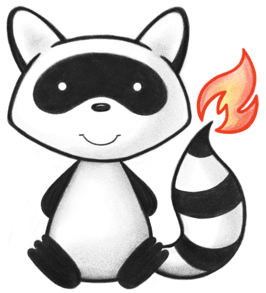
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.search; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import ca.uhn.fhir.jpa.config.JpaConfig; 024import ca.uhn.fhir.jpa.dao.ISearchBuilder; 025import ca.uhn.fhir.jpa.entity.Search; 026import ca.uhn.fhir.jpa.entity.SearchTypeEnum; 027import ca.uhn.fhir.jpa.model.dao.JpaPid; 028import ca.uhn.fhir.jpa.model.search.SearchStatusEnum; 029import ca.uhn.fhir.jpa.search.builder.tasks.SearchTask; 030import ca.uhn.fhir.rest.api.server.IBundleProvider; 031import ca.uhn.fhir.rest.api.server.RequestDetails; 032import ca.uhn.fhir.rest.param.HistorySearchStyleEnum; 033import jakarta.annotation.Nullable; 034import org.springframework.beans.factory.annotation.Autowired; 035import org.springframework.context.ApplicationContext; 036 037import java.util.Date; 038import java.util.UUID; 039 040import static org.apache.commons.lang3.StringUtils.defaultIfBlank; 041 042public class PersistedJpaBundleProviderFactory { 043 044 @Autowired 045 private ApplicationContext myApplicationContext; 046 047 public PersistedJpaBundleProvider newInstance(RequestDetails theRequest, String theUuid) { 048 Object retVal = myApplicationContext.getBean(JpaConfig.PERSISTED_JPA_BUNDLE_PROVIDER, theRequest, theUuid); 049 return (PersistedJpaBundleProvider) retVal; 050 } 051 052 public PersistedJpaBundleProvider newInstance(RequestDetails theRequest, Search theSearch) { 053 Object retVal = 054 myApplicationContext.getBean(JpaConfig.PERSISTED_JPA_BUNDLE_PROVIDER_BY_SEARCH, theRequest, theSearch); 055 return (PersistedJpaBundleProvider) retVal; 056 } 057 058 public PersistedJpaSearchFirstPageBundleProvider newInstanceFirstPage( 059 RequestDetails theRequestDetails, 060 SearchTask theTask, 061 ISearchBuilder<JpaPid> theSearchBuilder, 062 RequestPartitionId theRequestPartitionId) { 063 return (PersistedJpaSearchFirstPageBundleProvider) myApplicationContext.getBean( 064 JpaConfig.PERSISTED_JPA_SEARCH_FIRST_PAGE_BUNDLE_PROVIDER, 065 theRequestDetails, 066 theTask, 067 theSearchBuilder, 068 theRequestPartitionId); 069 } 070 071 public IBundleProvider history( 072 RequestDetails theRequest, 073 String theResourceType, 074 @Nullable JpaPid theResourcePid, 075 Date theRangeStartInclusive, 076 Date theRangeEndInclusive, 077 Integer theOffset, 078 RequestPartitionId theRequestPartitionId) { 079 return history( 080 theRequest, 081 theResourceType, 082 theResourcePid, 083 theRangeStartInclusive, 084 theRangeEndInclusive, 085 theOffset, 086 null, 087 theRequestPartitionId); 088 } 089 090 public IBundleProvider history( 091 RequestDetails theRequest, 092 String theResourceType, 093 @Nullable JpaPid theResourcePid, 094 Date theRangeStartInclusive, 095 Date theRangeEndInclusive, 096 Integer theOffset, 097 HistorySearchStyleEnum searchParameterType, 098 RequestPartitionId theRequestPartitionId) { 099 String resourceName = defaultIfBlank(theResourceType, null); 100 101 Search search = new Search(); 102 search.setOffset(theOffset); 103 search.setDeleted(false); 104 search.setCreated(new Date()); 105 search.setLastUpdated(theRangeStartInclusive, theRangeEndInclusive); 106 search.setUuid(UUID.randomUUID().toString()); 107 search.setResourceType(resourceName); 108 search.setResourceId(theResourcePid); 109 search.setSearchType(SearchTypeEnum.HISTORY); 110 search.setStatus(SearchStatusEnum.FINISHED); 111 search.setHistorySearchStyle(searchParameterType); 112 113 PersistedJpaBundleProvider provider = newInstance(theRequest, search); 114 provider.setRequestPartitionId(theRequestPartitionId); 115 116 return provider; 117 } 118}