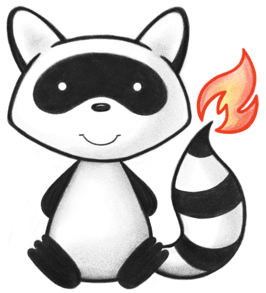
001 002package ca.uhn.fhir.jpa.rp.r4b; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r4b.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class ObservationResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProviderObservation<Observation> 021 { 022 023 @Override 024 public Class<Observation> getResourceType() { 025 return Observation.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 083 @OptionalParam(name="_lastUpdated") 084 DateRangeParam the_lastUpdated, 085 086 087 @Description(shortDefinition="The profile of the resource") 088 @OptionalParam(name="_profile") 089 UriAndListParam the_profile, 090 091 092 @Description(shortDefinition="The security of the resource") 093 @OptionalParam(name="_security") 094 TokenAndListParam the_security, 095 096 097 @Description(shortDefinition="The tag of the resource") 098 @OptionalParam(name="_tag") 099 TokenAndListParam the_tag, 100 101 102 @Description(shortDefinition="Search on the narrative of the resource") 103 @OptionalParam(name="_text") 104 StringAndListParam the_text, 105 106 107 @Description(shortDefinition="HGVS Protein Change") 108 @OptionalParam(name="amino-acid-change") 109 StringAndListParam theAmino_acid_change, 110 111 112 @Description(shortDefinition="Reference to the service request.") 113 @OptionalParam(name="based-on", targetTypes={ } ) 114 ReferenceAndListParam theBased_on, 115 116 117 @Description(shortDefinition="The classification of the type of observation") 118 @OptionalParam(name="category") 119 TokenAndListParam theCategory, 120 121 122 @Description(shortDefinition="Multiple Resources: * [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance* [Condition](condition.html): Code for the condition* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code* [List](list.html): What the purpose of this list is* [Medication](medication.html): Returns medications for a specific code* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code* [MedicationStatement](medicationstatement.html): Return statements of this medication code* [Observation](observation.html): The code of the observation type* [Procedure](procedure.html): A code to identify a procedure* [ServiceRequest](servicerequest.html): What is being requested/ordered") 123 @OptionalParam(name="code") 124 TokenAndListParam theCode, 125 126 127 @Description(shortDefinition="Code and coded value parameter pair") 128 @OptionalParam(name="code-value-concept", compositeTypes= { TokenParam.class, TokenParam.class }) 129 CompositeAndListParam<TokenParam, TokenParam> theCode_value_concept, 130 131 132 @Description(shortDefinition="Code and date/time value parameter pair") 133 @OptionalParam(name="code-value-date", compositeTypes= { TokenParam.class, DateParam.class }) 134 CompositeAndListParam<TokenParam, DateParam> theCode_value_date, 135 136 137 @Description(shortDefinition="Code and quantity value parameter pair") 138 @OptionalParam(name="code-value-quantity", compositeTypes= { TokenParam.class, QuantityParam.class }) 139 CompositeAndListParam<TokenParam, QuantityParam> theCode_value_quantity, 140 141 142 @Description(shortDefinition="Code and string value parameter pair") 143 @OptionalParam(name="code-value-string", compositeTypes= { TokenParam.class, StringParam.class }) 144 CompositeAndListParam<TokenParam, StringParam> theCode_value_string, 145 146 147 @Description(shortDefinition="The code of the observation type or component type") 148 @OptionalParam(name="combo-code") 149 TokenAndListParam theCombo_code, 150 151 152 @Description(shortDefinition="Code and coded value parameter pair, including in components") 153 @OptionalParam(name="combo-code-value-concept", compositeTypes= { TokenParam.class, TokenParam.class }) 154 CompositeAndListParam<TokenParam, TokenParam> theCombo_code_value_concept, 155 156 157 @Description(shortDefinition="Code and quantity value parameter pair, including in components") 158 @OptionalParam(name="combo-code-value-quantity", compositeTypes= { TokenParam.class, QuantityParam.class }) 159 CompositeAndListParam<TokenParam, QuantityParam> theCombo_code_value_quantity, 160 161 162 @Description(shortDefinition="The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.") 163 @OptionalParam(name="combo-data-absent-reason") 164 TokenAndListParam theCombo_data_absent_reason, 165 166 167 @Description(shortDefinition="The value or component value of the observation, if the value is a CodeableConcept") 168 @OptionalParam(name="combo-value-concept") 169 TokenAndListParam theCombo_value_concept, 170 171 172 @Description(shortDefinition="The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)") 173 @OptionalParam(name="combo-value-quantity") 174 QuantityAndListParam theCombo_value_quantity, 175 176 177 @Description(shortDefinition="The component code of the observation type") 178 @OptionalParam(name="component-code") 179 TokenAndListParam theComponent_code, 180 181 182 @Description(shortDefinition="Component code and component coded value parameter pair") 183 @OptionalParam(name="component-code-value-concept", compositeTypes= { TokenParam.class, TokenParam.class }) 184 CompositeAndListParam<TokenParam, TokenParam> theComponent_code_value_concept, 185 186 187 @Description(shortDefinition="Component code and component quantity value parameter pair") 188 @OptionalParam(name="component-code-value-quantity", compositeTypes= { TokenParam.class, QuantityParam.class }) 189 CompositeAndListParam<TokenParam, QuantityParam> theComponent_code_value_quantity, 190 191 192 @Description(shortDefinition="The reason why the expected value in the element Observation.component.value[x] is missing.") 