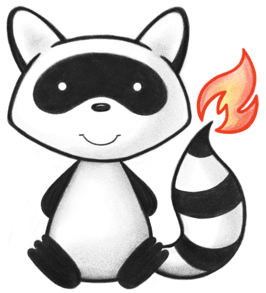
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.loinc; 021 022import java.util.Collections; 023import java.util.HashMap; 024import java.util.Map; 025 026/** 027 * This enum is used to facilitate configurable filenames when uploading LOINC. 028 */ 029public enum LoincUploadPropertiesEnum { 030 /** 031 * Sorting agnostic. 032 */ 033 LOINC_UPLOAD_PROPERTIES_FILE("loincupload.properties"), 034 LOINC_XML_FILE("loinc.xml"), 035 036 LOINC_MAPTO_FILE("loinc.mapto.file"), 037 LOINC_MAPTO_FILE_DEFAULT("LoincTable/MapTo.csv"), 038 039 /* 040 * MANDATORY 041 */ 042 // Answer lists (ValueSets of potential answers/values for LOINC "questions") 043 LOINC_ANSWERLIST_FILE("loinc.answerlist.file"), 044 LOINC_ANSWERLIST_FILE_DEFAULT("AccessoryFiles/AnswerFile/AnswerList.csv"), 045 // Answer list links (connects LOINC observation codes to answer list codes) 046 LOINC_ANSWERLIST_LINK_FILE("loinc.answerlist.link.file"), 047 LOINC_ANSWERLIST_LINK_FILE_DEFAULT("AccessoryFiles/AnswerFile/LoincAnswerListLink.csv"), 048 049 // Document ontology 050 LOINC_DOCUMENT_ONTOLOGY_FILE("loinc.document.ontology.file"), 051 LOINC_DOCUMENT_ONTOLOGY_FILE_DEFAULT("AccessoryFiles/DocumentOntology/DocumentOntology.csv"), 052 053 // LOINC codes 054 LOINC_FILE("loinc.file"), 055 LOINC_FILE_DEFAULT("LoincTable/Loinc.csv"), 056 057 // LOINC hierarchy 058 LOINC_HIERARCHY_FILE("loinc.hierarchy.file"), 059 LOINC_HIERARCHY_FILE_DEFAULT("AccessoryFiles/MultiAxialHierarchy/MultiAxialHierarchy.csv"), 060 061 // IEEE medical device codes 062 LOINC_IEEE_MEDICAL_DEVICE_CODE_MAPPING_TABLE_FILE("loinc.ieee.medical.device.code.mapping.table.file"), 063 LOINC_IEEE_MEDICAL_DEVICE_CODE_MAPPING_TABLE_FILE_DEFAULT( 064 "AccessoryFiles/LoincIeeeMedicalDeviceCodeMappingTable/LoincIeeeMedicalDeviceCodeMappingTable.csv"), 065 066 // Imaging document codes 067 LOINC_IMAGING_DOCUMENT_CODES_FILE("loinc.imaging.document.codes.file"), 068 LOINC_IMAGING_DOCUMENT_CODES_FILE_DEFAULT("AccessoryFiles/ImagingDocuments/ImagingDocumentCodes.csv"), 069 070 // Part 071 LOINC_PART_FILE("loinc.part.file"), 072 LOINC_PART_FILE_DEFAULT("AccessoryFiles/PartFile/Part.csv"), 073 074 // Part link 075 LOINC_PART_LINK_FILE("loinc.part.link.file"), 076 LOINC_PART_LINK_FILE_DEFAULT("AccessoryFiles/PartFile/LoincPartLink.csv"), 077 LOINC_PART_LINK_FILE_PRIMARY("loinc.part.link.primary.file"), 078 LOINC_PART_LINK_FILE_PRIMARY_DEFAULT("AccessoryFiles/PartFile/LoincPartLink_Primary.csv"), 079 LOINC_PART_LINK_FILE_SUPPLEMENTARY("loinc.part.link.supplementary.file"), 080 LOINC_PART_LINK_FILE_SUPPLEMENTARY_DEFAULT("AccessoryFiles/PartFile/LoincPartLink_Supplementary.csv"), 081 082 // Part related code mapping 083 LOINC_PART_RELATED_CODE_MAPPING_FILE("loinc.part.related.code.mapping.file"), 084 LOINC_PART_RELATED_CODE_MAPPING_FILE_DEFAULT("AccessoryFiles/PartFile/PartRelatedCodeMapping.csv"), 085 086 // RSNA playbook 087 LOINC_RSNA_PLAYBOOK_FILE("loinc.rsna.playbook.file"), 088 LOINC_RSNA_PLAYBOOK_FILE_DEFAULT("AccessoryFiles/LoincRsnaRadiologyPlaybook/LoincRsnaRadiologyPlaybook.csv"), 089 090 // Top 2000 codes - SI 091 LOINC_TOP2000_COMMON_LAB_RESULTS_SI_FILE("loinc.top2000.common.lab.results.si.file"), 092 LOINC_TOP2000_COMMON_LAB_RESULTS_SI_FILE_DEFAULT("AccessoryFiles/Top2000Results/SI/Top2000CommonLabResultsSi.csv"), 