearchContainedMode(theSearchContainedMode); 140 141 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 142 143 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 144 return retVal; 145 } finally { 146 endRequest(theServletRequest); 147 } 148 } 149 150}