193 @OptionalParam(name="component-data-absent-reason") 194 TokenAndListParam theComponent_data_absent_reason, 195 196 197 @Description(shortDefinition="The value of the component observation, if the value is a CodeableConcept") 198 @OptionalParam(name="component-value-concept") 199 TokenAndListParam theComponent_value_concept, 200 201 202 @Description(shortDefinition="The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)") 203 @OptionalParam(name="component-value-quantity") 204 QuantityAndListParam theComponent_value_quantity, 205 206 207 @Description(shortDefinition="The reason why the expected value in the element Observation.value[x] is missing.") 208 @OptionalParam(name="data-absent-reason") 209 TokenAndListParam theData_absent_reason, 210 211 212 @Description(shortDefinition="Multiple Resources: * [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded* [CarePlan](careplan.html): Time period plan covers* [CareTeam](careteam.html): Time period team covers* [ClinicalImpression](clinicalimpression.html): When the assessment was documented* [Composition](composition.html): Composition editing time* [Consent](consent.html): When this Consent was created or indexed* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report* [Encounter](encounter.html): A date within the period the Encounter lasted* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated* [Flag](flag.html): Time period when flag is active* [Immunization](immunization.html): Vaccination (non)-Administration Date* [List](list.html): When the list was prepared* [Observation](observation.html): Obtained date/time. If the obtained element is a period, a date that falls in the period* [Procedure](procedure.html): When the procedure was performed* [RiskAssessment](riskassessment.html): When was assessment made?* [SupplyRequest](supplyrequest.html): When the request was made") 213 @OptionalParam(name="date") 214 DateRangeParam theDate, 215 216 217 @Description(shortDefinition="Related measurements the observation is made from") 218 @OptionalParam(name="derived-from", targetTypes={ } ) 219 ReferenceAndListParam theDerived_from, 220 221 222 @Description(shortDefinition="The Device that generated the observation data.") 223 @OptionalParam(name="device", targetTypes={ } ) 224 ReferenceAndListParam theDevice, 225 226 227 @Description(shortDefinition="HGVS DNA variant") 228 @OptionalParam(name="dna-variant") 229 StringAndListParam theDna_variant, 230 231 232 @Description(shortDefinition="Multiple Resources: * [Composition](composition.html): Context of the Composition* [DeviceRequest](devicerequest.html): Encounter during which request was created* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made* [DocumentReference](documentreference.html): Context of the document content* [Flag](flag.html): Alert relevant during encounter* [List](list.html): Context in which list created* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier* [Observation](observation.html): Encounter related to the observation* [Procedure](procedure.html): Encounter created as part of* [RiskAssessment](riskassessment.html): Where was assessment performed?* [ServiceRequest](servicerequest.html): An encounter in which this request is made* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier") 233 @OptionalParam(name="encounter", targetTypes={ } ) 234 ReferenceAndListParam theEncounter, 235 236 237 @Description(shortDefinition="The focus of an observation when the focus is not the patient of record.") 238 @OptionalParam(name="focus", targetTypes={ } ) 239 ReferenceAndListParam theFocus, 240 241 242 @Description(shortDefinition="HGNC gene symbol and HGVS Protein change") 243 @OptionalParam(name="gene-amino-acid-change") 244 StringAndListParam theGene_amino_acid_change, 245 246 247 @Description(shortDefinition="HGNC gene symbol and HGVS DNA Variant") 248 @OptionalParam(name="gene-dnavariant") 249 StringAndListParam theGene_dnavariant, 250 251 252 @Description(shortDefinition="HGNC gene symbol and identifier") 253 @OptionalParam(name="gene-identifier") 254 TokenAndListParam theGene_identifier, 255 256 257 @Description(shortDefinition="Related resource that belongs to the Observation group") 258 @OptionalParam(name="has-member", targetTypes={ } ) 259 ReferenceAndListParam theHas_member, 260 261 