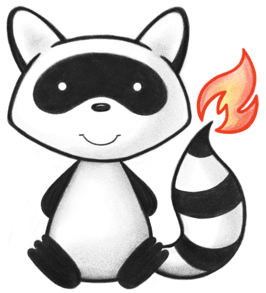
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.util; 021 022import org.apache.commons.lang3.Validate; 023import org.hibernate.ScrollableResults; 024 025import java.io.Closeable; 026import java.util.Iterator; 027 028public class ScrollableResultsIterator<T extends Object> extends BaseIterator<T> implements Iterator<T>, Closeable { 029 private boolean hasNext; 030 private T myNext; 031 private ScrollableResults myScroll; 032 033 public ScrollableResultsIterator(ScrollableResults theScroll) { 034 myScroll = theScroll; 035 } 036 037 @SuppressWarnings("unchecked") 038 private void ensureHaveNext() { 039 if (myNext == null) { 040 if (myScroll.next()) { 041 hasNext = true; 042 myNext = (T) myScroll.get(); 043 } else { 044 hasNext = false; 045 } 046 } 047 } 048 049 @Override 050 public boolean hasNext() { 051 ensureHaveNext(); 052 return hasNext; 053 } 054 055 @Override 056 public T next() { 057 ensureHaveNext(); 058 Validate.isTrue(hasNext); 059 T next = myNext; 060 myNext = null; 061 return next; 062 } 063 064 @Override 065 public void close() { 066 if (myScroll != null) { 067 myScroll.close(); 068 myScroll = null; 069 } 070 } 071}