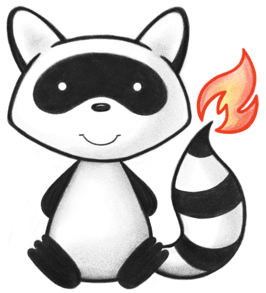
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.search.autocomplete; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 024import ca.uhn.fhir.rest.param.TokenParamModifier; 025import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027import org.hl7.fhir.instance.model.api.IIdType; 028import org.hl7.fhir.instance.model.api.IPrimitiveType; 029 030import java.util.Arrays; 031import java.util.List; 032import java.util.Optional; 033 034import static org.apache.commons.lang3.StringUtils.defaultString; 035import static org.apache.commons.lang3.StringUtils.isNotBlank; 036 037public class ValueSetAutocompleteOptions { 038 039 private final String myResourceType; 040 private final String mySearchParamCode; 041 private final String mySearchParamModifier; 042 private final String myFilter; 043 private final Integer myCount; 044 045 static final List<String> ourSupportedModifiers = Arrays.asList("", TokenParamModifier.TEXT.getBareModifier()); 046 047 public ValueSetAutocompleteOptions(String theContext, String theFilter, Integer theCount) { 048 myFilter = theFilter; 049 myCount = theCount; 050 int separatorIdx = theContext.indexOf('.'); 051 String codeWithPossibleModifier; 052 if (separatorIdx >= 0) { 053 myResourceType = theContext.substring(0, separatorIdx); 054 codeWithPossibleModifier = theContext.substring(separatorIdx + 1); 055 } else { 056 myResourceType = null; 057 codeWithPossibleModifier = theContext; 058 } 059 int modifierIdx = codeWithPossibleModifier.indexOf(':'); 060 if (modifierIdx >= 0) { 061 mySearchParamCode = codeWithPossibleModifier.substring(0, modifierIdx); 062 mySearchParamModifier = codeWithPossibleModifier.substring(modifierIdx + 1); 063 } else { 064 mySearchParamCode = codeWithPossibleModifier; 065 mySearchParamModifier = null; 066 } 067 } 068 069 public static ValueSetAutocompleteOptions validateAndParseOptions( 070 JpaStorageSettings theStorageSettings, 071 IPrimitiveType<String> theContext, 072 IPrimitiveType<String> theFilter, 073 IPrimitiveType<Integer> theCount, 074 IIdType theId, 075 IPrimitiveType<String> theUrl, 076 IBaseResource theValueSet) { 077 boolean haveId = theId != null && theId.hasIdPart(); 078 boolean haveIdentifier = theUrl != null && isNotBlank(theUrl.getValue()); 079 boolean haveValueSet = theValueSet != null && !theValueSet.isEmpty(); 080 if (haveId || haveIdentifier || haveValueSet) { 081 throw new InvalidRequestException( 082 Msg.code(2020) 083 + "$expand with contexDirection='existing' is only supported at the type leve. It is not supported at instance level, with a url specified, or with a ValueSet ."); 084 } 085 if (!theStorageSettings.isAdvancedHSearchIndexing()) { 086 throw new InvalidRequestException( 087 Msg.code(2022) + "$expand with contexDirection='existing' requires Extended Lucene Indexing."); 088 } 089 if (theContext == null || theContext.isEmpty()) { 090 throw new InvalidRequestException( 091 Msg.code(2021) + "$expand with contexDirection='existing' requires a context"); 092 } 093 String filter = theFilter == null ? null : theFilter.getValue(); 094 ValueSetAutocompleteOptions result = 095 new ValueSetAutocompleteOptions(theContext.getValue(), filter, IPrimitiveType.toValueOrNull(theCount)); 096 097 if (!ourSupportedModifiers.contains(defaultString(result.getSearchParamModifier()))) { 098 throw new InvalidRequestException(Msg.code(2069) 099 + "$expand with contexDirection='existing' only supports plain token search, or the :text modifier. Received " 100 + result.getSearchParamModifier()); 101 } 102 103 return result; 104 } 105 106 public String getResourceType() { 107 return myResourceType; 108 } 109 110 public String getSearchParamCode() { 111 return mySearchParamCode; 112 } 113 114 public String getSearchParamModifier() { 115 return mySearchParamModifier; 116 } 117 118 public String getFilter() { 119 return myFilter; 120 } 121 122 public Optional<Integer> getCount() { 123 return Optional.ofNullable(myCount); 124 } 125}