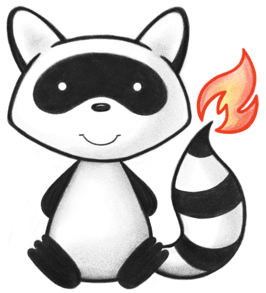
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.search.reindex; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.RuntimeResourceDefinition; 024import ca.uhn.fhir.i18n.Msg; 025import ca.uhn.fhir.jpa.api.dao.DaoRegistry; 026import ca.uhn.fhir.jpa.api.dao.IFhirResourceDao; 027import ca.uhn.fhir.jpa.dao.IFulltextSearchSvc; 028import ca.uhn.fhir.jpa.dao.data.IResourceHistoryTableDao; 029import ca.uhn.fhir.jpa.dao.data.IResourceTableDao; 030import ca.uhn.fhir.jpa.model.entity.ResourceTable; 031import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 032import org.hl7.fhir.instance.model.api.IBaseResource; 033import org.slf4j.Logger; 034import org.slf4j.LoggerFactory; 035import org.springframework.beans.factory.annotation.Autowired; 036import org.springframework.stereotype.Service; 037 038/** 039 * @deprecated 040 */ 041@Service 042public class ResourceReindexer { 043 private static final Logger ourLog = LoggerFactory.getLogger(ResourceReindexer.class); 044 045 @Autowired 046 private IResourceHistoryTableDao myResourceHistoryTableDao; 047 048 @Autowired 049 private IResourceTableDao myResourceTableDao; 050 051 @Autowired 052 private DaoRegistry myDaoRegistry; 053 054 @Autowired(required = false) 055 private IFulltextSearchSvc myFulltextSearchSvc; 056 057 private final FhirContext myFhirContext; 058 059 public ResourceReindexer(FhirContext theFhirContext) { 060 myFhirContext = theFhirContext; 061 } 062 063 public void reindexResourceEntity(ResourceTable theResourceTable) { 064 IFhirResourceDao<?> dao = myDaoRegistry.getResourceDao(theResourceTable.getResourceType()); 065 long expectedVersion = theResourceTable.getVersion(); 066 IBaseResource resource = dao.readByPid(theResourceTable.getPersistentId(), true); 067 068 if (resource == null) { 069 throw new InternalErrorException(Msg.code(1171) + "Could not find resource version " 070 + theResourceTable.getIdDt().toUnqualified().getValue() + " in database"); 071 } 072 073 Long actualVersion = resource.getIdElement().getVersionIdPartAsLong(); 074 if (actualVersion < expectedVersion) { 075 ourLog.warn( 076 "Resource {} version {} does not exist, renumbering version {}", 077 resource.getIdElement().toUnqualifiedVersionless().getValue(), 078 resource.getIdElement().getVersionIdPart(), 079 expectedVersion); 080 myResourceHistoryTableDao.updateVersion(theResourceTable.getId().toFk(), actualVersion, expectedVersion); 081 } 082 083 doReindex(theResourceTable, resource); 084 } 085 086 @SuppressWarnings("unchecked") 087 <T extends IBaseResource> void doReindex(ResourceTable theResourceTable, T theResource) { 088 RuntimeResourceDefinition resourceDefinition = myFhirContext.getResourceDefinition(theResource.getClass()); 089 Class<T> resourceClass = (Class<T>) resourceDefinition.getImplementingClass(); 090 final IFhirResourceDao<T> dao = myDaoRegistry.getResourceDao(resourceClass); 091 dao.reindex(theResource, theResourceTable); 092 if (myFulltextSearchSvc != null && !myFulltextSearchSvc.isDisabled()) { 093 // update the full-text index, if active. 094 myFulltextSearchSvc.reindex(theResourceTable); 095 } 096 } 097}