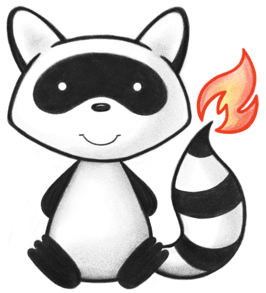
001 002package ca.uhn.fhir.jpa.rp.r5; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r5.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class MedicationRequestResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<MedicationRequest> 021 { 022 023 @Override 024 public Class<MedicationRequest> getResourceType() { 025 return MedicationRequest.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 083 @OptionalParam(name="_lastUpdated") 084 DateRangeParam the_lastUpdated, 085 086 087 @Description(shortDefinition="The profile of the resource") 088 @OptionalParam(name="_profile") 089 UriAndListParam the_profile, 090 091 092 @Description(shortDefinition="The security of the resource") 093 @OptionalParam(name="_security") 094 TokenAndListParam the_security, 095 096 097 @Description(shortDefinition="The tag of the resource") 098 @OptionalParam(name="_tag") 099 TokenAndListParam the_tag, 100 101 102 @Description(shortDefinition="Search on the narrative of the resource") 103 @OptionalParam(name="_text") 104 SpecialAndListParam the_text, 105 106 107 @Description(shortDefinition="Return prescriptions written on this date") 108 @OptionalParam(name="authoredon") 109 DateRangeParam theAuthoredon, 110 111 112 @Description(shortDefinition="Returns prescriptions with different categories") 113 @OptionalParam(name="category") 114 TokenAndListParam theCategory, 115 116 117 @Description(shortDefinition="Multiple Resources: * [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance* [AuditEvent](auditevent.html): More specific code for the event* [Basic](basic.html): Kind of Resource* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code* [Condition](condition.html): Code for the condition* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code* [ImagingSelection](imagingselection.html): The imaging selection status* [List](list.html): What the purpose of this list is* [Medication](medication.html): Returns medications for a specific code* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code* [MedicationStatement](medicationstatement.html): Return statements of this medication code* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake* [Observation](observation.html): The code of the observation type* [Procedure](procedure.html): A code to identify a procedure* [RequestOrchestration](requestorchestration.html): The code of the request orchestration* [Task](task.html): Search by task code") 118 @OptionalParam(name="code") 119 TokenAndListParam theCode, 120 121 122 @Description(shortDefinition="Returns medication request to be administered on a specific date or within a date range") 123 @OptionalParam(name="combo-date") 124 DateRangeParam theCombo_date, 125 126 127 @Description(shortDefinition="Multiple Resources: * [MedicationAdministration](medicationadministration.html): Return administrations that share this encounter* [MedicationRequest](medicationrequest.html): Return prescriptions with this encounter identifier") 128 @OptionalParam(name="encounter", targetTypes={ } ) 129 ReferenceAndListParam theEncounter, 130 131 132 @Description(shortDefinition="Composite request this is part of") 133 @OptionalParam(name="group-identifier") 134 TokenAndListParam theGroup_identifier, 135 136 137 @Description(shortDefinition="Multiple Resources: * [Account](account.html): Account number* [AdverseEvent](adverseevent.html): Business identifier for the event* [AllergyIntolerance](allergyintolerance.html): External ids for this item* [Appointment](appointment.html): An Identifier of the Appointment* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response* [Basic](basic.html): Business identifier* [BodyStructure](bodystructure.html): Bodystructure identifier* [CarePlan](careplan.html): External Ids for this plan* [CareTeam](careteam.html): External Ids for this team* [ChargeItem](chargeitem.html): Business Identifier for item* [Claim](claim.html): The primary identifier of the financial resource* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse* [ClinicalImpression](clinicalimpression.html): Business identifier* [Communication](communication.html): Unique identifier* [CommunicationRequest](communicationrequest.html): Unique identifier* [Composition](composition.html): Version-independent identifier for the Composition* [Condition](condition.html): A unique identifier of the condition record* [Consent](consent.html): Identifier for this record (external references)* [Contract](contract.html): The identity of the contract* [Coverage](coverage.html): The primary identifier of the insured and the coverage* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier* [DetectedIssue](detectedissue.html): Unique id for the detected issue* [DeviceRequest](devicerequest.html): Business identifier for request/order* [DeviceUsage](deviceusage.html): Search by identifier* [DiagnosticReport](diagnosticreport.html): An identifier for the report* [DocumentReference](documentreference.html): Identifier of the attachment binary* [Encounter](encounter.html): Identifier(s) by which this encounter is known* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier* [Flag](flag.html): Business identifier* [Goal](goal.html): External Ids for this goal* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID* [Immunization](immunization.html): Business identifier* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier* [Invoice](invoice.html): Business Identifier for item* [List](list.html): Business identifier* [MeasureReport](measurereport.html): External identifier of the measure report to be returned* [Medication](medication.html): Returns medications with this external identifier* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier* [MedicationStatement](medicationstatement.html): Return statements with this external identifier* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence* [NutritionIntake](nutritionintake.html): Return statements with this external identifier* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier* [Observation](observation.html): The unique id for a particular observation* [Person](person.html): A person Identifier* [Procedure](procedure.html): A unique identifier for a procedure* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study* [RiskAssessment](riskassessment.html): Unique identifier for the assessment* [ServiceRequest](servicerequest.html): Identifiers assigned to this order* [Specimen](specimen.html): The unique identifier associated with the specimen* [SupplyDelivery](supplydelivery.html): External identifier* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest* [Task](task.html): Search for a task instance by its business identifier* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier") 138 @OptionalParam(name="identifier") 139 TokenAndListParam theIdentifier, 140 141 142 @Description(shortDefinition="Returns prescriptions intended to be dispensed by this Organization") 143 @OptionalParam(name="intended-dispenser", targetTypes={ } ) 144 ReferenceAndListParam theIntended_dispenser, 145 146 147 @Description(shortDefinition="Returns the intended performer of the administration of the medication request") 148 @OptionalParam(name="intended-performer", targetTypes={ } ) 149 ReferenceAndListParam theIntended_performer, 150 151 152 @Description(shortDefinition="Returns requests for a specific type of performer") 153 @OptionalParam(name="intended-performertype") 154 TokenAndListParam theIntended_performertype, 155 156 157 @Description(shortDefinition="Returns prescriptions with different intents") 158 @OptionalParam(name="intent") 159 TokenAndListParam theIntent, 160 161 162 @Description(shortDefinition="Multiple Resources: * [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference* [MedicationStatement](medicationstatement.html): Return statements of this medication reference") 163 @OptionalParam(name="medication", targetTypes={ } ) 164 ReferenceAndListParam theMedication, 165 166 167 @Description(shortDefinition="Multiple Resources: * [Account](account.html): The entity that caused the expenses* [AdverseEvent](adverseevent.html): Subject impacted by event* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for* [Appointment](appointment.html): One of the individuals of the appointment is this patient* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient* [AuditEvent](auditevent.html): Where the activity involved patient data* [Basic](basic.html): Identifies the focus of this resource* [BodyStructure](bodystructure.html): Who this is about* [CarePlan](careplan.html): Who the care plan is for* [CareTeam](careteam.html): Who care team is for* [ChargeItem](chargeitem.html): Individual service was done for/to* [Claim](claim.html): Patient receiving the products or services* [ClaimResponse](claimresponse.html): The subject of care* [ClinicalImpression](clinicalimpression.html): Patient assessed* [Communication](communication.html): Focus of message* [CommunicationRequest](communicationrequest.html): Focus of message* [Composition](composition.html): Who and/or what the composition is about* [Condition](condition.html): Who has the condition?* [Consent](consent.html): Who the consent applies to* [Contract](contract.html): The identity of the subject of the contract (if a patient)* [Coverage](coverage.html): Retrieve coverages for a patient* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient* [DetectedIssue](detectedissue.html): Associated patient* [DeviceRequest](devicerequest.html): Individual the service is ordered for* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient* [DocumentReference](documentreference.html): Who/what is the subject of the document* [Encounter](encounter.html): The patient present at the encounter* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for* [Flag](flag.html): The identity of a subject to list flags for* [Goal](goal.html): Who this goal is intended for* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results* [ImagingSelection](imagingselection.html): Who the study is about* [ImagingStudy](imagingstudy.html): Who the study is about* [Immunization](immunization.html): The patient for the vaccination record* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for* [Invoice](invoice.html): Recipient(s) of goods and services* [List](list.html): If all resources have the same subject* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.