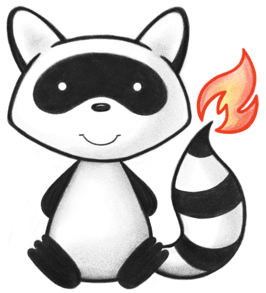
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.loinc; 021 022import ca.uhn.fhir.jpa.entity.TermConcept; 023import ca.uhn.fhir.jpa.term.IZipContentsHandlerCsv; 024import org.apache.commons.csv.CSVRecord; 025 026import java.util.Map; 027 028import static org.apache.commons.lang3.StringUtils.isBlank; 029import static org.apache.commons.lang3.StringUtils.isNotBlank; 030import static org.apache.commons.lang3.StringUtils.trim; 031 032public class LoincAnswerListLinkHandler implements IZipContentsHandlerCsv { 033 034 private final Map<String, TermConcept> myCode2Concept; 035 036 public LoincAnswerListLinkHandler(Map<String, TermConcept> theCode2concept) { 037 myCode2Concept = theCode2concept; 038 } 039 040 @Override 041 public void accept(CSVRecord theRecord) { 042 String applicableContext = trim(theRecord.get("ApplicableContext")); 043 044 /* 045 * Per Dan V's Notes: 046 * 047 * Note: in our current format, we support binding of the same 048 * LOINC term to different answer lists depending on the panel 049 * context. I don?t believe there?s a way to handle that in 050 * the current FHIR spec, so I might suggest we discuss either 051 * only binding the ?default? (non-context specific) list or 052 * if multiple bindings could be supported. 053 */ 054 if (isNotBlank(applicableContext)) { 055 return; 056 } 057 058 String answerListId = trim(theRecord.get("AnswerListId")); 059 if (isBlank(answerListId)) { 060 return; 061 } 062 063 String loincNumber = trim(theRecord.get("LoincNumber")); 064 if (isBlank(loincNumber)) { 065 return; 066 } 067 068 TermConcept loincCode = myCode2Concept.get(loincNumber); 069 if (loincCode != null) { 070 loincCode.addPropertyString("answer-list", answerListId); 071 } 072 073 TermConcept answerListCode = myCode2Concept.get(answerListId); 074 if (answerListCode != null) { 075 answerListCode.addPropertyString("answers-for", loincNumber); 076 } 077 } 078}