262 @Description(shortDefinition="Multiple Resources: * [AllergyIntolerance](allergyintolerance.html): External ids for this item* [CarePlan](careplan.html): External Ids for this plan* [CareTeam](careteam.html): External Ids for this team* [Composition](composition.html): Version-independent identifier for the Composition* [Condition](condition.html): A unique identifier of the condition record* [Consent](consent.html): Identifier for this record (external references)* [DetectedIssue](detectedissue.html): Unique id for the detected issue* [DeviceRequest](devicerequest.html): Business identifier for request/order* [DiagnosticReport](diagnosticreport.html): An identifier for the report* [DocumentManifest](documentmanifest.html): Unique Identifier for the set of documents* [DocumentReference](documentreference.html): Master Version Specific Identifier* [Encounter](encounter.html): Identifier(s) by which this encounter is known* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier* [Goal](goal.html): External Ids for this goal* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID and Accession number* [Immunization](immunization.html): Business identifier* [List](list.html): Business identifier* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier* [MedicationStatement](medicationstatement.html): Return statements with this external identifier* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier* [Observation](observation.html): The unique id for a particular observation* [Procedure](procedure.html): A unique identifier for a procedure* [RiskAssessment](riskassessment.html): Unique identifier for the assessment* [ServiceRequest](servicerequest.html): Identifiers assigned to this order* [SupplyDelivery](supplydelivery.html): External identifier* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier") 263 @OptionalParam(name="identifier") 264 TokenAndListParam theIdentifier, 265 266 267 @Description(shortDefinition="The method used for the observation") 268 @OptionalParam(name="method") 269 TokenAndListParam theMethod, 270 271 272 @Description(shortDefinition="Part of referenced event") 273 @OptionalParam(name="part-of", targetTypes={ } ) 274 ReferenceAndListParam thePart_of, 275 276 277 @Description(shortDefinition="Multiple Resources: * [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for* [CarePlan](careplan.html): Who the care plan is for* [CareTeam](careteam.html): Who care team is for* [ClinicalImpression](clinicalimpression.html): Patient or group assessed* [Composition](composition.html): Who and/or what the composition is about* [Condition](condition.html): Who has the condition?* [Consent](consent.html): Who the consent applies to* [DetectedIssue](detectedissue.html): Associated patient* [DeviceRequest](devicerequest.html): Individual the service is ordered for* [DeviceUseStatement](deviceusestatement.html): Search by subject - a patient* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient* [DocumentManifest](documentmanifest.html): The subject of the set of documents* [DocumentReference](documentreference.html): Who/what is the subject of the document* [Encounter](encounter.html): The patient or group present at the encounter* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for* [Flag](flag.html): The identity of a subject to list flags for* [Goal](goal.html): Who this goal is intended for* [ImagingStudy](imagingstudy.html): Who the study is about* [Immunization](immunization.html): The patient for the vaccination record* [List](list.html): If all resources have the same subject* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.* [NutritionOrder](nutritionorder.html): The identity of the person who requires the diet, formula or nutritional supplement* [Observation](observation.html): The subject that the observation is about (if patient)* [Procedure](procedure.html): Search by subject - a patient* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?* [ServiceRequest](servicerequest.html): Search by subject - a patient* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for") 278 @OptionalParam(name="patient", targetTypes={ } ) 279 ReferenceAndListParam thePatient, 280 281 282 @Description(shortDefinition="Who performed the observation") 283 @OptionalParam(name="performer", targetTypes={ } ) 284 ReferenceAndListParam thePerformer, 285 286 287 @Description(shortDefinition="Specimen used for this observation") 288 @OptionalParam(name="specimen", targetTypes={ } ) 289 ReferenceAndListParam theSpecimen, 290 291 292 @Description(shortDefinition="The status of the observation") 293 @OptionalParam(name="status") 294 TokenAndListParam theStatus, 295 296 297 @Description(shortDefinition="The subject that the observation is about") 298 @OptionalParam(name="subject", targetTypes={ } ) 299 ReferenceAndListParam theSubject, 300 301 302 @Description(shortDefinition="The value of the observation, if the value is a CodeableConcept") 303 @OptionalParam(name="value-concept") 304 