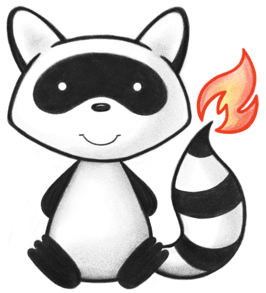
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.search; 021 022import ca.uhn.fhir.jpa.api.dao.DaoRegistry; 023import ca.uhn.fhir.jpa.dao.SearchBuilderFactory; 024import ca.uhn.fhir.jpa.partition.IRequestPartitionHelperSvc; 025import ca.uhn.fhir.rest.api.server.IBundleProvider; 026import ca.uhn.fhir.rest.api.server.RequestDetails; 027import ca.uhn.fhir.rest.server.BasePagingProvider; 028import jakarta.annotation.Nullable; 029import org.springframework.beans.factory.annotation.Autowired; 030 031// Note: this class is not annotated with @Service because we want to 032// explicitly define it in BaseConfig.java. This is done so that 033// implementors can override if they want to. 034public class DatabaseBackedPagingProvider extends BasePagingProvider { 035 036 @Autowired 037 private DaoRegistry myDaoRegistry; 038 039 @Autowired 040 private SearchBuilderFactory mySearchBuilderFactory; 041 042 @Autowired 043 private PersistedJpaBundleProviderFactory myPersistedJpaBundleProviderFactory; 044 045 @Autowired 046 private IRequestPartitionHelperSvc myRequestPartitionHelperSvc; 047 048 /** 049 * Constructor 050 */ 051 public DatabaseBackedPagingProvider() { 052 super(); 053 } 054 055 /** 056 * Constructor 057 * 058 * @deprecated Use {@link DatabaseBackedPagingProvider} as this constructor has no purpose 059 */ 060 @Deprecated 061 public DatabaseBackedPagingProvider(int theSize) { 062 this(); 063 } 064 065 @Override 066 public synchronized IBundleProvider retrieveResultList(RequestDetails theRequestDetails, String theId) { 067 PersistedJpaBundleProvider provider = myPersistedJpaBundleProviderFactory.newInstance(theRequestDetails, theId); 068 return validateAndReturnBundleProvider(provider); 069 } 070 071 /** 072 * Subclasses may override in order to modify the bundle provider being returned 073 */ 074 @Nullable 075 protected PersistedJpaBundleProvider validateAndReturnBundleProvider(PersistedJpaBundleProvider theBundleProvider) { 076 if (!theBundleProvider.ensureSearchEntityLoaded()) { 077 return null; 078 } 079 return theBundleProvider; 080 } 081 082 @Override 083 public synchronized String storeResultList(RequestDetails theRequestDetails, IBundleProvider theList) { 084 String uuid = theList.getUuid(); 085 return uuid; 086 } 087}