* [MolecularSequence](molecularsequence.html): The subject that the sequence is about* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement* [Observation](observation.html): The subject that the observation is about (if patient)* [Person](person.html): The Person links to this Patient* [Procedure](procedure.html): Search by subject - a patient* [Provenance](provenance.html): Where the activity involved patient data* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response* [RelatedPerson](relatedperson.html): The patient this related person is related to* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations* [ResearchSubject](researchsubject.html): Who or what is part of study* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?* [ServiceRequest](servicerequest.html): Search by subject - a patient* [Specimen](specimen.html): The patient the specimen comes from* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined* [Task](task.html): Search by patient* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for") 168 @OptionalParam(name="patient", targetTypes={ } ) 169 ReferenceAndListParam thePatient, 170 171 172 @Description(shortDefinition="Returns prescriptions with different priorities") 173 @OptionalParam(name="priority") 174 TokenAndListParam thePriority, 175 176 177 @Description(shortDefinition="Returns prescriptions prescribed by this prescriber") 178 @OptionalParam(name="requester", targetTypes={ } ) 179 ReferenceAndListParam theRequester, 180 181 182 @Description(shortDefinition="Multiple Resources: * [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified)* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status* [MedicationRequest](medicationrequest.html): Status of the prescription* [MedicationStatement](medicationstatement.html): Return statements that match the given status") 183 @OptionalParam(name="status") 184 TokenAndListParam theStatus, 185 186 187 @Description(shortDefinition="The identity of a patient to list orders for") 188 @OptionalParam(name="subject", targetTypes={ } ) 189 ReferenceAndListParam theSubject, 190 191 @RawParam 192 Map<String, List<String>> theAdditionalRawParams, 193 194 195 @IncludeParam 196 Set<Include> theIncludes, 197 198 @IncludeParam(reverse=true) 199 Set<Include> theRevIncludes, 200 201 @Sort 202 SortSpec theSort, 203 204 @ca.uhn.fhir.rest.annotation.Count 205 Integer theCount, 206 207 @ca.uhn.fhir.rest.annotation.Offset 208 Integer theOffset, 209 210 SummaryEnum theSummaryMode, 211 212 SearchTotalModeEnum theSearchTotalMode, 213 214 SearchContainedModeEnum theSearchContainedMode 215 216 ) { 217 startRequest(theServletRequest); 218 try { 219 SearchParameterMap paramMap = new SearchParameterMap(); 220 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 221 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 222 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 223 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 224 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 225 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 226 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 227 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 228 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 229 230 paramMap.add("_has", theHas); 231 paramMap.add("_id", the_id); 232 paramMap.add("_profile", the_profile); 233 paramMap.add("_security", the_security); 234 paramMap.add("_tag", the_tag); 235 paramMap.add("_text", the_text); 236 paramMap.add("authoredon", theAuthoredon); 237 paramMap.add("category", theCategory); 238 paramMap.add("code", theCode); 239 paramMap.add("combo-date", theCombo_date); 240 paramMap.add("encounter", theEncounter); 241 paramMap.add("group-identifier", theGroup_identifier); 242 paramMap.add("identifier", theIdentifier); 243 paramMap.add("intended-dispenser", theIntended_dispenser); 244 paramMap.add("intended-performer", theIntended_performer); 245 paramMap.add("intended-performertype", theIntended_performertype); 246 paramMap.add("intent", theIntent); 247 paramMap.add("medication", theMedication); 248 paramMap.add("patient", thePatient); 249 paramMap.add("priority", thePriority); 250 paramMap.add("requester", theRequester); 251 paramMap.add("status", theStatus); 252 paramMap.add("subject", theSubject); 253paramMap.setRevIncludes(theRevIncludes); 254 paramMap.setLastUpdated(the_lastUpdated); 255 paramMap.setIncludes(theIncludes); 256 paramMap.setSort(theSort); 257 paramMap.setCount(theCount); 258 paramMap.setOffset(theOffset); 259 paramMap.setSummaryMode(theSummaryMode); 260 paramMap.setSearchTotalMode(theSearchTotalMode); 261 paramMap.setSearchContainedMode(theSearchContainedMode); 262 263 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 264 265 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 266 return retVal; 267 } finally { 268 endRequest(theServletRequest); 269 } 270 } 271 272}