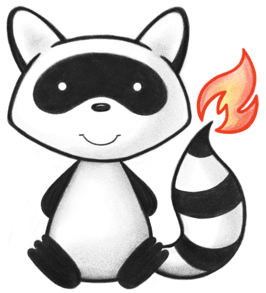
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.loinc; 021 022import ca.uhn.fhir.jpa.entity.TermConcept; 023import ca.uhn.fhir.jpa.term.IZipContentsHandlerCsv; 024import ca.uhn.fhir.jpa.term.api.ITermLoaderSvc; 025import org.apache.commons.csv.CSVRecord; 026 027import java.util.Map; 028 029import static org.apache.commons.lang3.StringUtils.isBlank; 030import static org.apache.commons.lang3.StringUtils.trim; 031import static org.apache.commons.lang3.StringUtils.trimToEmpty; 032 033public class LoincLinguisticVariantHandler implements IZipContentsHandlerCsv { 034 035 private final Map<String, TermConcept> myCode2Concept; 036 private final String myLanguageCode; 037 038 public LoincLinguisticVariantHandler(Map<String, TermConcept> theCode2Concept, String theLanguageCode) { 039 myCode2Concept = theCode2Concept; 040 myLanguageCode = theLanguageCode; 041 } 042 043 @Override 044 public void accept(CSVRecord theRecord) { 045 046 String loincNumber = trim(theRecord.get("LOINC_NUM")); 047 if (isBlank(loincNumber)) { 048 return; 049 } 050 051 TermConcept concept = myCode2Concept.get(loincNumber); 052 if (concept == null) { 053 return; 054 } 055 056 // The following should be created as designations for each term: 057 // COMPONENT:PROPERTY:TIME_ASPCT:SYSTEM:SCALE_TYP:METHOD_TYP (as colon-separated concatenation - FormalName) 058 // SHORTNAME 059 // LONG_COMMON_NAME 060 // LinguisticVariantDisplayName 061 062 // -- add formalName designation 063 StringBuilder fullySpecifiedName = new StringBuilder(); 064 fullySpecifiedName.append(trimToEmpty(theRecord.get("COMPONENT") + ":")); 065 fullySpecifiedName.append(trimToEmpty(theRecord.get("PROPERTY") + ":")); 066 fullySpecifiedName.append(trimToEmpty(theRecord.get("TIME_ASPCT") + ":")); 067 fullySpecifiedName.append(trimToEmpty(theRecord.get("SYSTEM") + ":")); 068 fullySpecifiedName.append(trimToEmpty(theRecord.get("SCALE_TYP") + ":")); 069 fullySpecifiedName.append(trimToEmpty(theRecord.get("METHOD_TYP"))); 070 071 String fullySpecifiedNameStr = fullySpecifiedName.toString(); 072 073 // skip if COMPONENT, PROPERTY, TIME_ASPCT, SYSTEM, SCALE_TYP and METHOD_TYP are all empty 074 if (!fullySpecifiedNameStr.equals(":::::")) { 075 concept.addDesignation() 076 .setLanguage(myLanguageCode) 077 .setUseSystem(ITermLoaderSvc.LOINC_URI) 078 .setUseCode("FullySpecifiedName") 079 .setUseDisplay("FullySpecifiedName") 080 .setValue(fullySpecifiedNameStr); 081 } 082 083 // -- other designations 084 addDesignation(theRecord, concept, "SHORTNAME"); 085 addDesignation(theRecord, concept, "LONG_COMMON_NAME"); 086 addDesignation(theRecord, concept, "LinguisticVariantDisplayName"); 087 } 088 089 private void addDesignation(CSVRecord theRecord, TermConcept concept, String fieldName) { 090 091 String field = trim(theRecord.get(fieldName)); 092 if (isBlank(field)) { 093 return; 094 } 095 096 concept.addDesignation() 097 .setLanguage(myLanguageCode) 098 .setUseSystem(ITermLoaderSvc.LOINC_URI) 099 .setUseCode(fieldName) 100 .setUseDisplay(fieldName) 101 .setValue(field); 102 } 103}