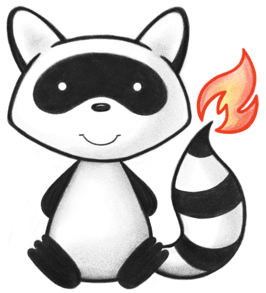
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.api; 021 022import ca.uhn.fhir.context.support.ConceptValidationOptions; 023import ca.uhn.fhir.context.support.IValidationSupport; 024import ca.uhn.fhir.context.support.ValidationSupportContext; 025import ca.uhn.fhir.context.support.ValueSetExpansionOptions; 026import ca.uhn.fhir.jpa.api.dao.IFhirResourceDaoCodeSystem; 027import ca.uhn.fhir.jpa.entity.TermConcept; 028import ca.uhn.fhir.jpa.entity.TermValueSet; 029import ca.uhn.fhir.jpa.model.entity.ResourceTable; 030import ca.uhn.fhir.jpa.term.IValueSetConceptAccumulator; 031import ca.uhn.fhir.rest.api.server.RequestDetails; 032import ca.uhn.fhir.util.FhirVersionIndependentConcept; 033import jakarta.annotation.Nonnull; 034import jakarta.annotation.Nullable; 035import org.hl7.fhir.instance.model.api.IBaseCoding; 036import org.hl7.fhir.instance.model.api.IBaseDatatype; 037import org.hl7.fhir.instance.model.api.IBaseResource; 038import org.hl7.fhir.instance.model.api.IIdType; 039import org.hl7.fhir.instance.model.api.IPrimitiveType; 040import org.hl7.fhir.r4.model.CodeSystem; 041import org.hl7.fhir.r4.model.ValueSet; 042import org.springframework.transaction.annotation.Transactional; 043 044import java.util.List; 045import java.util.Optional; 046import java.util.Set; 047 048/** 049 * This interface is the "read" interface for the terminology service. It handles things like 050 * lookups, code validations, expansions, concept mappings, etc. 051 * <p> 052 * It is intended to only handle read operations, leaving various write operations to 053 * other services within the terminology service APIs. 054 * (Note that at present, a few write operations remain here- they should be moved but haven't 055 * been moved yet) 056 * </p> 057 */ 058public interface ITermReadSvc extends IValidationSupport { 059 060 @Override 061 default String getName() { 062 return getClass().getSimpleName() + " JPA Term Read Service"; 063 } 064 065 ValueSet expandValueSet( 066 @Nullable ValueSetExpansionOptions theExpansionOptions, @Nonnull String theValueSetCanonicalUrl); 067 068 ValueSet expandValueSet( 069 @Nullable ValueSetExpansionOptions theExpansionOptions, @Nonnull ValueSet theValueSetToExpand); 070 071 void expandValueSet( 072 @Nullable ValueSetExpansionOptions theExpansionOptions, 073 ValueSet theValueSetToExpand, 074 IValueSetConceptAccumulator theValueSetCodeAccumulator); 075 076 /** 077 * Version independent 078 */ 079 IBaseResource expandValueSet( 080 @Nullable ValueSetExpansionOptions theExpansionOptions, IBaseResource theValueSetToExpand); 081 082 void expandValueSet( 083 @Nullable ValueSetExpansionOptions theExpansionOptions, 084 IBaseResource theValueSetToExpand, 085 IValueSetConceptAccumulator theValueSetCodeAccumulator); 086 087 List<FhirVersionIndependentConcept> expandValueSetIntoConceptList( 088 ValueSetExpansionOptions theExpansionOptions, String theValueSetCanonicalUrl); 089 090 Optional<TermConcept> findCode(String theCodeSystem, String theCode); 091 092 List<TermConcept> findCodes(String theCodeSystem, List<String> theCodes); 093 094 Set<TermConcept> findCodesAbove( 095 Long theCodeSystemResourcePid, Long theCodeSystemResourceVersionPid, String theCode); 096 097 List<FhirVersionIndependentConcept> findCodesAbove(String theSystem, String theCode); 098 099 List<FhirVersionIndependentConcept> findCodesAboveUsingBuiltInSystems(String theSystem, String theCode); 100 101 Set<TermConcept> findCodesBelow( 102 Long theCodeSystemResourcePid, Long theCodeSystemResourceVersionPid, String theCode); 103 104 List<FhirVersionIndependentConcept> findCodesBelow(String theSystem, String theCode); 105 106 List<FhirVersionIndependentConcept> findCodesBelowUsingBuiltInSystems(String theSystem, String theCode); 107 108 CodeSystem fetchCanonicalCodeSystemFromCompleteContext(String theSystem); 109 110 void deleteValueSetAndChildren(ResourceTable theResourceTable); 111 112 void storeTermValueSet(ResourceTable theResourceTable, ValueSet theValueSet); 113 114 IFhirResourceDaoCodeSystem.SubsumesResult subsumes( 115 IPrimitiveType<String> theCodeA, 116 IPrimitiveType<String> theCodeB, 117 IPrimitiveType<String> theSystem, 118 IBaseCoding theCodingA, 119 IBaseCoding theCodingB); 120 121 void preExpandDeferredValueSetsToTerminologyTables(); 122 123 /** 124 * Version independent 125 */ 126 @Transactional() 127 CodeValidationResult validateCodeIsInPreExpandedValueSet( 128 ValidationSupportContext theValidationSupportContext, 129 ConceptValidationOptions theOptions, 130 IBaseResource theValueSet, 131 String theSystem, 132 String theCode, 133 String theDisplay, 134 IBaseDatatype theCoding, 135 IBaseDatatype theCodeableConcept); 136 137 boolean isValueSetPreExpandedForCodeValidation(ValueSet theValueSet); 138 139 /** 140 * Version independent 141 */ 142 boolean isValueSetPreExpandedForCodeValidation(IBaseResource theValueSet); 143 144 String invalidatePreCalculatedExpansion(IIdType theValueSetId, RequestDetails theRequestDetails); 145 146 /** 147 * Version independent 148 */ 149 Optional<TermValueSet> findCurrentTermValueSet(String theUrl); 150 151 /** 152 * Version independent 153 */ 154 Optional<IBaseResource> readCodeSystemByForcedId(String theForcedId); 155 156 /** 157 * Version independent 158 * Recreates freetext indexes for TermConcept and nested TermConceptProperty 159 */ 160 ReindexTerminologyResult reindexTerminology() throws InterruptedException; 161}