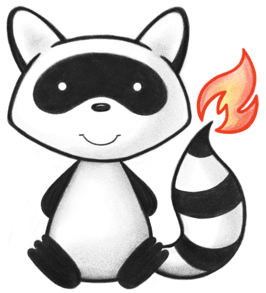
001 002package ca.uhn.fhir.jpa.rp.r4; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r4.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class OrganizationResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<Organization> 021 { 022 023 @Override 024 public Class<Organization> getResourceType() { 025 return Organization.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 083 @OptionalParam(name="_lastUpdated") 084 DateRangeParam the_lastUpdated, 085 086 087 @Description(shortDefinition="The profile of the resource") 088 @OptionalParam(name="_profile") 089 UriAndListParam the_profile, 090 091 092 @Description(shortDefinition="The security of the resource") 093 @OptionalParam(name="_security") 094 TokenAndListParam the_security, 095 096 097 @Description(shortDefinition="The tag of the resource") 098 @OptionalParam(name="_tag") 099 TokenAndListParam the_tag, 100 101 102 @Description(shortDefinition="Is the Organization record active") 103 @OptionalParam(name="active") 104 TokenAndListParam theActive, 105 106 107 @Description(shortDefinition="A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text") 108 @OptionalParam(name="address") 109 StringAndListParam theAddress, 110 111 112 @Description(shortDefinition="A city specified in an address") 113 @OptionalParam(name="address-city") 114 StringAndListParam theAddress_city, 115 116 117 @Description(shortDefinition="A country specified in an address") 118 @OptionalParam(name="address-country") 119 StringAndListParam theAddress_country, 120 121 122 @Description(shortDefinition="A postal code specified in an address") 123 @OptionalParam(name="address-postalcode") 124 StringAndListParam theAddress_postalcode, 125 126 127 @Description(shortDefinition="A state specified in an address") 128 @OptionalParam(name="address-state") 129 StringAndListParam theAddress_state, 130 131 132 @Description(shortDefinition="A use code specified in an address") 133 @OptionalParam(name="address-use") 134 TokenAndListParam theAddress_use, 135 136 137 @Description(shortDefinition="Technical endpoints providing access to services operated for the organization") 138 @OptionalParam(name="endpoint", targetTypes={ } ) 139 ReferenceAndListParam theEndpoint, 140 141 142 @Description(shortDefinition="Any identifier for the organization (not the accreditation issuer's identifier)") 143 @OptionalParam(name="identifier") 144 TokenAndListParam theIdentifier, 145 146 147 @Description(shortDefinition="A portion of the organization's name or alias") 148 @OptionalParam(name="name") 149 StringAndListParam theName, 150 151 152 @Description(shortDefinition="An organization of which this organization forms a part") 153 @OptionalParam(name="partof", targetTypes={ } ) 154 ReferenceAndListParam thePartof, 155 156 157 @Description(shortDefinition="A portion of the organization's name using some kind of phonetic matching algorithm") 158 @OptionalParam(name="phonetic") 159 StringAndListParam thePhonetic, 160 161 162 @Description(shortDefinition="A code for the type of organization") 163 @OptionalParam(name="type") 164 TokenAndListParam theType, 165 166 @RawParam 167 Map<String, List<String>> theAdditionalRawParams, 168 169 170 @IncludeParam 171 Set<Include> theIncludes, 172 173 @IncludeParam(reverse=true) 174 Set<Include> theRevIncludes, 175 176 @Sort 177 SortSpec theSort, 178 179 @ca.uhn.fhir.rest.annotation.Count 180 Integer theCount, 181 182 @ca.uhn.fhir.rest.annotation.Offset 183 Integer theOffset, 184 185 SummaryEnum theSummaryMode, 186 187 SearchTotalModeEnum theSearchTotalMode, 188 189 SearchContainedModeEnum theSearchContainedMode 190 191 ) { 192 startRequest(theServletRequest); 193 try { 194 SearchParameterMap paramMap = new SearchParameterMap(); 195 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 196 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 197 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 198 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 199 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 200 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 201 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 202 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 203 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 204 205 paramMap.add("_has", theHas); 206 paramMap.add("_id", the_id); 207 paramMap.add("_profile", the_profile); 208 paramMap.add("_security", the_security); 209 paramMap.add("_tag", the_tag); 210 paramMap.add("active", theActive); 211 paramMap.add("address", theAddress); 212 paramMap.add("address-city", theAddress_city); 213 paramMap.add("address-country", theAddress_country); 214 paramMap.add("address-postalcode", theAddress_postalcode); 215 paramMap.add("address-state", theAddress_state); 216 paramMap.add("address-use", theAddress_use); 217 paramMap.add("endpoint", theEndpoint); 218 paramMap.add("identifier", theIdentifier); 219 paramMap.add("name", theName); 220 paramMap.add("partof", thePartof); 221 paramMap.add("phonetic", thePhonetic); 222 paramMap.add("type", theType); 223paramMap.setRevIncludes(theRevIncludes); 224 paramMap.setLastUpdated(the_lastUpdated); 225 paramMap.setIncludes(theIncludes); 226 paramMap.setSort(theSort); 227 paramMap.setCount(theCount); 228 paramMap.setOffset(theOffset); 229 paramMap.setSummaryMode(theSummaryMode); 230 paramMap.setSearchTotalMode(theSearchTotalMode); 231 paramMap.setSearchContainedMode(theSearchContainedMode); 232 233 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 234 235 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 236 return retVal; 237 } finally { 238 endRequest(theServletRequest); 239 } 240 } 241 242}