TokenAndListParam theValue_concept, 305 306 307 @Description(shortDefinition="The value of the observation, if the value is a date or period of time") 308 @OptionalParam(name="value-date") 309 DateRangeParam theValue_date, 310 311 312 @Description(shortDefinition="The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)") 313 @OptionalParam(name="value-quantity") 314 QuantityAndListParam theValue_quantity, 315 316 317 @Description(shortDefinition="The value of the observation, if the value is a string, and also searches in CodeableConcept.text") 318 @OptionalParam(name="value-string") 319 StringAndListParam theValue_string, 320 321 @RawParam 322 Map<String, List<String>> theAdditionalRawParams, 323 324 325 @IncludeParam 326 Set<Include> theIncludes, 327 328 @IncludeParam(reverse=true) 329 Set<Include> theRevIncludes, 330 331 @Sort 332 SortSpec theSort, 333 334 @ca.uhn.fhir.rest.annotation.Count 335 Integer theCount, 336 337 @ca.uhn.fhir.rest.annotation.Offset 338 Integer theOffset, 339 340 SummaryEnum theSummaryMode, 341 342 SearchTotalModeEnum theSearchTotalMode, 343 344 SearchContainedModeEnum theSearchContainedMode 345 346 ) { 347 startRequest(theServletRequest); 348 try { 349 SearchParameterMap paramMap = new SearchParameterMap(); 350 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 351 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 352 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 353 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 354 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 355 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 356 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 357 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 358 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 359 360 paramMap.add("_has", theHas); 361 paramMap.add("_id", the_id); 362 paramMap.add("_profile", the_profile); 363 paramMap.add("_security", the_security); 364 paramMap.add("_tag", the_tag); 365 paramMap.add("_text", the_text); 366 paramMap.add("amino-acid-change", theAmino_acid_change); 367 paramMap.add("based-on", theBased_on); 368 paramMap.add("category", theCategory); 369 paramMap.add("code", theCode); 370 paramMap.add("code-value-concept", theCode_value_concept); 371 paramMap.add("code-value-date", theCode_value_date); 372 paramMap.add("code-value-quantity", theCode_value_quantity); 373 paramMap.add("code-value-string", theCode_value_string); 374 paramMap.add("combo-code", theCombo_code); 375 paramMap.add("combo-code-value-concept", theCombo_code_value_concept); 376 paramMap.add("combo-code-value-quantity", theCombo_code_value_quantity); 377 paramMap.add("combo-data-absent-reason", theCombo_data_absent_reason); 378 paramMap.add("combo-value-concept", theCombo_value_concept); 379 paramMap.add("combo-value-quantity", theCombo_value_quantity); 380 paramMap.add("component-code", theComponent_code); 381 paramMap.add("component-code-value-concept", theComponent_code_value_concept); 382 paramMap.add("component-code-value-quantity", theComponent_code_value_quantity); 383 paramMap.add("component-data-absent-reason", theComponent_data_absent_reason); 384 paramMap.add("component-value-concept", theComponent_value_concept); 385 paramMap.add("component-value-quantity", theComponent_value_quantity); 386 paramMap.add("data-absent-reason", theData_absent_reason); 387 paramMap.add("date", theDate); 388 paramMap.add("derived-from", theDerived_from); 389 paramMap.add("device", theDevice); 390 paramMap.add("dna-variant", theDna_variant); 391 paramMap.add("encounter", theEncounter); 392 paramMap.add("focus", theFocus); 393 paramMap.add("gene-amino-acid-change", theGene_amino_acid_change); 394 paramMap.add("gene-dnavariant", theGene_dnavariant); 395 paramMap.add("gene-identifier", theGene_identifier); 396 paramMap.add("has-member", theHas_member); 397 paramMap.add("identifier", theIdentifier); 398 paramMap.add("method", theMethod); 399 paramMap.add("part-of", thePart_of); 400 paramMap.add("patient", thePatient); 401 paramMap.add("performer", thePerformer); 402 paramMap.add("specimen", theSpecimen); 403 paramMap.add("status", theStatus); 404 paramMap.add("subject", theSubject); 405 paramMap.add("value-concept", theValue_concept); 406 paramMap.add("value-date", theValue_date); 407 paramMap.add("value-quantity", theValue_quantity); 408 paramMap.add("value-string", theValue_string); 409paramMap.setRevIncludes(theRevIncludes); 410 paramMap.setLastUpdated(the_lastUpdated); 411 paramMap.setIncludes(theIncludes); 412 paramMap.setSort(theSort); 413 paramMap.setCount(theCount); 414 paramMap.setOffset(theOffset); 415 paramMap.setSummaryMode(theSummaryMode); 416 paramMap.setSearchTotalMode(theSearchTotalMode); 417 paramMap.setSearchContainedMode(theSearchContainedMode); 418 419 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 420 421 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 422 return retVal; 423 } finally { 424 endRequest(theServletRequest); 425 } 426 } 427 428}