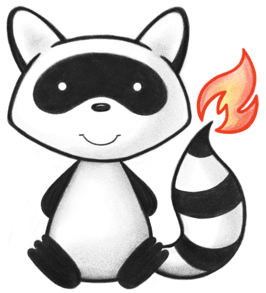
001 002package ca.uhn.fhir.jpa.rp.r4; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r4.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class EncounterResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProviderEncounter<Encounter> 021 { 022 023 @Override 024 public Class<Encounter> getResourceType() { 025 return Encounter.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 083 @OptionalParam(name="_lastUpdated") 084 DateRangeParam the_lastUpdated, 085 086 087 @Description(shortDefinition="The profile of the resource") 088 @OptionalParam(name="_profile") 089 UriAndListParam the_profile, 090 091 092 @Description(shortDefinition="The security of the resource") 093 @OptionalParam(name="_security") 094 TokenAndListParam the_security, 095 096 097 @Description(shortDefinition="The tag of the resource") 098 @OptionalParam(name="_tag") 099 TokenAndListParam the_tag, 100 101 102 @Description(shortDefinition="The set of accounts that may be used for billing for this Encounter") 103 @OptionalParam(name="account", targetTypes={ } ) 104 ReferenceAndListParam theAccount, 105 106 107 @Description(shortDefinition="The appointment that scheduled this encounter") 108 @OptionalParam(name="appointment", targetTypes={ } ) 109 ReferenceAndListParam theAppointment, 110 111 112 @Description(shortDefinition="The ServiceRequest that initiated this encounter") 113 @OptionalParam(name="based-on", targetTypes={ } ) 114 ReferenceAndListParam theBased_on, 115 116 117 @Description(shortDefinition="Classification of patient encounter") 118 @OptionalParam(name="class") 119 TokenAndListParam theClass, 120 121 122 @Description(shortDefinition="A date within the period the Encounter lasted") 123 @OptionalParam(name="date") 124 DateRangeParam theDate, 125 126 127 @Description(shortDefinition="The diagnosis or procedure relevant to the encounter") 128 @OptionalParam(name="diagnosis", targetTypes={ } ) 129 ReferenceAndListParam theDiagnosis, 130 131 132 @Description(shortDefinition="Episode(s) of care that this encounter should be recorded against") 133 @OptionalParam(name="episode-of-care", targetTypes={ } ) 134 ReferenceAndListParam theEpisode_of_care, 135 136 137 @Description(shortDefinition="Identifier(s) by which this encounter is known") 138 @OptionalParam(name="identifier") 139 TokenAndListParam theIdentifier, 140 141 142 @Description(shortDefinition="Length of encounter in days") 143 @OptionalParam(name="length") 144 QuantityAndListParam theLength, 145 146 147 @Description(shortDefinition="Location the encounter takes place") 148 @OptionalParam(name="location", targetTypes={ } ) 149 ReferenceAndListParam theLocation, 150 151 152 @Description(shortDefinition="Time period during which the patient was present at the location") 153 @OptionalParam(name="location-period") 154 DateRangeParam theLocation_period, 155 156 157 @Description(shortDefinition="Another Encounter this encounter is part of") 158 @OptionalParam(name="part-of", targetTypes={ } ) 159 ReferenceAndListParam thePart_of, 160 161 162 @Description(shortDefinition="Persons involved in the encounter other than the patient") 163 @OptionalParam(name="participant", targetTypes={ } ) 164 ReferenceAndListParam theParticipant, 165 166 167 @Description(shortDefinition="Role of participant in encounter") 168 @OptionalParam(name="participant-type") 169 TokenAndListParam theParticipant_type, 170 171 172 @Description(shortDefinition="The patient or group present at the encounter") 173 @OptionalParam(name="patient", targetTypes={ } ) 174 ReferenceAndListParam thePatient, 175 176 177 @Description(shortDefinition="Persons involved in the encounter other than the patient") 178 @OptionalParam(name="practitioner", targetTypes={ } ) 179 ReferenceAndListParam thePractitioner, 180 181 182 @Description(shortDefinition="Coded reason the encounter takes place") 183 @OptionalParam(name="reason-code") 184 TokenAndListParam theReason_code, 185 186 187 @Description(shortDefinition="Reason the encounter takes