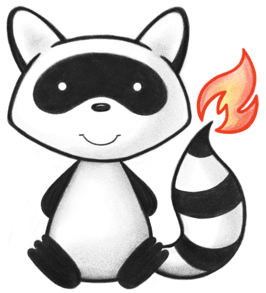
001 002package ca.uhn.fhir.jpa.rp.r5; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r5.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class ConsentResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<Consent> 021 { 022 023 @Override 024 public Class<Consent> getResourceType() { 025 return Consent.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 083 @OptionalParam(name="_lastUpdated") 084 DateRangeParam the_lastUpdated, 085 086 087 @Description(shortDefinition="The profile of the resource") 088 @OptionalParam(name="_profile") 089 UriAndListParam the_profile, 090 091 092 @Description(shortDefinition="The security of the resource") 093 @OptionalParam(name="_security") 094 TokenAndListParam the_security, 095 096 097 @Description(shortDefinition="The tag of the resource") 098 @OptionalParam(name="_tag") 099 TokenAndListParam the_tag, 100 101 102 @Description(shortDefinition="Search on the narrative of the resource") 103 @OptionalParam(name="_text") 104 SpecialAndListParam the_text, 105 106 107 @Description(shortDefinition="Actions controlled by this rule") 108 @OptionalParam(name="action") 109 TokenAndListParam theAction, 110 111 112 @Description(shortDefinition="Resource for the actor (or group, by role)") 113 @OptionalParam(name="actor", targetTypes={ } ) 114 ReferenceAndListParam theActor, 115 116 117 @Description(shortDefinition="Classification of the consent statement - for indexing/retrieval") 118 @OptionalParam(name="category") 119 TokenAndListParam theCategory, 120 121 122 @Description(shortDefinition="Consent Enforcer") 123 @OptionalParam(name="controller", targetTypes={ } ) 124 ReferenceAndListParam theController, 125 126 127 @Description(shortDefinition="The actual data reference") 128 @OptionalParam(name="data", targetTypes={ } ) 129 ReferenceAndListParam theData, 130 131 132 @Description(shortDefinition="Multiple Resources: * [AdverseEvent](adverseevent.html): When the event occurred* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded* [Appointment](appointment.html): Appointment date/time.* [AuditEvent](auditevent.html): Time when the event was recorded* [CarePlan](careplan.html): Time period plan covers* [CareTeam](careteam.html): A date within the coverage time period.* [ClinicalImpression](clinicalimpression.html): When the assessment was documented* [Composition](composition.html): Composition editing time* [Consent](consent.html): When consent was agreed to* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report* [DocumentReference](documentreference.html): When this document reference was created* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated* [Flag](flag.html): Time period when flag is active* [Immunization](immunization.html): Vaccination (non)-Administration Date* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created* [Invoice](invoice.html): Invoice date / posting date* [List](list.html): When the list was prepared* [MeasureReport](measurereport.html): The date of the measure report* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication* [Observation](observation.html): Clinically relevant time/time-period for observation* [Procedure](procedure.html): When the procedure occurred or is occurring* [ResearchSubject](researchsubject.html): Start and end of participation* [RiskAssessment](riskassessment.html): When was assessment made?* [SupplyRequest](supplyrequest.html): When the request was made") 133 @OptionalParam(name="date") 134 DateRangeParam theDate, 135 136 137 @Description(shortDefinition="Who is agreeing to the policy and rules") 138 @OptionalParam(name="grantee", targetTypes={ } ) 139 ReferenceAndListParam theGrantee, 140 141 142 @Description(shortDefinition="Multiple Resources: * [Account](account.html): Account number* [AdverseEvent](adverseevent.html): Business identifier for the event* [AllergyIntolerance](allergyintolerance.html): External ids for this item* [Appointment](appointment.html): An Identifier of the Appointment* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response* [Basic](basic.html): Business identifier* [BodyStructure](bodystructure.html): Bodystructure identifier* [CarePlan](careplan.html): External Ids for this plan* [CareTeam](careteam.html): External Ids for this team* [ChargeItem](chargeitem.html): Business Identifier for item* [Claim](claim.html): The primary identifier of the financial resource* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse* [ClinicalImpression](clinicalimpression.html): Business identifier* [Communication](communication.html): Unique identifier* [CommunicationRequest](communicationrequest.html): Unique identifier* [Composition](composition.html): Version-independent identifier for the Composition* [Condition](condition.html): A unique identifier of the condition record* [Consent](consent.html): Identifier for this record (external references)* [Contract](contract.html): The identity of the contract* [Coverage](coverage.html): The primary identifier of the insured