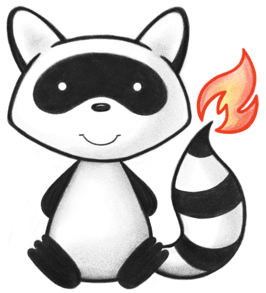
001 002package ca.uhn.fhir.jpa.rp.r4; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r4.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class LocationResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<Location> 021 { 022 023 @Override 024 public Class<Location> getResourceType() { 025 return Location.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 083 @OptionalParam(name="_lastUpdated") 084 DateRangeParam the_lastUpdated, 085 086 087 @Description(shortDefinition="The profile of the resource") 088 @OptionalParam(name="_profile") 089 UriAndListParam the_profile, 090 091 092 @Description(shortDefinition="The security of the resource") 093 @OptionalParam(name="_security") 094 TokenAndListParam the_security, 095 096 097 @Description(shortDefinition="The tag of the resource") 098 @OptionalParam(name="_tag") 099 TokenAndListParam the_tag, 100 101 102 @Description(shortDefinition="A (part of the) address of the location") 103 @OptionalParam(name="address") 104 StringAndListParam theAddress, 105 106 107 @Description(shortDefinition="A city specified in an address") 108 @OptionalParam(name="address-city") 109 StringAndListParam theAddress_city, 110 111 112 @Description(shortDefinition="A country specified in an address") 113 @OptionalParam(name="address-country") 114 StringAndListParam theAddress_country, 115 116 117 @Description(shortDefinition="A postal code specified in an address") 118 @OptionalParam(name="address-postalcode") 119 StringAndListParam theAddress_postalcode, 120 121 122 @Description(shortDefinition="A state specified in an address") 123 @OptionalParam(name="address-state") 124 StringAndListParam theAddress_state, 125 126 127 @Description(shortDefinition="A use code specified in an address") 128 @OptionalParam(name="address-use") 129 TokenAndListParam theAddress_use, 130 131 132 @Description(shortDefinition="Technical endpoints providing access to services operated for the location") 133 @OptionalParam(name="endpoint", targetTypes={ } ) 134 ReferenceAndListParam theEndpoint, 135 136 137 @Description(shortDefinition="An identifier for the location") 138 @OptionalParam(name="identifier") 139 TokenAndListParam theIdentifier, 140 141 142 @Description(shortDefinition="A portion of the location's name or alias") 143 @OptionalParam(name="name") 144 StringAndListParam theName, 145 146 147 @Description(shortDefinition="Search for locations where the location.position is near to, or within a specified distance of, the provided coordinates expressed as [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes).If the units are omitted, then kms should be assumed. If the distance is omitted, then the server can use its own discretion as to what distances should be considered near (and units are irrelevant)Servers may search using various techniques that might have differing accuracies, depending on implementation efficiency.Requires the near-distance parameter to be provided also") 148 @OptionalParam(name="near") 149 SpecialAndListParam theNear, 150 151 152 @Description(shortDefinition="Searches for locations (typically bed/room) that have an operational status (e.g. contaminated, housekeeping)") 153 @OptionalParam(name="operational-status") 154 TokenAndListParam theOperational_status, 155 156 157 @Description(shortDefinition="Searches for locations that are managed by the provided organization") 158 @OptionalParam(name="organization", targetTypes={ } ) 159 ReferenceAndListParam theOrganization, 160 161 162 @Description(shortDefinition="A location of which this location is a part") 163 @OptionalParam(name="partof", targetTypes={ } ) 164 ReferenceAndListParam thePartof, 165 166 167 @Description(shortDefinition="Searches for locations with a specific kind of status") 168 @OptionalParam(name="status") 169 TokenAndListParam theStatus, 170 171 172 @Description(shortDefinition="A code for the type of location") 173 @OptionalParam(name="type") 174 TokenAndListParam theType, 175 176 @RawParam 177 Map<String, List<String>> theAdditionalRawParams, 178 179 180 @IncludeParam 181 Set<Include> theIncludes, 182 183 @IncludeParam(reverse=true) 184 Set<Include> theRevIncludes, 185 186 @Sort 187 SortSpec theSort, 188 189 @ca.uhn.fhir.rest.annotation.Count 190 Integer theCount, 191 192 @ca.uhn.fhir.rest.annotation.Offset 193 Integer theOffset, 194 195 SummaryEnum theSummaryMode, 196 197 SearchTotalModeEnum theSearchTotalMode, 198 199 SearchContainedModeEnum theSearchContainedMode 200 201 ) { 202 startRequest(theServletRequest); 203 try { 204 SearchParameterMap paramMap = new SearchParameterMap(); 205 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 206 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 207 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 208 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 209 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 210 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 211 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 212 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 213 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 214 215 paramMap.add("_has", theHas); 216 paramMap.add("_id", the_id); 217 paramMap.add("_profile", the_profile); 218 paramMap.add("_security", the_security); 219 paramMap.add("_tag", the_tag); 220 paramMap.add("address", theAddress); 221 paramMap.add("address-city", theAddress_city); 222 paramMap.add("address-country", theAddress_country); 223 paramMap.add("address-postalcode", theAddress_postalcode); 224 paramMap.add("address-state", theAddress_state); 225 paramMap.add("address-use", theAddress_use); 226 paramMap.add("endpoint", theEndpoint); 227 paramMap.add("identifier", theIdentifier); 228 paramMap.add("name", theName); 229 paramMap.add("near", theNear); 230 paramMap.add("operational-status", theOperational_status); 231 paramMap.add("organization", theOrganization); 232 paramMap.add("partof", thePartof); 233 paramMap.add("status", theStatus); 234 paramMap.add("type", theType); 235paramMap.setRevIncludes(theRevIncludes); 236 paramMap.setLastUpdated(the_lastUpdated); 237 paramMap.setIncludes(theIncludes); 238 paramMap.setSort(theSort); 239 paramMap.setCount(theCount); 240 paramMap.setOffset(theOffset); 241 paramMap.setSummaryMode(theSummaryMode); 242 paramMap.setSearchTotalMode(theSearchTotalMode); 243 paramMap.setSearchContainedMode(theSearchContainedMode); 244 245 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 246 247 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 248 return retVal; 249 } finally { 250 endRequest(theServletRequest); 251 } 252 } 253 254}