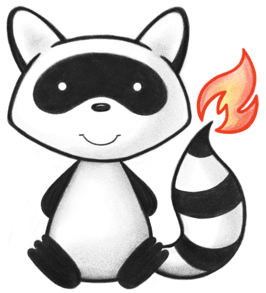
001 002package ca.uhn.fhir.jpa.rp.r5; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r5.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class DeviceResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<Device> 021 { 022 023 @Override 024 public Class<Device> getResourceType() { 025 return Device.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 083 @OptionalParam(name="_lastUpdated") 084 DateRangeParam the_lastUpdated, 085 086 087 @Description(shortDefinition="The profile of the resource") 088 @OptionalParam(name="_profile") 089 UriAndListParam the_profile, 090 091 092 @Description(shortDefinition="The security of the resource") 093 @OptionalParam(name="_security") 094 TokenAndListParam the_security, 095 096 097 @Description(shortDefinition="The tag of the resource") 098 @OptionalParam(name="_tag") 099 TokenAndListParam the_tag, 100 101 102 @Description(shortDefinition="Search on the narrative of the resource") 103 @OptionalParam(name="_text") 104 SpecialAndListParam the_text, 105 106 107 @Description(shortDefinition="The biological source for the device") 108 @OptionalParam(name="biological-source-event") 109 TokenAndListParam theBiological_source_event, 110 111 112 @Description(shortDefinition="The definition / type of the device (code)") 113 @OptionalParam(name="code") 114 TokenAndListParam theCode, 115 116 117 @Description(shortDefinition="Code and value parameter pair") 118 @OptionalParam(name="code-value-concept", compositeTypes= { TokenParam.class, StringParam.class }) 119 CompositeAndListParam<TokenParam, StringParam> theCode_value_concept, 120 121 122 @Description(shortDefinition="The definition / type of the device") 123 @OptionalParam(name="definition", targetTypes={ } ) 124 ReferenceAndListParam theDefinition, 125 126 127 @Description(shortDefinition="A server defined search that may match any of the string fields in Device.name or Device.type.") 128 @OptionalParam(name="device-name") 129 StringAndListParam theDevice_name, 130 131 132 @Description(shortDefinition="The expiration date of the device") 133 @OptionalParam(name="expiration-date") 134 DateRangeParam theExpiration_date, 135 136 137 @Description(shortDefinition="Instance id from manufacturer, owner, and others") 138 @OptionalParam(name="identifier") 139 TokenAndListParam theIdentifier, 140 141 142 @Description(shortDefinition="A location, where the resource is found") 143 @OptionalParam(name="location", targetTypes={ } ) 144 ReferenceAndListParam theLocation, 145 146 147 @Description(shortDefinition="The lot number of the device") 148 @OptionalParam(name="lot-number") 149 StringAndListParam theLot_number, 150 151 152 @Description(shortDefinition="The manufacture date of the device") 153 @OptionalParam(name="manufacture-date") 154 DateRangeParam theManufacture_date, 155 156 157 @Description(shortDefinition="The manufacturer of the device") 158 @OptionalParam(name="manufacturer") 159 StringAndListParam theManufacturer, 160 161 162 @Description(shortDefinition="The model of the device") 163 @OptionalParam(name="model") 164 StringAndListParam theModel, 165 166 167 @Description(shortDefinition="The organization responsible for the device") 168 @OptionalParam(name="organization", targetTypes={ } ) 169 ReferenceAndListParam theOrganization, 170 171 172 @Description(shortDefinition="The parent device") 173 @OptionalParam(name="parent", targetTypes={ } ) 174 ReferenceAndListParam theParent, 175 176 177 @Description(shortDefinition="The serial number of the device") 178 @OptionalParam(name="serial-number") 179 StringAndListParam theSerial_number, 180 181 182 @Description(shortDefinition="The standards, specifications, or formal guidances.") 