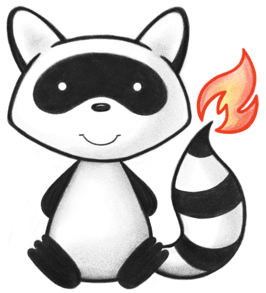
001 002package ca.uhn.fhir.jpa.rp.r4b; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r4b.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class PatientResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProviderPatient<Patient> 021 { 022 023 @Override 024 public Class<Patient> getResourceType() { 025 return Patient.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 083 @OptionalParam(name="_lastUpdated") 084 DateRangeParam the_lastUpdated, 085 086 087 @Description(shortDefinition="The profile of the resource") 088 @OptionalParam(name="_profile") 089 UriAndListParam the_profile, 090 091 092 @Description(shortDefinition="The security of the resource") 093 @OptionalParam(name="_security") 094 TokenAndListParam the_security, 095 096 097 @Description(shortDefinition="The tag of the resource") 098 @OptionalParam(name="_tag") 099 TokenAndListParam the_tag, 100 101 102 @Description(shortDefinition="Search on the narrative of the resource") 103 @OptionalParam(name="_text") 104 StringAndListParam the_text, 105 106 107 @Description(shortDefinition="Whether the patient record is active") 108 @OptionalParam(name="active") 109 TokenAndListParam theActive, 110 111 112 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text") 113 @OptionalParam(name="address") 114 StringAndListParam theAddress, 115 116 117 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A city specified in an address* [Person](person.html): A city specified in an address* [Practitioner](practitioner.html): A city specified in an address* [RelatedPerson](relatedperson.html): A city specified in an address") 118 @OptionalParam(name="address-city") 119 StringAndListParam theAddress_city, 120 121 122 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A country specified in an address* [Person](person.html): A country specified in an address* [Practitioner](practitioner.html): A country specified in an address* [RelatedPerson](relatedperson.html): A country specified in an address") 123 @OptionalParam(name="address-country") 124 StringAndListParam theAddress_country, 125 126 127 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A postalCode specified in an address* [Person](person.html): A postal code specified in an address* [Practitioner](practitioner.html): A postalCode specified in an address* [RelatedPerson](relatedperson.html): A postal code specified in an address") 128 @OptionalParam(name="address-postalcode") 129 StringAndListParam theAddress_postalcode, 130 131 132 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A state specified in an address* [Person](person.html): A state specified in an address* [Practitioner](practitioner.html): A state specified in an address* [RelatedPerson](relatedperson.html): A state specified in an address") 133 @OptionalParam(name="address-state") 134 StringAndListParam theAddress_state, 135 136 137 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A use code specified in an address* [Person](person.html): A use code specified in an address* [Practitioner](practitioner.html): A use code specified in an address* [RelatedPerson](relatedperson.html): A use code specified in an address") 138 @OptionalParam(name="address-use") 139 TokenAndListParam theAddress_use, 140 141 142 @Description(shortDefinition="Searches for patients based on age as calculated based on current date and date of birth. Deceased patients are excluded from the search.") 143 @OptionalParam(name="age") 144 NumberAndListParam theAge, 145 146 147 @Description(shortDefinition="Search based on whether a patient was part of a multiple birth or not.") 148 @OptionalParam(name="birthOrderBoolean") 149 TokenAndListParam theBirthOrderBoolean, 150 151 152 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): The patient's date of birth* [Person](person.html): The person's date of birth* [RelatedPerson](relatedperson.html): The Related Person's date of birth") 153 @OptionalParam(name="birthdate") 154 DateRangeParam theBirthdate, 155 156 157 @Description(shortDefinition="The date of death has been provided and satisfies this search value") 158 @OptionalParam(name="death-date") 159 DateRangeParam theDeath_date, 160 161 162 @Description(shortDefinition="This patient has been marked as deceased, or as a death date entered") 163 @OptionalParam(name="deceased") 164 TokenAndListParam theDeceased, 165 166 167 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A value in an email contact* [Person](person.html): A value in an email contact* [Practitioner](practitioner.html): A value in an email contact* [PractitionerRole](practitionerrole.html): A value in an email contact* [RelatedPerson](relatedperson.html): A value in an email contact") 168 @OptionalParam(name="email") 169 TokenAndListParam theEmail, 170 171 172 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A portion of the family name of the patient* [Practitioner](practitioner.html): A portion of the family name") 173 @OptionalParam(name="family") 174 StringAndListParam theFamily, 175 176 177 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): Gender of the patient* [Person](person.html): The gender of the person* [Practitioner](practitioner.html): Gender of the practitioner* [RelatedPerson](relatedperson.html): Gender of the related person") 178 @OptionalParam(name="gender") 179 TokenAndListParam theGender, 180 181 182 @Description(shortDefinition="Patient's nominated general practitioner, not the organization that manages the record") 183 @OptionalParam(name="general-practitioner", targetTypes={ } ) 184 ReferenceAndListParam theGeneral_practitioner, 185 186 187 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A portion of the given name of the