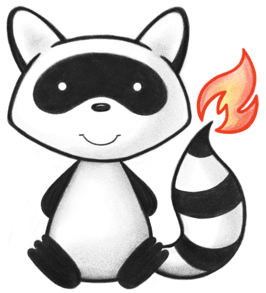
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.api; 021 022import ca.uhn.fhir.jpa.entity.TermCodeSystemVersion; 023import ca.uhn.fhir.jpa.entity.TermConcept; 024import ca.uhn.fhir.jpa.model.entity.ResourceTable; 025import ca.uhn.fhir.jpa.term.UploadStatistics; 026import ca.uhn.fhir.jpa.term.custom.CustomTerminologySet; 027import ca.uhn.fhir.rest.api.server.RequestDetails; 028import org.hl7.fhir.instance.model.api.IIdType; 029import org.hl7.fhir.r4.model.CodeSystem; 030import org.hl7.fhir.r4.model.ValueSet; 031import org.springframework.transaction.annotation.Transactional; 032 033import java.util.List; 034 035/** 036 * This service handles writes to the CodeSystem/Concept tables within the terminology services 037 */ 038public interface ITermCodeSystemStorageSvc { 039 040 String MAKE_LOADING_VERSION_CURRENT = "make.loading.version.current"; 041 042 /** 043 * Defaults to true when parameter is null or entry is not present in requestDetails.myUserData 044 */ 045 static boolean isMakeVersionCurrent(RequestDetails theRequestDetails) { 046 return theRequestDetails == null 047 || (boolean) theRequestDetails.getUserData().getOrDefault(MAKE_LOADING_VERSION_CURRENT, Boolean.TRUE); 048 } 049 050 void storeNewCodeSystemVersion( 051 String theSystemUri, 052 String theSystemName, 053 String theSystemVersionId, 054 TermCodeSystemVersion theCodeSystemVersion, 055 ResourceTable theCodeSystemResourceTable, 056 RequestDetails theRequestDetails); 057 058 /** 059 * Default implementation supports previous signature of method which was added RequestDetails parameter 060 */ 061 @Transactional 062 default void storeNewCodeSystemVersion( 063 String theSystemUri, 064 String theSystemName, 065 String theSystemVersionId, 066 TermCodeSystemVersion theCodeSystemVersion, 067 ResourceTable theCodeSystemResourceTable) { 068 069 storeNewCodeSystemVersion( 070 theSystemUri, 071 theSystemName, 072 theSystemVersionId, 073 theCodeSystemVersion, 074 theCodeSystemResourceTable, 075 null); 076 } 077 078 /** 079 * @return Returns the ID of the created/updated code system 080 */ 081 IIdType storeNewCodeSystemVersion( 082 org.hl7.fhir.r4.model.CodeSystem theCodeSystemResource, 083 TermCodeSystemVersion theCodeSystemVersion, 084 RequestDetails theRequestDetails, 085 List<ValueSet> theValueSets, 086 List<org.hl7.fhir.r4.model.ConceptMap> theConceptMaps); 087 088 void storeNewCodeSystemVersionIfNeeded( 089 CodeSystem theCodeSystem, ResourceTable theResourceEntity, RequestDetails theRequestDetails); 090 091 /** 092 * Default implementation supports previous signature of method which was added RequestDetails parameter 093 */ 094 default void storeNewCodeSystemVersionIfNeeded(CodeSystem theCodeSystem, ResourceTable theResourceEntity) { 095 storeNewCodeSystemVersionIfNeeded(theCodeSystem, theResourceEntity, null); 096 } 097 098 UploadStatistics applyDeltaCodeSystemsAdd(String theSystem, CustomTerminologySet theAdditions); 099 100 UploadStatistics applyDeltaCodeSystemsRemove(String theSystem, CustomTerminologySet theRemovals); 101 102 int saveConcept(TermConcept theNextConcept); 103}