183 @OptionalParam(name="specification") 184 TokenAndListParam theSpecification, 185 186 187 @Description(shortDefinition="A composite of both specification and version") 188 @OptionalParam(name="specification-version", compositeTypes= { TokenParam.class, StringParam.class }) 189 CompositeAndListParam<TokenParam, StringParam> theSpecification_version, 190 191 192 @Description(shortDefinition="active | inactive | entered-in-error | unknown") 193 @OptionalParam(name="status") 194 TokenAndListParam theStatus, 195 196 197 @Description(shortDefinition="The type of the device") 198 @OptionalParam(name="type") 199 TokenAndListParam theType, 200 201 202 @Description(shortDefinition="UDI Barcode (RFID or other technology) string in *HRF* format.") 203 @OptionalParam(name="udi-carrier") 204 StringAndListParam theUdi_carrier, 205 206 207 @Description(shortDefinition="The udi Device Identifier (DI)") 208 @OptionalParam(name="udi-di") 209 StringAndListParam theUdi_di, 210 211 212 @Description(shortDefinition="Network address to contact device") 213 @OptionalParam(name="url") 214 UriAndListParam theUrl, 215 216 217 @Description(shortDefinition="The specific version of the device") 218 @OptionalParam(name="version") 219 StringAndListParam theVersion, 220 221 @RawParam 222 Map<String, List<String>> theAdditionalRawParams, 223 224 225 @IncludeParam 226 Set<Include> theIncludes, 227 228 @IncludeParam(reverse=true) 229 Set<Include> theRevIncludes, 230 231 @Sort 232 SortSpec theSort, 233 234 @ca.uhn.fhir.rest.annotation.Count 235 Integer theCount, 236 237 @ca.uhn.fhir.rest.annotation.Offset 238 Integer theOffset, 239 240 SummaryEnum theSummaryMode, 241 242 SearchTotalModeEnum theSearchTotalMode, 243 244 SearchContainedModeEnum theSearchContainedMode 245 246 ) { 247 startRequest(theServletRequest); 248 try { 249 SearchParameterMap paramMap = new SearchParameterMap(); 250 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 251 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 252 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 253 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 254 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 255 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 256 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 257 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 258 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 259 260 paramMap.add("_has", theHas); 261 paramMap.add("_id", the_id); 262 paramMap.add("_profile", the_profile); 263 paramMap.add("_security", the_security); 264 paramMap.add("_tag", the_tag); 265 paramMap.add("_text", the_text); 266 paramMap.add("biological-source-event", theBiological_source_event); 267 paramMap.add("code", theCode); 268 paramMap.add("code-value-concept", theCode_value_concept); 269 paramMap.add("definition", theDefinition); 270 paramMap.add("device-name", theDevice_name); 271 paramMap.add("expiration-date", theExpiration_date); 272 paramMap.add("identifier", theIdentifier); 273 paramMap.add("location", theLocation); 274 paramMap.add("lot-number", theLot_number); 275 paramMap.add("manufacture-date", theManufacture_date); 276 paramMap.add("manufacturer", theManufacturer); 277 paramMap.add("model", theModel); 278 paramMap.add("organization", theOrganization); 279 paramMap.add("parent", theParent); 280 paramMap.add("serial-number", theSerial_number); 281 paramMap.add("specification", theSpecification); 282 paramMap.add("specification-version", theSpecification_version); 283 paramMap.add("status", theStatus); 284 paramMap.add("type", theType); 285 paramMap.add("udi-carrier", theUdi_carrier); 286 paramMap.add("udi-di", theUdi_di); 287 paramMap.add("url", theUrl); 288 paramMap.add("version", theVersion); 289paramMap.setRevIncludes(theRevIncludes); 290 paramMap.setLastUpdated(the_lastUpdated); 291 paramMap.setIncludes(theIncludes); 292 paramMap.setSort(theSort); 293 paramMap.setCount(theCount); 294 paramMap.setOffset(theOffset); 295 paramMap.setSummaryMode(theSummaryMode); 296 paramMap.setSearchTotalMode(theSearchTotalMode); 297 paramMap.setSearchContainedMode(theSearchContainedMode); 298 299 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 300 301 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 302 return retVal; 303 } finally { 304 endRequest(theServletRequest); 305 } 306 } 307 308}