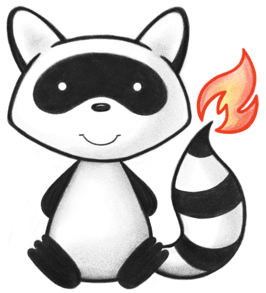
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.search.builder.sql; 021 022import ca.uhn.fhir.jpa.model.dao.JpaPid; 023import com.healthmarketscience.common.util.AppendableExt; 024import com.healthmarketscience.sqlbuilder.Expression; 025import com.healthmarketscience.sqlbuilder.ValidationContext; 026 027import java.io.IOException; 028import java.util.ArrayList; 029import java.util.Arrays; 030import java.util.Collection; 031import java.util.Iterator; 032import java.util.List; 033 034/** 035 * Outputs an SQL tuple for a collection of JpaPids, consisting of 036 * ((resId,partitionId),(resId,partitionId),(resId,partitionId),...) 037 */ 038public class JpaPidValueTuples extends Expression { 039 040 private final Collection<String> myValues; 041 042 public JpaPidValueTuples(Collection<String> theValues) { 043 myValues = theValues; 044 } 045 046 @Override 047 protected void collectSchemaObjects(ValidationContext vContext) { 048 // nothing 049 } 050 051 @Override 052 public void appendTo(AppendableExt app) throws IOException { 053 app.append('('); 054 055 String value; 056 for (Iterator<String> iter = myValues.iterator(); iter.hasNext(); ) { 057 if (hasParens()) { 058 app.append("('"); 059 } 060 value = iter.next(); 061 app.append(value); 062 app.append("','"); 063 value = iter.next(); 064 app.append(value); 065 app.append("')"); 066 if (iter.hasNext()) { 067 app.append(','); 068 } 069 } 070 if (hasParens()) { 071 app.append(')'); 072 } 073 } 074 075 public static JpaPidValueTuples from(SearchQueryBuilder theSearchQueryBuilder, JpaPid[] thePids) { 076 return from(theSearchQueryBuilder, Arrays.asList(thePids)); 077 } 078 079 public static JpaPidValueTuples from(SearchQueryBuilder theSearchQueryBuilder, Collection<JpaPid> thePids) { 080 List<String> placeholders = new ArrayList<>(thePids.size() * 2); 081 for (JpaPid next : thePids) { 082 placeholders.add(theSearchQueryBuilder.generatePlaceholder(next.getPartitionId())); 083 placeholders.add(theSearchQueryBuilder.generatePlaceholder(next.getId())); 084 } 085 return new JpaPidValueTuples(placeholders); 086 } 087}