place (reference)") 188 @OptionalParam(name="reason-reference", targetTypes={ } ) 189 ReferenceAndListParam theReason_reference, 190 191 192 @Description(shortDefinition="The organization (facility) responsible for this encounter") 193 @OptionalParam(name="service-provider", targetTypes={ } ) 194 ReferenceAndListParam theService_provider, 195 196 197 @Description(shortDefinition="Wheelchair, translator, stretcher, etc.") 198 @OptionalParam(name="special-arrangement") 199 TokenAndListParam theSpecial_arrangement, 200 201 202 @Description(shortDefinition="planned | arrived | triaged | in-progress | onleave | finished | cancelled +") 203 @OptionalParam(name="status") 204 TokenAndListParam theStatus, 205 206 207 @Description(shortDefinition="The patient or group present at the encounter") 208 @OptionalParam(name="subject", targetTypes={ } ) 209 ReferenceAndListParam theSubject, 210 211 212 @Description(shortDefinition="Specific type of encounter") 213 @OptionalParam(name="type") 214 TokenAndListParam theType, 215 216 @RawParam 217 Map<String, List<String>> theAdditionalRawParams, 218 219 220 @IncludeParam 221 Set<Include> theIncludes, 222 223 @IncludeParam(reverse=true) 224 Set<Include> theRevIncludes, 225 226 @Sort 227 SortSpec theSort, 228 229 @ca.uhn.fhir.rest.annotation.Count 230 Integer theCount, 231 232 @ca.uhn.fhir.rest.annotation.Offset 233 Integer theOffset, 234 235 SummaryEnum theSummaryMode, 236 237 SearchTotalModeEnum theSearchTotalMode, 238 239 SearchContainedModeEnum theSearchContainedMode 240 241 ) { 242 startRequest(theServletRequest); 243 try { 244 SearchParameterMap paramMap = new SearchParameterMap(); 245 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 246 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 247 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 248 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 249 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 250 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 251 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 252 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 253 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 254 255 paramMap.add("_has", theHas); 256 paramMap.add("_id", the_id); 257 paramMap.add("_profile", the_profile); 258 paramMap.add("_security", the_security); 259 paramMap.add("_tag", the_tag); 260 paramMap.add("account", theAccount); 261 paramMap.add("appointment", theAppointment); 262 paramMap.add("based-on", theBased_on); 263 paramMap.add("class", theClass); 264 paramMap.add("date", theDate); 265 paramMap.add("diagnosis", theDiagnosis); 266 paramMap.add("episode-of-care", theEpisode_of_care); 267 paramMap.add("identifier", theIdentifier); 268 paramMap.add("length", theLength); 269 paramMap.add("location", theLocation); 270 paramMap.add("location-period", theLocation_period); 271 paramMap.add("part-of", thePart_of); 272 paramMap.add("participant", theParticipant); 273 paramMap.add("participant-type", theParticipant_type); 274 paramMap.add("patient", thePatient); 275 paramMap.add("practitioner", thePractitioner); 276 paramMap.add("reason-code", theReason_code); 277 paramMap.add("reason-reference", theReason_reference); 278 paramMap.add("service-provider", theService_provider); 279 paramMap.add("special-arrangement", theSpecial_arrangement); 280 paramMap.add("status", theStatus); 281 paramMap.add("subject", theSubject); 282 paramMap.add("type", theType); 283paramMap.setRevIncludes(theRevIncludes); 284 paramMap.setLastUpdated(the_lastUpdated); 285 paramMap.setIncludes(theIncludes); 286 paramMap.setSort(theSort); 287 paramMap.setCount(theCount); 288 paramMap.setOffset(theOffset); 289 paramMap.setSummaryMode(theSummaryMode); 290 paramMap.setSearchTotalMode(theSearchTotalMode); 291 paramMap.setSearchContainedMode(theSearchContainedMode); 292 293 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 294 295 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 296 return retVal; 297 } finally { 298 endRequest(theServletRequest); 299 } 300 } 301 302}