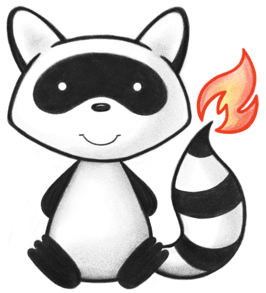
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term; 021 022import ca.uhn.fhir.jpa.entity.TermConceptDesignation; 023import jakarta.annotation.Nullable; 024 025import java.util.Collection; 026 027public interface IValueSetConceptAccumulator { 028 029 void addMessage(String theMessage); 030 031 void includeConcept( 032 String theSystem, 033 String theCode, 034 String theDisplay, 035 Long theSourceConceptPid, 036 String theSourceConceptDirectParentPids, 037 @Nullable String theSystemVersion); 038 039 void includeConceptWithDesignations( 040 String theSystem, 041 String theCode, 042 String theDisplay, 043 @Nullable Collection<TermConceptDesignation> theDesignations, 044 Long theSourceConceptPid, 045 String theSourceConceptDirectParentPids, 046 @Nullable String theSystemVersion); 047 048 /** 049 * @return Returns <code>true</code> if the code was actually present and was removed 050 */ 051 boolean excludeConcept(String theSystem, String theCode); 052 053 @Nullable 054 default Integer getCapacityRemaining() { 055 return null; 056 } 057 058 @Nullable 059 default Integer getSkipCountRemaining() { 060 return null; 061 } 062 063 default boolean isTrackingHierarchy() { 064 return true; 065 } 066 067 @Nullable 068 default void consumeSkipCount(int theSkipCountToConsume) { 069 // nothing 070 } 071 072 /** 073 * Add or subtract from the total concept count (this is not necessarily the same thing as the number of concepts in 074 * the accumulator, since the <code>offset</code> and <code>count</code> parameters applied to the expansion can cause 075 * concepts to not actually be added. 076 * 077 * @param theAdd If <code>true</code>, increment. If <code>false</code>, decrement. 078 * @param theDelta The number of codes to add or subtract 079 */ 080 default void incrementOrDecrementTotalConcepts(boolean theAdd, int theDelta) { 081 // nothing 082 } 083}