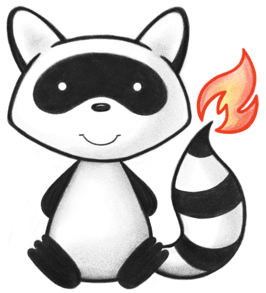
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.jpa.api.dao.IFhirResourceDao; 024import ca.uhn.fhir.jpa.term.api.ITermVersionAdapterSvc; 025import ca.uhn.fhir.rest.api.server.RequestDetails; 026import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 027import ca.uhn.fhir.util.UrlUtil; 028import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_30_40; 029import org.hl7.fhir.convertors.factory.VersionConvertorFactory_30_40; 030import org.hl7.fhir.dstu3.model.CodeSystem; 031import org.hl7.fhir.dstu3.model.ConceptMap; 032import org.hl7.fhir.dstu3.model.ValueSet; 033import org.hl7.fhir.exceptions.FHIRException; 034import org.hl7.fhir.instance.model.api.IIdType; 035import org.springframework.beans.factory.annotation.Autowired; 036import org.springframework.context.ApplicationContext; 037import org.springframework.context.event.ContextRefreshedEvent; 038import org.springframework.context.event.EventListener; 039 040import static org.apache.commons.lang3.StringUtils.isBlank; 041 042public class TermVersionAdapterSvcDstu3 extends BaseTermVersionAdapterSvcImpl implements ITermVersionAdapterSvc { 043 044 private IFhirResourceDao<ConceptMap> myConceptMapResourceDao; 045 private IFhirResourceDao<CodeSystem> myCodeSystemResourceDao; 046 private IFhirResourceDao<ValueSet> myValueSetResourceDao; 047 048 @Autowired 049 private ApplicationContext myAppCtx; 050 051 public TermVersionAdapterSvcDstu3() { 052 super(); 053 } 054 055 /** 056 * Initialize the beans that are used by this service. 057 * <p> 058 * Note: There is a circular dependency here where the CodeSystem DAO 059 * needs terminology services, and the term services need the CodeSystem DAO. 060 * So we look these up in a refresh event instead of just autowiring them 061 * in order to avoid weird circular reference errors. 062 */ 063 @SuppressWarnings({"unchecked", "unused"}) 064 @EventListener 065 public void start(ContextRefreshedEvent theEvent) { 066 myCodeSystemResourceDao = (IFhirResourceDao<CodeSystem>) myAppCtx.getBean("myCodeSystemDaoDstu3"); 067 myValueSetResourceDao = (IFhirResourceDao<ValueSet>) myAppCtx.getBean("myValueSetDaoDstu3"); 068 myConceptMapResourceDao = (IFhirResourceDao<ConceptMap>) myAppCtx.getBean("myConceptMapDaoDstu3"); 069 } 070 071 @Override 072 public IIdType createOrUpdateCodeSystem( 073 org.hl7.fhir.r4.model.CodeSystem theCodeSystemResource, RequestDetails theRequestDetails) { 074 CodeSystem resourceToStore; 075 try { 076 resourceToStore = (CodeSystem) 077 VersionConvertorFactory_30_40.convertResource(theCodeSystemResource, new BaseAdvisor_30_40(false)); 078 } catch (FHIRException e) { 079 throw new InternalErrorException(Msg.code(879) + e); 080 } 081 validateCodeSystemForStorage(theCodeSystemResource); 082 if (isBlank(resourceToStore.getIdElement().getIdPart())) { 083 String matchUrl = "CodeSystem?url=" + UrlUtil.escapeUrlParam(theCodeSystemResource.getUrl()); 084 return myCodeSystemResourceDao 085 .update(resourceToStore, matchUrl, theRequestDetails) 086 .getId(); 087 } else { 088 return myCodeSystemResourceDao 089 .update(resourceToStore, theRequestDetails) 090 .getId(); 091 } 092 } 093 094 @Override 095 public void createOrUpdateConceptMap(org.hl7.fhir.r4.model.ConceptMap theConceptMap) { 096 ConceptMap resourceToStore; 097 try { 098 resourceToStore = (ConceptMap) 099 VersionConvertorFactory_30_40.convertResource(theConceptMap, new BaseAdvisor_30_40(false)); 100 } catch (FHIRException e) { 101 throw new InternalErrorException(Msg.code(880) + e); 102 } 103 if (isBlank(resourceToStore.getIdElement().getIdPart())) { 104 String matchUrl = "ConceptMap?url=" + UrlUtil.escapeUrlParam(theConceptMap.getUrl()); 105 myConceptMapResourceDao.update(resourceToStore, matchUrl); 106 } else { 107 myConceptMapResourceDao.update(resourceToStore); 108 } 109 } 110 111 @Override 112 public void createOrUpdateValueSet(org.hl7.fhir.r4.model.ValueSet theValueSet) { 113 ValueSet valueSetDstu3; 114 try { 115 valueSetDstu3 = 116 (ValueSet) VersionConvertorFactory_30_40.convertResource(theValueSet, new BaseAdvisor_30_40(false)); 117 } catch (FHIRException e) { 118 throw new InternalErrorException(Msg.code(881) + e); 119 } 120 121 if (isBlank(valueSetDstu3.getIdElement().getIdPart())) { 122 String matchUrl = "ValueSet?url=" + UrlUtil.escapeUrlParam(theValueSet.getUrl()); 123 myValueSetResourceDao.update(valueSetDstu3, matchUrl); 124 } else { 125 myValueSetResourceDao.update(valueSetDstu3); 126 } 127 } 128}