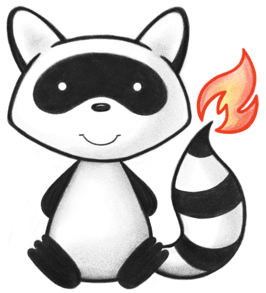
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.search.lastn.json; 021 022import com.fasterxml.jackson.annotation.JsonAutoDetect; 023import com.fasterxml.jackson.annotation.JsonInclude; 024import com.fasterxml.jackson.annotation.JsonProperty; 025 026import java.util.ArrayList; 027import java.util.Date; 028import java.util.List; 029 030@JsonInclude(JsonInclude.Include.NON_NULL) 031@JsonAutoDetect( 032 creatorVisibility = JsonAutoDetect.Visibility.NONE, 033 fieldVisibility = JsonAutoDetect.Visibility.NONE, 034 getterVisibility = JsonAutoDetect.Visibility.NONE, 035 isGetterVisibility = JsonAutoDetect.Visibility.NONE, 036 setterVisibility = JsonAutoDetect.Visibility.NONE) 037public class ObservationJson { 038 039 @JsonProperty(value = "identifier", required = true) 040 private String myIdentifier; 041 042 @JsonProperty(value = "subject", required = true) 043 private String mySubject; 044 045 @JsonProperty(value = "categoryconcepttext", required = false) 046 private List<String> myCategory_concept_text = new ArrayList<>(); 047 048 @JsonProperty(value = "categoryconceptcodingcode", required = false) 049 private List<List<String>> myCategory_coding_code = new ArrayList<>(); 050 051 @JsonProperty(value = "categoryconceptcodingcode_system_hash", required = false) 052 private List<List<String>> myCategory_coding_code_system_hash = new ArrayList<>(); 053 054 @JsonProperty(value = "categoryconceptcodingdisplay", required = false) 055 private List<List<String>> myCategory_coding_display = new ArrayList<>(); 056 057 @JsonProperty(value = "categoryconceptcodingsystem", required = false) 058 private List<List<String>> myCategory_coding_system = new ArrayList<>(); 059 060 @JsonProperty(value = "codeconceptid", required = false) 061 private String myCode_concept_id; 062 063 @JsonProperty(value = "codeconcepttext", required = false) 064 private String myCode_concept_text; 065 066 @JsonProperty(value = "codeconceptcodingcode", required = false) 067 private String myCode_coding_code; 068 069 @JsonProperty(value = "codeconceptcodingcode_system_hash", required = false) 070 private String myCode_coding_code_system_hash; 071 072 @JsonProperty(value = "codeconceptcodingdisplay", required = false) 073 private String myCode_coding_display; 074 075 @JsonProperty(value = "codeconceptcodingsystem", required = false) 076 private String myCode_coding_system; 077 078 @JsonProperty(value = "effectivedtm", required = true) 079 private Date myEffectiveDtm; 080 081 @JsonProperty(value = "resource") 082 private String myResource; 083 084 public ObservationJson() {} 085 086 public void setIdentifier(String theIdentifier) { 087 myIdentifier = theIdentifier; 088 } 089 090 public void setSubject(String theSubject) { 091 mySubject = theSubject; 092 } 093 094 public void setCategories(List<CodeJson> theCategories) { 095 for (CodeJson theConcept : theCategories) { 096 myCategory_concept_text.add(theConcept.getCodeableConceptText()); 097 List<String> coding_code_system_hashes = new ArrayList<>(); 098 List<String> coding_codes = new ArrayList<>(); 099 List<String> coding_displays = new ArrayList<>(); 100 List<String> coding_systems = new ArrayList<>(); 101 for (String theCategoryCoding_code : theConcept.getCoding_code()) { 102 coding_codes.add(theCategoryCoding_code); 103 } 104 for (String theCategoryCoding_system : theConcept.getCoding_system()) { 105 coding_systems.add(theCategoryCoding_system); 106 } 107 for (String theCategoryCoding_code_system_hash : theConcept.getCoding_code_system_hash()) { 108 coding_code_system_hashes.add(theCategoryCoding_code_system_hash); 109 } 110 for (String theCategoryCoding_display : theConcept.getCoding_display()) { 111 coding_displays.add(theCategoryCoding_display); 112 } 113 myCategory_coding_code_system_hash.add(coding_code_system_hashes); 114 myCategory_coding_code.add(coding_codes); 115 myCategory_coding_display.add(coding_displays); 116 myCategory_coding_system.add(coding_systems); 117 } 118 } 119 120 public List<String> getCategory_concept_text() { 121 return myCategory_concept_text; 122 } 123 124 public List<List<String>> getCategory_coding_code_system_hash() { 125 return myCategory_coding_code_system_hash; 126 } 127 128 public List<List<String>> getCategory_coding_code() { 129 return myCategory_coding_code; 130 } 131 132 public List<List<String>> getCategory_coding_display() { 133 return myCategory_coding_display; 134 } 135 136 public List<List<String>> getCategory_coding_system() { 137 return myCategory_coding_system; 138 } 139 140 public void setCode(CodeJson theCode) { 141 myCode_concept_id = theCode.getCodeableConceptId(); 142 myCode_concept_text = theCode.getCodeableConceptText(); 143 // Currently can only support one Coding for Observation Code 144 myCode_coding_code_system_hash = theCode.getCoding_code_system_hash().get(0); 145 myCode_coding_code = theCode.getCoding_code().get(0); 146 myCode_coding_display = theCode.getCoding_display().get(0); 147 myCode_coding_system = theCode.getCoding_system().get(0); 148 } 149 150 public CodeJson getCode() { 151 CodeJson code = new CodeJson(); 152 code.setCodeableConceptId(myCode_concept_id); 153 code.setCodeableConceptText(myCode_concept_text); 154 code.getCoding_code_system_hash().add(myCode_coding_code_system_hash); 155 code.getCoding_code().add(myCode_coding_code); 156 code.getCoding_display().add(myCode_coding_display); 157 code.getCoding_system().add(myCode_coding_system); 158 return code; 159 } 160 161 public String getCode_concept_text() { 162 return myCode_concept_text; 163 } 164 165 public String getCode_coding_code_system_hash() { 166 return myCode_coding_code_system_hash; 167 } 168 169 public String getCode_coding_code() { 170 return myCode_coding_code; 171 } 172 173 public String getCode_coding_display() { 174 return myCode_coding_display; 175 } 176 177 public String getCode_coding_system() { 178 return myCode_coding_system; 179 } 180 181 public String getCode_concept_id() { 182 return myCode_concept_id; 183 } 184 185 public void setEffectiveDtm(Date theEffectiveDtm) { 186 myEffectiveDtm = theEffectiveDtm; 187 } 188 189 public Date getEffectiveDtm() { 190 return myEffectiveDtm; 191 } 192 193 public String getSubject() { 194 return mySubject; 195 } 196 197 public String getIdentifier() { 198 return myIdentifier; 199 } 200 201 public String getResource() { 202 return myResource; 203 } 204 205 public void setResource(String theResource) { 206 myResource = theResource; 207 } 208}