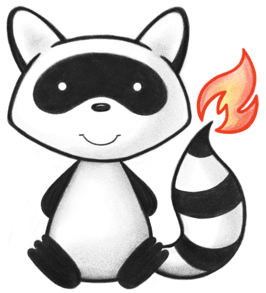
001 002package ca.uhn.fhir.jpa.rp.r5; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r5.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class PractitionerResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<Practitioner> 021 { 022 023 @Override 024 public Class<Practitioner> getResourceType() { 025 return Practitioner.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 083 @OptionalParam(name="_lastUpdated") 084 DateRangeParam the_lastUpdated, 085 086 087 @Description(shortDefinition="The profile of the resource") 088 @OptionalParam(name="_profile") 089 UriAndListParam the_profile, 090 091 092 @Description(shortDefinition="The security of the resource") 093 @OptionalParam(name="_security") 094 TokenAndListParam the_security, 095 096 097 @Description(shortDefinition="The tag of the resource") 098 @OptionalParam(name="_tag") 099 TokenAndListParam the_tag, 100 101 102 @Description(shortDefinition="Search on the narrative of the resource") 103 @OptionalParam(name="_text") 104 SpecialAndListParam the_text, 105 106 107 @Description(shortDefinition="Whether the practitioner record is active") 108 @OptionalParam(name="active") 109 TokenAndListParam theActive, 110 111 112 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text") 113 @OptionalParam(name="address") 114 StringAndListParam theAddress, 115 116 117 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A city specified in an address* [Person](person.html): A city specified in an address* [Practitioner](practitioner.html): A city specified in an address* [RelatedPerson](relatedperson.html): A city specified in an address") 118 @OptionalParam(name="address-city") 119 StringAndListParam theAddress_city, 120 121 122 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A country specified in an address* [Person](person.html): A country specified in an address* [Practitioner](practitioner.html): A country specified in an address* [RelatedPerson](relatedperson.html): A country specified in an address") 123 @OptionalParam(name="address-country") 124 StringAndListParam theAddress_country, 125 126 127 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A postalCode specified in an address* [Person](person.html): A postal code specified in an address* [Practitioner](practitioner.html): A postalCode specified in an address* [RelatedPerson](relatedperson.html): A postal code specified in an address") 128 @OptionalParam(name="address-postalcode") 129 StringAndListParam theAddress_postalcode, 130 131 132 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A state specified in an address* [Person](person.html): A state specified in an address* [Practitioner](practitioner.html): A state specified in an address* [RelatedPerson](relatedperson.html): A state specified in an address") 133 @OptionalParam(name="address-state") 134 StringAndListParam theAddress_state, 135 136 137 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A use code specified in an address* [Person](person.html): A use code specified in an address* [Practitioner](practitioner.html): A use code specified in an address* [RelatedPerson](relatedperson.html): A use code specified in an address") 138 @OptionalParam(name="address-use") 139 TokenAndListParam theAddress_use, 140 141 142 @Description(shortDefinition="A language to communicate with the practitioner") 143 @OptionalParam(name="communication") 144 TokenAndListParam theCommunication, 145 146 147 @Description(shortDefinition="The date of death has been provided and satisfies this search value") 148 @OptionalParam(name="death-date") 149 DateRangeParam theDeath_date, 150 151 152 @Description(shortDefinition="This Practitioner has been marked as deceased, or has a death date entered") 153 @OptionalParam(name="deceased") 154 TokenAndListParam theDeceased, 155 156 157 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A value in an email contact* [Person](person.html): A value in an email contact* [Practitioner](practitioner.html): A value in an email contact* [PractitionerRole](practitionerrole.html): A value in an email contact* [RelatedPerson](relatedperson.html): A value in an email contact") 158 @OptionalParam(name="email") 159 TokenAndListParam theEmail, 160 161 162 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A portion of the family name of the patient* [Practitioner](practitioner.html): A portion of the family name") 163 @OptionalParam(name="family") 164 StringAndListParam theFamily, 165 166 167 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): Gender of the patient* [Person](person.html): The gender of the person* [Practitioner](practitioner.html): Gender of the practitioner* [RelatedPerson](relatedperson.html): Gender of the related person") 168 @OptionalParam(name="gender") 169 TokenAndListParam