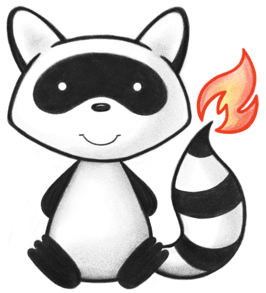
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.loinc; 021 022import ca.uhn.fhir.jpa.entity.TermConcept; 023import ca.uhn.fhir.jpa.term.IZipContentsHandlerCsv; 024import ca.uhn.fhir.jpa.term.api.ITermLoaderSvc; 025import org.apache.commons.csv.CSVRecord; 026import org.slf4j.Logger; 027import org.slf4j.LoggerFactory; 028 029import java.util.Map; 030 031import static org.apache.commons.lang3.StringUtils.isBlank; 032import static org.apache.commons.lang3.StringUtils.trim; 033 034/** 035 * Handles addition of MAP_TO properties to TermConcepts 036 */ 037public class LoincMapToHandler implements IZipContentsHandlerCsv { 038 private static final Logger ourLog = LoggerFactory.getLogger(LoincMapToHandler.class); 039 040 public static final String CONCEPT_CODE_PROP_NAME = "LOINC"; 041 public static final String MAP_TO_PROP_NAME = "MAP_TO"; 042 public static final String DISPLAY_PROP_NAME = "COMMENT"; 043 044 private final Map<String, TermConcept> myCode2Concept; 045 046 public LoincMapToHandler(Map<String, TermConcept> theCode2concept) { 047 myCode2Concept = theCode2concept; 048 } 049 050 @Override 051 public void accept(CSVRecord theRecord) { 052 String code = trim(theRecord.get(CONCEPT_CODE_PROP_NAME)); 053 String mapTo = trim(theRecord.get(MAP_TO_PROP_NAME)); 054 String display = trim(theRecord.get(DISPLAY_PROP_NAME)); 055 056 if (isBlank(code)) { 057 ourLog.warn("MapTo record was found with a blank '" + CONCEPT_CODE_PROP_NAME + "' property"); 058 return; 059 } 060 061 if (isBlank(mapTo)) { 062 ourLog.warn("MapTo record was found with a blank '" + MAP_TO_PROP_NAME + "' property"); 063 return; 064 } 065 066 TermConcept concept = myCode2Concept.get(code); 067 if (concept == null) { 068 ourLog.warn("A TermConcept was not found for MapTo '" + CONCEPT_CODE_PROP_NAME + "' property: '" + code 069 + "' MapTo record ignored."); 070 return; 071 } 072 073 concept.addPropertyCoding(MAP_TO_PROP_NAME, ITermLoaderSvc.LOINC_URI, mapTo, display); 074 ourLog.trace( 075 "Adding " + MAP_TO_PROP_NAME + " coding property: {} to concept.code {}", mapTo, concept.getCode()); 076 } 077}