and the coverage* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier* [DetectedIssue](detectedissue.html): Unique id for the detected issue* [DeviceRequest](devicerequest.html): Business identifier for request/order* [DeviceUsage](deviceusage.html): Search by identifier* [DiagnosticReport](diagnosticreport.html): An identifier for the report* [DocumentReference](documentreference.html): Identifier of the attachment binary* [Encounter](encounter.html): Identifier(s) by which this encounter is known* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier* [Flag](flag.html): Business identifier* [Goal](goal.html): External Ids for this goal* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID* [Immunization](immunization.html): Business identifier* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier* [Invoice](invoice.html): Business Identifier for item* [List](list.html): Business identifier* [MeasureReport](measurereport.html): External identifier of the measure report to be returned* [Medication](medication.html): Returns medications with this external identifier* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier* [MedicationStatement](medicationstatement.html): Return statements with this external identifier* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence* [NutritionIntake](nutritionintake.html): Return statements with this external identifier* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier* [Observation](observation.html): The unique id for a particular observation* [Person](person.html): A person Identifier* [Procedure](procedure.html): A unique identifier for a procedure* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study* [RiskAssessment](riskassessment.html): Unique identifier for the assessment* [ServiceRequest](servicerequest.html): Identifiers assigned to this order* [Specimen](specimen.html): The unique identifier associated with the specimen* [SupplyDelivery](supplydelivery.html): External identifier* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest* [Task](task.html): Search for a task instance by its business identifier* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier") 143 @OptionalParam(name="identifier") 144 TokenAndListParam theIdentifier, 145 146 147 @Description(shortDefinition="Consent workflow management") 148 @OptionalParam(name="manager", targetTypes={ } ) 149 ReferenceAndListParam theManager, 150 151 152 @Description(shortDefinition="Multiple Resources: * [Account](account.html): The entity that caused the expenses* [AdverseEvent](adverseevent.html): Subject impacted by event* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for* [Appointment](appointment.html): One of the individuals of the appointment is this patient* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient* [AuditEvent](auditevent.html): Where the activity involved patient data* [Basic](basic.html): Identifies the focus of this resource* [BodyStructure](bodystructure.html): Who this is about* [CarePlan](careplan.html): Who the care plan is for* [CareTeam](careteam.html): Who care team is for* [ChargeItem](chargeitem.html): Individual service was done for/to* [Claim](claim.html): Patient receiving the products or services* [ClaimResponse](claimresponse.html): The subject of care* [ClinicalImpression](clinicalimpression.html): Patient assessed* [Communication](communication.html): Focus of message* [CommunicationRequest](communicationrequest.html): Focus of message* [Composition](composition.html): Who and/or what the composition is about* [Condition](condition.html): Who has the condition?* [Consent](consent.html): Who the consent applies to* [Contract](contract.html): The identity of the subject of the contract (if a patient)* [Coverage](coverage.html): Retrieve coverages for a patient* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient* [DetectedIssue](detectedissue.html): Associated patient* [DeviceRequest](devicerequest.html): Individual the service is ordered for* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient* [DocumentReference](documentreference.html): Who/what is the subject of the document* [Encounter](encounter.html): The patient present at the encounter* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for* [Flag](flag.html): The identity of a subject to list flags for* [Goal](goal.html): Who this goal is intended for* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results* [ImagingSelection](imagingselection.html): Who the study is about* [ImagingStudy](imagingstudy.html): Who the study is about* [Immunization](immunization.html): The patient for the vaccination record* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for* [Invoice](invoice.html): Recipient(s) of goods and services* [List](list.html): If all resources have the same subject* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.