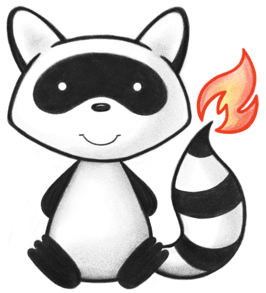
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.loinc; 021 022import ca.uhn.fhir.jpa.entity.TermConcept; 023import ca.uhn.fhir.jpa.term.IZipContentsHandlerCsv; 024import ca.uhn.fhir.jpa.term.api.ITermLoaderSvc; 025import org.apache.commons.csv.CSVRecord; 026import org.hl7.fhir.r4.model.ConceptMap; 027import org.hl7.fhir.r4.model.Enumerations; 028import org.hl7.fhir.r4.model.ValueSet; 029 030import java.util.List; 031import java.util.Map; 032import java.util.Properties; 033 034import static ca.uhn.fhir.jpa.term.loinc.LoincUploadPropertiesEnum.LOINC_CODESYSTEM_VERSION; 035import static ca.uhn.fhir.jpa.term.loinc.LoincUploadPropertiesEnum.LOINC_CONCEPTMAP_VERSION; 036import static org.apache.commons.lang3.StringUtils.trim; 037 038public class LoincIeeeMedicalDeviceCodeHandler extends BaseLoincHandler implements IZipContentsHandlerCsv { 039 040 public static final String LOINC_IEEE_CM_ID = "loinc-to-ieee-11073-10101"; 041 public static final String LOINC_IEEE_CM_URI = "http://loinc.org/cm/loinc-to-ieee-11073-10101"; 042 public static final String LOINC_IEEE_CM_NAME = "LOINC/IEEE Device Code Mappings"; 043 private static final String CM_COPYRIGHT = 044 "The LOINC/IEEE Medical Device Code Mapping Table contains content from IEEE (http://ieee.org), copyright © 2017 IEEE."; 045 046 /** 047 * Constructor 048 */ 049 public LoincIeeeMedicalDeviceCodeHandler( 050 Map<String, TermConcept> theCode2concept, 051 List<ValueSet> theValueSets, 052 List<ConceptMap> theConceptMaps, 053 Properties theUploadProperties, 054 String theCopyrightStatement) { 055 super(theCode2concept, theValueSets, theConceptMaps, theUploadProperties, theCopyrightStatement); 056 } 057 058 @Override 059 public void accept(CSVRecord theRecord) { 060 061 String codeSystemVersionId = myUploadProperties.getProperty(LOINC_CODESYSTEM_VERSION.getCode()); 062 String loincIeeeCmVersion; 063 if (codeSystemVersionId != null) { 064 loincIeeeCmVersion = 065 myUploadProperties.getProperty(LOINC_CONCEPTMAP_VERSION.getCode()) + "-" + codeSystemVersionId; 066 } else { 067 loincIeeeCmVersion = myUploadProperties.getProperty(LOINC_CONCEPTMAP_VERSION.getCode()); 068 } 069 String loincNumber = trim(theRecord.get("LOINC_NUM")); 070 String longCommonName = trim(theRecord.get("LOINC_LONG_COMMON_NAME")); 071 String ieeeCode = trim(theRecord.get("IEEE_CF_CODE10")); 072 String ieeeDisplayName = trim(theRecord.get("IEEE_REFID")); 073 074 // LOINC Part -> IEEE 11073:10101 Mappings 075 String sourceCodeSystemUri = ITermLoaderSvc.LOINC_URI; 076 String targetCodeSystemUri = ITermLoaderSvc.IEEE_11073_10101_URI; 077 String conceptMapId; 078 if (codeSystemVersionId != null) { 079 conceptMapId = LOINC_IEEE_CM_ID + "-" + codeSystemVersionId; 080 } else { 081 conceptMapId = LOINC_IEEE_CM_ID; 082 } 083 addConceptMapEntry( 084 new ConceptMapping() 085 .setConceptMapId(conceptMapId) 086 .setConceptMapUri(LOINC_IEEE_CM_URI) 087 .setConceptMapVersion(loincIeeeCmVersion) 088 .setConceptMapName(LOINC_IEEE_CM_NAME) 089 .setSourceCodeSystem(sourceCodeSystemUri) 090 .setSourceCodeSystemVersion(codeSystemVersionId) 091 .setSourceCode(loincNumber) 092 .setSourceDisplay(longCommonName) 093 .setTargetCodeSystem(targetCodeSystemUri) 094 .setTargetCode(ieeeCode) 095 .setTargetDisplay(ieeeDisplayName) 096 .setEquivalence(Enumerations.ConceptMapEquivalence.EQUAL), 097 myLoincCopyrightStatement + " " + CM_COPYRIGHT); 098 } 099}