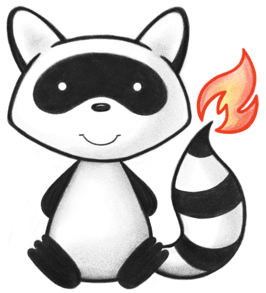
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.search.cache; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import ca.uhn.fhir.jpa.entity.Search; 024import ca.uhn.fhir.jpa.model.dao.JpaPid; 025import ca.uhn.fhir.rest.api.server.RequestDetails; 026import jakarta.annotation.Nullable; 027 028import java.util.List; 029 030public interface ISearchResultCacheSvc { 031 /** 032 * @param theSearch The search - This method is not required to persist any chances to the Search object, it is only provided here for identification 033 * @param thePreviouslyStoredResourcePids A list of resource PIDs that have previously been saved to this search 034 * @param theNewResourcePids A list of new resource PIDs to add to this search (these ones have not been previously saved) 035 */ 036 void storeResults( 037 Search theSearch, 038 List<JpaPid> thePreviouslyStoredResourcePids, 039 List<JpaPid> theNewResourcePids, 040 RequestDetails theRequestDetails, 041 RequestPartitionId theRequestPartitionId); 042 043 /** 044 * Fetch a subset of the search result IDs from the cache 045 * 046 * @param theSearch The search to fetch IDs for 047 * @param theFrom The starting index (inclusive) 048 * @param theTo The ending index (exclusive) 049 * @return A list of resource PIDs, or <code>null</code> if the results no longer exist (this should only happen if the results 050 * have been removed from the cache for some reason, such as expiry or manual purge) 051 */ 052 @Nullable 053 List<JpaPid> fetchResultPids( 054 Search theSearch, 055 int theFrom, 056 int theTo, 057 RequestDetails theRequestDetails, 058 RequestPartitionId theRequestPartitionId); 059 060 /** 061 * Fetch all result PIDs for a given search with no particular order required 062 * 063 * @param theSearch The search object 064 * @return A list of resource PIDs, or <code>null</code> if the results no longer exist (this should only happen if the results 065 * have been removed from the cache for some reason, such as expiry or manual purge) 066 */ 067 @Nullable 068 List<JpaPid> fetchAllResultPids( 069 Search theSearch, RequestDetails theRequestDetails, RequestPartitionId theRequestPartitionId); 070}