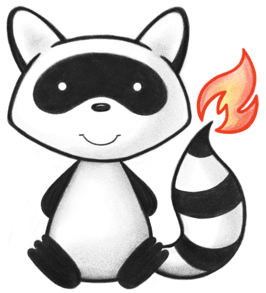
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.search.builder.models; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.interceptor.model.RequestPartitionId; 024import ca.uhn.fhir.jpa.search.builder.sql.SearchQueryBuilder; 025import ca.uhn.fhir.model.api.IQueryParameterType; 026import ca.uhn.fhir.rest.api.RestSearchParameterTypeEnum; 027import com.healthmarketscience.sqlbuilder.dbspec.basic.DbColumn; 028 029import java.security.InvalidParameterException; 030import java.util.List; 031 032public class MissingParameterQueryParams { 033 /** 034 * The sql builder 035 */ 036 private final SearchQueryBuilder mySqlBuilder; 037 038 /** 039 * The parameter type 040 */ 041 private final RestSearchParameterTypeEnum myParamType; 042 043 /** 044 * The list of query parameter types (only needed for validation) 045 */ 046 private final List<? extends IQueryParameterType> myQueryParameterTypes; 047 048 /** 049 * The missing boolean value from :missing=true/false 050 */ 051 private final boolean myIsMissing; 052 053 /** 054 * The name of the parameter. 055 */ 056 private final String myParamName; 057 058 /** 059 * The resource type 060 */ 061 private final String myResourceType; 062 063 /** 064 * The column on which to join. 065 */ 066 private final DbColumn[] mySourceJoinColumn; 067 068 /** 069 * The partition id 070 */ 071 private final RequestPartitionId myRequestPartitionId; 072 073 public MissingParameterQueryParams( 074 SearchQueryBuilder theSqlBuilder, 075 RestSearchParameterTypeEnum theParamType, 076 List<? extends IQueryParameterType> theList, 077 String theParamName, 078 String theResourceType, 079 DbColumn[] theSourceJoinColumn, 080 RequestPartitionId theRequestPartitionId) { 081 mySqlBuilder = theSqlBuilder; 082 myParamType = theParamType; 083 myQueryParameterTypes = theList; 084 if (theList.isEmpty()) { 085 // this will never happen 086 throw new InvalidParameterException(Msg.code(2140) + " Invalid search parameter list. Cannot be empty!"); 087 } 088 myIsMissing = theList.get(0).getMissing(); 089 myParamName = theParamName; 090 myResourceType = theResourceType; 091 mySourceJoinColumn = theSourceJoinColumn; 092 myRequestPartitionId = theRequestPartitionId; 093 } 094 095 public SearchQueryBuilder getSqlBuilder() { 096 return mySqlBuilder; 097 } 098 099 public RestSearchParameterTypeEnum getParamType() { 100 return myParamType; 101 } 102 103 public List<? extends IQueryParameterType> getQueryParameterTypes() { 104 return myQueryParameterTypes; 105 } 106 107 public boolean isMissing() { 108 return myIsMissing; 109 } 110 111 public String getParamName() { 112 return myParamName; 113 } 114 115 public String getResourceType() { 116 return myResourceType; 117 } 118 119 public DbColumn[] getSourceJoinColumn() { 120 return mySourceJoinColumn; 121 } 122 123 public ca.uhn.fhir.interceptor.model.RequestPartitionId getRequestPartitionId() { 124 return myRequestPartitionId; 125 } 126}