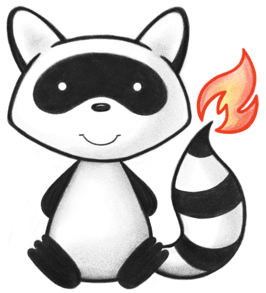
001 002package ca.uhn.fhir.jpa.rp.r4b; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r4b.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class LocationResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<Location> 021 { 022 023 @Override 024 public Class<Location> getResourceType() { 025 return Location.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 083 @OptionalParam(name="_lastUpdated") 084 DateRangeParam the_lastUpdated, 085 086 087 @Description(shortDefinition="The profile of the resource") 088 @OptionalParam(name="_profile") 089 UriAndListParam the_profile, 090 091 092 @Description(shortDefinition="The security of the resource") 093 @OptionalParam(name="_security") 094 TokenAndListParam the_security, 095 096 097 @Description(shortDefinition="The tag of the resource") 098 @OptionalParam(name="_tag") 099 TokenAndListParam the_tag, 100 101 102 @Description(shortDefinition="Search on the narrative of the resource") 103 @OptionalParam(name="_text") 104 StringAndListParam the_text, 105 106 107 @Description(shortDefinition="A (part of the) address of the location") 108 @OptionalParam(name="address") 109 StringAndListParam theAddress, 110 111 112 @Description(shortDefinition="A city specified in an address") 113 @OptionalParam(name="address-city") 114 StringAndListParam theAddress_city, 115 116 117 @Description(shortDefinition="A country specified in an address") 118 @OptionalParam(name="address-country") 119 StringAndListParam theAddress_country, 120 121 122 @Description(shortDefinition="A postal code specified in an address") 123 @OptionalParam(name="address-postalcode") 124 StringAndListParam theAddress_postalcode, 125 126 127 @Description(shortDefinition="A state specified in an address") 128 @OptionalParam(name="address-state") 129 StringAndListParam theAddress_state, 130 131 132 @Description(shortDefinition="A use code specified in an address") 133 @OptionalParam(name="address-use") 134 TokenAndListParam theAddress_use, 135 136 137 @Description(shortDefinition="Technical endpoints providing access to services operated for the location") 138 @OptionalParam(name="endpoint", targetTypes={ } ) 139 ReferenceAndListParam theEndpoint, 140 141 142 @Description(shortDefinition="An identifier for the location") 143 @OptionalParam(name="identifier") 144 TokenAndListParam theIdentifier, 145 146 147 @Description(shortDefinition="A portion of the location's name or alias") 148 @OptionalParam(name="name") 149 StringAndListParam theName, 150 151 152 @Description(shortDefinition="Search for locations where the location.position is near to, or within a specified distance of, the provided coordinates expressed as [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes).If the units are omitted, then kms should be assumed. If the distance is omitted, then the server can use its own discretion as to what distances should be considered near (and units are irrelevant)Servers may search using various techniques that might have differing accuracies, depending on implementation efficiency.Requires the near-distance parameter to be provided also") 153 @OptionalParam(name="near") 154 SpecialAndListParam theNear, 155 156 157 @Description(shortDefinition="Searches for locations (typically bed/room) that have an operational status (e.g. contaminated, housekeeping)") 158 @OptionalParam(name="operational-status") 159 TokenAndListParam theOperational_status, 160 161 162 @Description(shortDefinition="Searches for locations that are managed by the provided organization") 163 @OptionalParam(name="organization", targetTypes={ } ) 164 ReferenceAndListParam theOrganization, 165 166 167 @Description(shortDefinition="A location of which this location is a part") 168 @OptionalParam(name="partof", targetTypes={ } ) 169 ReferenceAndListParam thePartof, 170 171 172 @Description(shortDefinition="Searches for locations with a specific kind of status") 173 @OptionalParam(name="status") 174 TokenAndListParam theStatus, 175 176 177 @Description(shortDefinition="A code for the type of location") 178 @OptionalParam(name="type") 179 TokenAndListParam theType, 180 181 @RawParam 182 Map<String, List<String>> theAdditionalRawParams, 183 184 185 @IncludeParam 186 Set<Include> theIncludes, 187 188 @IncludeParam(reverse=true) 189 Set<Include> theRevIncludes, 190 191 @Sort 192 SortSpec theSort, 193 194 @ca.uhn.fhir.rest.annotation.Count 195 Integer theCount, 196 197 @ca.uhn.fhir.rest.annotation.Offset 198 Integer theOffset, 199 200 SummaryEnum theSummaryMode, 201 202 SearchTotalModeEnum theSearchTotalMode, 203 204 SearchContainedModeEnum theSearchContainedMode 205 206 ) { 207 startRequest(theServletRequest); 208 try { 209 SearchParameterMap paramMap = new SearchParameterMap(); 210 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 211 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 212 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 213 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 214 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 215 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 216 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 217 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 218 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 219 220 paramMap.add("_has", theHas); 221 paramMap.add("_id", the_id); 222 paramMap.add("_profile", the_profile); 223 paramMap.add("_security", the_security); 224 paramMap.add("_tag", the_tag); 225 paramMap.add("_text", the_text); 226 paramMap.add("address", theAddress); 227 paramMap.add("address-city", theAddress_city); 228 paramMap.add("address-country", theAddress_country); 229 paramMap.add("address-postalcode", theAddress_postalcode); 230 paramMap.add("address-state", theAddress_state); 231 paramMap.add("address-use", theAddress_use); 232 paramMap.add("endpoint", theEndpoint); 233 paramMap.add("identifier", theIdentifier); 234 paramMap.add("name", theName); 235 paramMap.add("near", theNear); 236 paramMap.add("operational-status", theOperational_status); 237 paramMap.add("organization", theOrganization); 238 paramMap.add("partof", thePartof); 239 paramMap.add("status", theStatus); 240 paramMap.add("type", theType); 241paramMap.setRevIncludes(theRevIncludes); 242 paramMap.setLastUpdated(the_lastUpdated); 243 paramMap.setIncludes(theIncludes); 244 paramMap.setSort(theSort); 245 paramMap.setCount(theCount); 246 paramMap.setOffset(theOffset); 247 paramMap.setSummaryMode(theSummaryMode); 248 paramMap.setSearchTotalMode(theSearchTotalMode); 249 paramMap.setSearchContainedMode(theSearchContainedMode); 250 251 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 252 253 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 254 return retVal; 255 } finally { 256 endRequest(theServletRequest); 257 } 258 } 259 260}