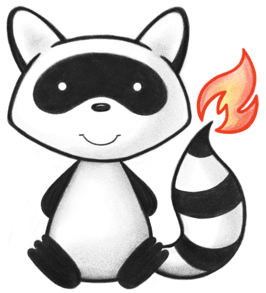
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.api; 021 022import ca.uhn.fhir.jpa.entity.TermCodeSystemVersion; 023import ca.uhn.fhir.jpa.entity.TermConcept; 024import ca.uhn.fhir.jpa.entity.TermConceptParentChildLink; 025import ca.uhn.fhir.jpa.model.entity.ResourceTable; 026import org.hl7.fhir.r4.model.ConceptMap; 027import org.hl7.fhir.r4.model.ValueSet; 028 029import java.util.List; 030 031/** 032 * This service handles processing "deferred" concept writes, meaning concepts that have neen 033 * queued for storage because there are too many of them to handle in a single transaction. 034 */ 035public interface ITermDeferredStorageSvc { 036 037 void saveDeferred(); 038 039 /** 040 * @deprecated Use {@link #isStorageQueueEmpty(boolean)} instead 041 */ 042 @Deprecated 043 default boolean isStorageQueueEmpty() { 044 return isStorageQueueEmpty(true); 045 } 046 047 /** 048 * If you are calling this in a loop or something similar, consider the impact of 049 * setting {@literal theIncludeExecutingJobs} to true. When that parameter is set 050 * to true, each call to this method results in a database lookup! 051 */ 052 boolean isStorageQueueEmpty(boolean theIncludeExecutingJobs); 053 054 /** 055 * This is mostly for unit tests - we can disable processing of deferred concepts 056 * by changing this flag 057 */ 058 void setProcessDeferred(boolean theProcessDeferred); 059 060 void addConceptToStorageQueue(TermConcept theConcept); 061 062 void addConceptLinkToStorageQueue(TermConceptParentChildLink theConceptLink); 063 064 void addConceptMapsToStorageQueue(List<ConceptMap> theConceptMaps); 065 066 void addValueSetsToStorageQueue(List<ValueSet> theValueSets); 067 068 void deleteCodeSystemForResource(ResourceTable theCodeSystemResourceToDelete); 069 070 void deleteCodeSystemVersion(TermCodeSystemVersion theCodeSystemVersion); 071 072 void notifyJobEnded(String theId); 073 074 /** 075 * This is mostly here for unit tests - Saves any and all deferred concepts and links 076 */ 077 void saveAllDeferred(); 078 079 void logQueueForUnitTest(); 080 081 boolean isJobsExecuting(); 082 083 /** 084 * Only to be used from tests - Disallow test timeouts on deferred tasks 085 */ 086 void disallowDeferredTaskTimeout(); 087}