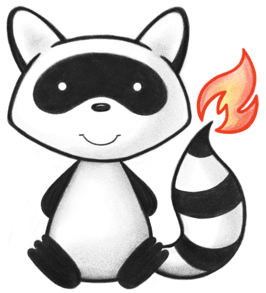
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.search.autocomplete; 021 022import com.google.gson.JsonObject; 023import jakarta.annotation.Nonnull; 024import org.apache.commons.lang3.Validate; 025 026public class RawElasticJsonBuilder { 027 @Nonnull 028 static JsonObject makeMatchBoolPrefixPredicate(String theFieldName, String queryText) { 029 030 JsonObject matchBoolBody = new JsonObject(); 031 matchBoolBody.addProperty(theFieldName, queryText); 032 033 JsonObject predicate = new JsonObject(); 034 predicate.add("match_bool_prefix", matchBoolBody); 035 return predicate; 036 } 037 038 public static JsonObject makeWildcardPredicate(String theFieldName, String theQueryText) { 039 Validate.notEmpty(theQueryText); 040 041 JsonObject params = new JsonObject(); 042 params.addProperty("value", theQueryText); 043 044 JsonObject wildcardBody = new JsonObject(); 045 wildcardBody.add(theFieldName, params); 046 047 JsonObject predicate = new JsonObject(); 048 predicate.add("wildcard", wildcardBody); 049 return predicate; 050 } 051 052 @Nonnull 053 public static JsonObject makeMatchAllPredicate() { 054 JsonObject o = new JsonObject(); 055 o.add("match_all", new JsonObject()); 056 return o; 057 } 058}