093 // Top 2000 codes - US 094 LOINC_TOP2000_COMMON_LAB_RESULTS_US_FILE("loinc.top2000.common.lab.results.us.file"), 095 LOINC_TOP2000_COMMON_LAB_RESULTS_US_FILE_DEFAULT("AccessoryFiles/Top2000Results/US/Top2000CommonLabResultsUs.csv"), 096 097 // Universal lab order ValueSet 098 LOINC_UNIVERSAL_LAB_ORDER_VALUESET_FILE("loinc.universal.lab.order.valueset.file"), 099 LOINC_UNIVERSAL_LAB_ORDER_VALUESET_FILE_DEFAULT( 100 "AccessoryFiles/LoincUniversalLabOrdersValueSet/LoincUniversalLabOrdersValueSet.csv"), 101 102 /* 103 * OPTIONAL 104 */ 105 // This is the version identifier for the LOINC code system 106 LOINC_CODESYSTEM_VERSION("loinc.codesystem.version"), 107 108 // Indicates if loading version has to become current 109 LOINC_CODESYSTEM_MAKE_CURRENT("loinc.codesystem.make.current"), 110 111 // This is the version identifier for the answer list file 112 LOINC_ANSWERLIST_VERSION("loinc.answerlist.version"), 113 114 // This is the version identifier for uploaded ConceptMap resources 115 LOINC_CONCEPTMAP_VERSION("loinc.conceptmap.version"), 116 117 // Group 118 LOINC_GROUP_FILE("loinc.group.file"), 119 LOINC_GROUP_FILE_DEFAULT("AccessoryFiles/GroupFile/Group.csv"), 120 // Group terms 121 LOINC_GROUP_TERMS_FILE("loinc.group.terms.file"), 122 LOINC_GROUP_TERMS_FILE_DEFAULT("AccessoryFiles/GroupFile/GroupLoincTerms.csv"), 123 // Parent group 124 LOINC_PARENT_GROUP_FILE("loinc.parent.group.file"), 125 LOINC_PARENT_GROUP_FILE_DEFAULT("AccessoryFiles/GroupFile/ParentGroup.csv"), 126 127 // Consumer Name 128 LOINC_CONSUMER_NAME_FILE("loinc.consumer.name.file"), 129 LOINC_CONSUMER_NAME_FILE_DEFAULT("AccessoryFiles/ConsumerName/ConsumerName.csv"), 130 131 // Linguistic Variants 132 LOINC_LINGUISTIC_VARIANTS_FILE("loinc.linguistic.variants.file"), 133 LOINC_LINGUISTIC_VARIANTS_FILE_DEFAULT("AccessoryFiles/LinguisticVariants/LinguisticVariants.csv"), 134 135 // Linguistic Variants Folder Path which contains variants for different languages 136 LOINC_LINGUISTIC_VARIANTS_PATH("loinc.linguistic.variants.path"), 137 LOINC_LINGUISTIC_VARIANTS_PATH_DEFAULT("AccessoryFiles/LinguisticVariants/"), 138 139 /* 140 * DUPLICATES 141 */ 142 LOINC_DUPLICATE_FILE_DEFAULT("AccessoryFiles/PanelsAndForms/Loinc.csv"), 143 LOINC_ANSWERLIST_DUPLICATE_FILE_DEFAULT("AccessoryFiles/PanelsAndForms/AnswerList.csv"), 144 LOINC_ANSWERLIST_LINK_DUPLICATE_FILE_DEFAULT("AccessoryFiles/PanelsAndForms/LoincAnswerListLink.csv"); 145 146 private static Map<String, LoincUploadPropertiesEnum> ourValues; 147 private String myCode; 148 149 LoincUploadPropertiesEnum(String theCode) { 150 myCode = theCode; 151 } 152 153 public String getCode() { 154 return myCode; 155 } 156 157 public static LoincUploadPropertiesEnum fromCode(String theCode) { 158 if (ourValues == null) { 159 HashMap<String, LoincUploadPropertiesEnum> values = new HashMap<>(); 160 for (LoincUploadPropertiesEnum next : values()) { 161 values.put(next.getCode(), next); 162 } 163 ourValues = Collections.unmodifiableMap(values); 164 } 165 return ourValues.get(theCode); 166 } 167 168 /** 169 * Convert from Enum ordinal to Enum type. 170 * 171 * Usage: 172 * 173 * <code>LoincUploadPropertiesEnum loincUploadPropertiesEnum = LoincUploadPropertiesEnum.values[ordinal];</code> 174 */ 175 public static final LoincUploadPropertiesEnum values[] = values(); 176}