* [MolecularSequence](molecularsequence.html): The subject that the sequence is about* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement* [Observation](observation.html): The subject that the observation is about (if patient)* [Person](person.html): The Person links to this Patient* [Procedure](procedure.html): Search by subject - a patient* [Provenance](provenance.html): Where the activity involved patient data* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response* [RelatedPerson](relatedperson.html): The patient this related person is related to* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations* [ResearchSubject](researchsubject.html): Who or what is part of study* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?* [ServiceRequest](servicerequest.html): Search by subject - a patient* [Specimen](specimen.html): The patient the specimen comes from* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined* [Task](task.html): Search by patient* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for") 153 @OptionalParam(name="patient", targetTypes={ } ) 154 ReferenceAndListParam thePatient, 155 156 157 @Description(shortDefinition="Timeframe for this rule") 158 @OptionalParam(name="period") 159 DateRangeParam thePeriod, 160 161 162 @Description(shortDefinition="Context of activities covered by this rule") 163 @OptionalParam(name="purpose") 164 TokenAndListParam thePurpose, 165 166 167 @Description(shortDefinition="Security Labels that define affected resources") 168 @OptionalParam(name="security-label") 169 TokenAndListParam theSecurity_label, 170 171 172 @Description(shortDefinition="Search by reference to a Consent, DocumentReference, Contract or QuestionnaireResponse") 173 @OptionalParam(name="source-reference", targetTypes={ } ) 174 ReferenceAndListParam theSource_reference, 175 176 177 @Description(shortDefinition="draft | active | inactive | entered-in-error | unknown") 178 @OptionalParam(name="status") 179 TokenAndListParam theStatus, 180 181 182 @Description(shortDefinition="Who the consent applies to") 183 @OptionalParam(name="subject", targetTypes={ } ) 184 ReferenceAndListParam theSubject, 185 186 187 @Description(shortDefinition="Has been verified") 188 @OptionalParam(name="verified") 189 TokenAndListParam theVerified, 190 191 192 @Description(shortDefinition="When consent verified") 193 @OptionalParam(name="verified-date") 194 DateRangeParam theVerified_date, 195 196 @RawParam 197 Map<String, List<String>> theAdditionalRawParams, 198 199 200 @IncludeParam 201 Set<Include> theIncludes, 202 203 @IncludeParam(reverse=true) 204 Set<Include> theRevIncludes, 205 206 @Sort 207 SortSpec theSort, 208 209 @ca.uhn.fhir.rest.annotation.Count 210 Integer theCount, 211 212 @ca.uhn.fhir.rest.annotation.Offset 213 Integer theOffset, 214 215 SummaryEnum theSummaryMode, 216 217 SearchTotalModeEnum theSearchTotalMode, 218 219 SearchContainedModeEnum theSearchContainedMode 220 221 ) { 222 startRequest(theServletRequest); 223 try { 224 SearchParameterMap paramMap = new SearchParameterMap(); 225 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 226 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 227 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 228 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 229 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 230 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 231 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 232 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 233 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 234 235 paramMap.add("_has", theHas); 236 paramMap.add("_id", the_id); 237 paramMap.add("_profile", the_profile); 238 paramMap.add("_security", the_security); 239 paramMap.add("_tag", the_tag); 240 paramMap.add("_text", the_text); 241 paramMap.add("action", theAction); 242 paramMap.add("actor", theActor); 243 paramMap.add("category", theCategory); 244 paramMap.add("controller", theController); 245 paramMap.add("data", theData); 246 paramMap.add("date", theDate); 247 paramMap.add("grantee", theGrantee); 248 paramMap.add("identifier", theIdentifier); 249 paramMap.add("manager", theManager); 250 paramMap.add("patient", thePatient); 251 paramMap.add("period", thePeriod); 252 paramMap.add("purpose", thePurpose); 253 paramMap.add("security-label", theSecurity_label); 254 paramMap.add("source-reference", theSource_reference); 255 paramMap.add("status", theStatus); 256 paramMap.add("subject", theSubject); 257 paramMap.add("verified", theVerified); 258 paramMap.add("verified-date", theVerified_date); 259paramMap.setRevIncludes(theRevIncludes); 260 paramMap.setLastUpdated(the_lastUpdated); 261 paramMap.setIncludes(theIncludes); 262 paramMap.setSort(theSort); 263 paramMap.setCount(theCount); 264 paramMap.setOffset(theOffset); 265 paramMap.setSummaryMode(theSummaryMode); 266 paramMap.setSearchTotalMode(theSearchTotalMode); 267 paramMap.setSearchContainedMode(theSearchContainedMode); 268 269 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 270 271 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 272 return retVal; 273 } finally { 274 endRequest(theServletRequest); 275 } 276 } 277 278}