theGender, 170 171 172 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A portion of the given name of the patient* [Practitioner](practitioner.html): A portion of the given name") 173 @OptionalParam(name="given") 174 StringAndListParam theGiven, 175 176 177 @Description(shortDefinition="A practitioner's Identifier") 178 @OptionalParam(name="identifier") 179 TokenAndListParam theIdentifier, 180 181 182 @Description(shortDefinition="A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text") 183 @OptionalParam(name="name") 184 StringAndListParam theName, 185 186 187 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A value in a phone contact* [Person](person.html): A value in a phone contact* [Practitioner](practitioner.html): A value in a phone contact* [PractitionerRole](practitionerrole.html): A value in a phone contact* [RelatedPerson](relatedperson.html): A value in a phone contact") 188 @OptionalParam(name="phone") 189 TokenAndListParam thePhone, 190 191 192 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm* [Person](person.html): A portion of name using some kind of phonetic matching algorithm* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm") 193 @OptionalParam(name="phonetic") 194 StringAndListParam thePhonetic, 195 196 197 @Description(shortDefinition="The date(s) a qualification is valid for") 198 @OptionalParam(name="qualification-period") 199 DateRangeParam theQualification_period, 200 201 202 @Description(shortDefinition="Multiple Resources: * [Patient](patient.html): The value in any kind of telecom details of the patient* [Person](person.html): The value in any kind of contact* [Practitioner](practitioner.html): The value in any kind of contact* [PractitionerRole](practitionerrole.html): The value in any kind of contact* [RelatedPerson](relatedperson.html): The value in any kind of contact") 203 @OptionalParam(name="telecom") 204 TokenAndListParam theTelecom, 205 206 @RawParam 207 Map<String, List<String>> theAdditionalRawParams, 208 209 210 @IncludeParam 211 Set<Include> theIncludes, 212 213 @IncludeParam(reverse=true) 214 Set<Include> theRevIncludes, 215 216 @Sort 217 SortSpec theSort, 218 219 @ca.uhn.fhir.rest.annotation.Count 220 Integer theCount, 221 222 @ca.uhn.fhir.rest.annotation.Offset 223 Integer theOffset, 224 225 SummaryEnum theSummaryMode, 226 227 SearchTotalModeEnum theSearchTotalMode, 228 229 SearchContainedModeEnum theSearchContainedMode 230 231 ) { 232 startRequest(theServletRequest); 233 try { 234 SearchParameterMap paramMap = new SearchParameterMap(); 235 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 236 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 237 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 238 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 239 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 240 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 241 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 242 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 243 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 244 245 paramMap.add("_has", theHas); 246 paramMap.add("_id", the_id); 247 paramMap.add("_profile", the_profile); 248 paramMap.add("_security", the_security); 249 paramMap.add("_tag", the_tag); 250 paramMap.add("_text", the_text); 251 paramMap.add("active", theActive); 252 paramMap.add("address", theAddress); 253 paramMap.add("address-city", theAddress_city); 254 paramMap.add("address-country", theAddress_country); 255 paramMap.add("address-postalcode", theAddress_postalcode); 256 paramMap.add("address-state", theAddress_state); 257 paramMap.add("address-use", theAddress_use); 258 paramMap.add("communication", theCommunication); 259 paramMap.add("death-date", theDeath_date); 260 paramMap.add("deceased", theDeceased); 261 paramMap.add("email", theEmail); 262 paramMap.add("family", theFamily); 263 paramMap.add("gender", theGender); 264 paramMap.add("given", theGiven); 265 paramMap.add("identifier", theIdentifier); 266 paramMap.add("name", theName); 267 paramMap.add("phone", thePhone); 268 paramMap.add("phonetic", thePhonetic); 269 paramMap.add("qualification-period", theQualification_period); 270 paramMap.add("telecom", theTelecom); 271paramMap.setRevIncludes(theRevIncludes); 272 paramMap.setLastUpdated(the_lastUpdated); 273 paramMap.setIncludes(theIncludes); 274 paramMap.setSort(theSort); 275 paramMap.setCount(theCount); 276 paramMap.setOffset(theOffset); 277 paramMap.setSummaryMode(theSummaryMode); 278 paramMap.setSearchTotalMode(theSearchTotalMode); 279 paramMap.setSearchContainedMode(theSearchContainedMode); 280 281 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 282 283 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 284 return retVal; 285 } finally { 286 endRequest(theServletRequest); 287 } 288 } 289 290}