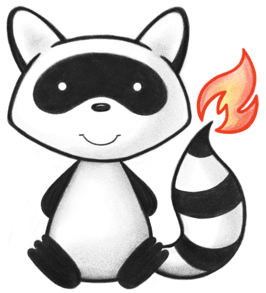
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.snomedct; 021 022import ca.uhn.fhir.jpa.entity.TermCodeSystemVersion; 023import ca.uhn.fhir.jpa.entity.TermConcept; 024import ca.uhn.fhir.jpa.term.IZipContentsHandlerCsv; 025import ca.uhn.fhir.jpa.term.TermLoaderSvcImpl; 026import org.apache.commons.csv.CSVRecord; 027 028import java.util.Map; 029import java.util.Set; 030 031public final class SctHandlerDescription implements IZipContentsHandlerCsv { 032 private final Map<String, TermConcept> myCode2concept; 033 private final TermCodeSystemVersion myCodeSystemVersion; 034 private final Map<String, TermConcept> myId2concept; 035 private Set<String> myValidConceptIds; 036 037 public SctHandlerDescription( 038 Set<String> theValidConceptIds, 039 Map<String, TermConcept> theCode2concept, 040 Map<String, TermConcept> theId2concept, 041 TermCodeSystemVersion theCodeSystemVersion) { 042 myCode2concept = theCode2concept; 043 myId2concept = theId2concept; 044 myCodeSystemVersion = theCodeSystemVersion; 045 myValidConceptIds = theValidConceptIds; 046 } 047 048 @Override 049 public void accept(CSVRecord theRecord) { 050 String id = theRecord.get("id"); 051 boolean active = "1".equals(theRecord.get("active")); 052 if (!active) { 053 return; 054 } 055 String conceptId = theRecord.get("conceptId"); 056 if (!myValidConceptIds.contains(conceptId)) { 057 return; 058 } 059 060 String term = theRecord.get("term"); 061 062 TermConcept concept = TermLoaderSvcImpl.getOrCreateConcept(myId2concept, id); 063 concept.setCode(conceptId); 064 concept.setDisplay(term); 065 concept.setCodeSystemVersion(myCodeSystemVersion); 066 myCode2concept.put(conceptId, concept); 067 } 068}