patient* [Practitioner](practitioner.html): A portion of the given name") 188 @OptionalParam(name="given") 189 StringAndListParam theGiven, 190 191 192 @Description(shortDefinition="A patient identifier") 193 @OptionalParam(name="identifier") 194 TokenAndListParam theIdentifier, 195 196 197 @Description(shortDefinition="Language code (irrespective of use value)") 198 @OptionalParam(name="language") 199 TokenAndListParam theLanguage, 200 201 202 @Description(shortDefinition="All patients linked to the given patient") 203 @OptionalParam(name="link", targetTypes={ } ) 204 ReferenceAndListParam theLink, 205 206 207 @Description(shortDefinition="Search based on patient's mother's maiden name") 208 @OptionalParam(name="mothersMaidenName") 209 StringAndListParam theMothersMaidenName, 210 211 212 @Description(shortDefinition="A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text") 213 @OptionalParam(name="name") 214 StringAndListParam theName, 215 216 217 @Description(shortDefinition="The organization that is the custodian of the patient record") 218 @OptionalParam(name="organization", targetTypes={ } ) 219 ReferenceAndListParam theOrganization, 220 221 222 @Description(shortDefinition="Search by url for a participation agreement, which is stored in a DocumentReference") 223 @OptionalParam(name="part-agree", targetTypes={ } ) 224 ReferenceAndListParam thePart_agree, 225 226 227 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A value in a phone contact* [Person](person.html): A value in a phone contact* [Practitioner](practitioner.html): A value in a phone contact* [PractitionerRole](practitionerrole.html): A value in a phone contact* [RelatedPerson](relatedperson.html): A value in a phone contact") 228 @OptionalParam(name="phone") 229 TokenAndListParam thePhone, 230 231 232 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm* [Person](person.html): A portion of name using some kind of phonetic matching algorithm* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm") 233 @OptionalParam(name="phonetic") 234 StringAndListParam thePhonetic, 235 236 237 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): The value in any kind of telecom details of the patient* [Person](person.html): The value in any kind of contact* [Practitioner](practitioner.html): The value in any kind of contact* [PractitionerRole](practitionerrole.html): The value in any kind of contact* [RelatedPerson](relatedperson.html): The value in any kind of contact") 238 @OptionalParam(name="telecom") 239 TokenAndListParam theTelecom, 240 241 @RawParam 242 Map<String, List<String>> theAdditionalRawParams, 243 244 245 @IncludeParam 246 Set<Include> theIncludes, 247 248 @IncludeParam(reverse=true) 249 Set<Include> theRevIncludes, 250 251 @Sort 252 SortSpec theSort, 253 254 @ca.uhn.fhir.rest.annotation.Count 255 Integer theCount, 256 257 @ca.uhn.fhir.rest.annotation.Offset 258 Integer theOffset, 259 260 SummaryEnum theSummaryMode, 261 262 SearchTotalModeEnum theSearchTotalMode, 263 264 SearchContainedModeEnum theSearchContainedMode 265 266 ) { 267 startRequest(theServletRequest); 268 try { 269 SearchParameterMap paramMap = new SearchParameterMap(); 270 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 271 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 272 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 273 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 274 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 275 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 276 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 277 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 278 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 279 280 paramMap.add("_has", theHas); 281 paramMap.add("_id", the_id); 282 paramMap.add("_profile", the_profile); 283 paramMap.add("_security", the_security); 284 paramMap.add("_tag", the_tag); 285 paramMap.add("_text", the_text); 286 paramMap.add("active", theActive); 287 paramMap.add("address", theAddress); 288 paramMap.add("address-city", theAddress_city); 289 paramMap.add("address-country", theAddress_country); 290 paramMap.add("address-postalcode", theAddress_postalcode); 291 paramMap.add("address-state", theAddress_state); 292 paramMap.add("address-use", theAddress_use); 293 paramMap.add("age", theAge); 294 paramMap.add("birthOrderBoolean", theBirthOrderBoolean); 295 paramMap.add("birthdate", theBirthdate); 296 paramMap.add("death-date", theDeath_date); 297 paramMap.add("deceased", theDeceased); 298 paramMap.add("email", theEmail); 299 paramMap.add("family", theFamily); 300 paramMap.add("gender", theGender); 301 paramMap.add("general-practitioner", theGeneral_practitioner); 302 paramMap.add("given", theGiven); 303 paramMap.add("identifier", theIdentifier); 304 paramMap.add("language", theLanguage); 305 paramMap.add("link", theLink); 306 paramMap.add("mothersMaidenName", theMothersMaidenName); 307 paramMap.add("name", theName); 308 paramMap.add("organization", theOrganization); 309 paramMap.add("part-agree", thePart_agree); 310 paramMap.add("phone", thePhone); 311 paramMap.add("phonetic", thePhonetic); 312 paramMap.add("telecom", theTelecom); 313paramMap.setRevIncludes(theRevIncludes); 314 paramMap.setLastUpdated(the_lastUpdated); 315 paramMap.setIncludes(theIncludes); 316 paramMap.setSort(theSort); 317 paramMap.setCount(theCount); 318 paramMap.setOffset(theOffset); 319 paramMap.setSummaryMode(theSummaryMode); 320 paramMap.setSearchTotalMode(theSearchTotalMode); 321 paramMap.setSearchContainedMode(theSearchContainedMode); 322 323 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 324 325 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 326 return retVal; 327 } finally { 328 endRequest(theServletRequest); 329 } 330 } 331 332}