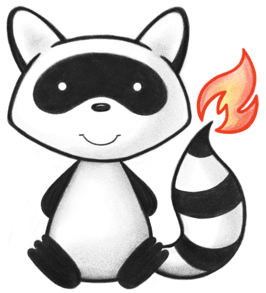
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020 021package ca.uhn.fhir.jpa.util; 022 023import org.springframework.aop.framework.Advised; 024import org.springframework.aop.support.AopUtils; 025 026/** 027 * Utility to get the Spring proxy object's target object 028 */ 029public class SpringObjectCaster { 030 031 /** 032 * Retrieve the Spring proxy object's target object 033 * @param proxy 034 * @param clazz 035 * @param <T> 036 * @return 037 * @throws Exception 038 */ 039 public static <T> T getTargetObject(Object proxy, Class<T> clazz) throws Exception { 040 while ((AopUtils.isJdkDynamicProxy(proxy))) { 041 return clazz.cast( 042 getTargetObject(((Advised) proxy).getTargetSource().getTarget(), clazz)); 043 } 044 045 return clazz.cast(proxy); 046 } 047}