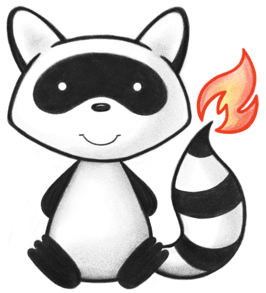
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.util; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.interceptor.api.Hook; 024import ca.uhn.fhir.interceptor.api.Interceptor; 025import ca.uhn.fhir.interceptor.api.Pointcut; 026import ca.uhn.fhir.jpa.api.dao.IFhirResourceDao; 027import ca.uhn.fhir.model.dstu2.valueset.ResourceTypeEnum; 028import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 029import ca.uhn.fhir.rest.api.server.RequestDetails; 030import ca.uhn.fhir.rest.server.exceptions.ResourceNotFoundException; 031import ca.uhn.fhir.rest.server.exceptions.UnprocessableEntityException; 032import org.hl7.fhir.instance.model.api.IBaseResource; 033import org.hl7.fhir.r4.model.Subscription; 034import org.hl7.fhir.r4.model.Subscription.SubscriptionChannelType; 035import org.hl7.fhir.r4.model.Subscription.SubscriptionStatus; 036import org.springframework.beans.factory.annotation.Autowired; 037import org.springframework.beans.factory.annotation.Qualifier; 038 039import static ca.uhn.fhir.subscription.SubscriptionConstants.ORDER_SUBSCRIPTION_ACTIVATING; 040import static org.apache.commons.lang3.StringUtils.isNotBlank; 041 042/** 043 * Interceptor which requires newly created {@link Subscription subscriptions} to be in 044 * {@link SubscriptionStatus#REQUESTED} state and prevents clients from changing the status. 045 */ 046@Interceptor 047public class SubscriptionsRequireManualActivationInterceptorR4 { 048 049 @Autowired 050 @Qualifier("mySubscriptionDaoR4") 051 private IFhirResourceDao<Subscription> myDao; 052 053 @Hook(value = Pointcut.STORAGE_PRESTORAGE_RESOURCE_CREATED, order = ORDER_SUBSCRIPTION_ACTIVATING) 054 public void resourceCreated(RequestDetails theRequest, IBaseResource theResource) { 055 if (myDao.getContext().getResourceType(theResource).equals(ResourceTypeEnum.SUBSCRIPTION.getCode())) { 056 verifyStatusOk(RestOperationTypeEnum.CREATE, null, theResource); 057 } 058 } 059 060 @Hook(value = Pointcut.STORAGE_PRESTORAGE_RESOURCE_UPDATED, order = ORDER_SUBSCRIPTION_ACTIVATING) 061 public void resourceUpdated(RequestDetails theRequest, IBaseResource theOldResource, IBaseResource theNewResource) { 062 if (myDao.getContext().getResourceType(theNewResource).equals(ResourceTypeEnum.SUBSCRIPTION.getCode())) { 063 verifyStatusOk(RestOperationTypeEnum.UPDATE, theOldResource, theNewResource); 064 } 065 } 066 067 public void setDao(IFhirResourceDao<Subscription> theDao) { 068 myDao = theDao; 069 } 070 071 private void verifyStatusOk( 072 RestOperationTypeEnum theOperation, IBaseResource theOldResourceOrNull, IBaseResource theResource) { 073 Subscription subscription = (Subscription) theResource; 074 SubscriptionStatus newStatus = subscription.getStatusElement().getValue(); 075 076 if (newStatus == SubscriptionStatus.REQUESTED || newStatus == SubscriptionStatus.OFF) { 077 return; 078 } 079 080 if (newStatus == null) { 081 String actualCode = subscription.getStatusElement().getValueAsString(); 082 throw new UnprocessableEntityException(Msg.code(807) + "Can not " + theOperation.getCode() 083 + " resource: Subscription.status must be populated on this server" 084 + ((isNotBlank(actualCode)) ? " (invalid value " + actualCode + ")" : "")); 085 } 086 087 if (theOldResourceOrNull != null) { 088 try { 089 Subscription existing = (Subscription) theOldResourceOrNull; 090 SubscriptionStatus existingStatus = existing.getStatusElement().getValue(); 091 if (existingStatus != newStatus) { 092 verifyActiveStatus(theOperation, subscription, newStatus, existingStatus); 093 } 094 } catch (ResourceNotFoundException e) { 095 verifyActiveStatus(theOperation, subscription, newStatus, null); 096 } 097 } else { 098 verifyActiveStatus(theOperation, subscription, newStatus, null); 099 } 100 } 101 102 private void verifyActiveStatus( 103 RestOperationTypeEnum theOperation, 104 Subscription theSubscription, 105 SubscriptionStatus newStatus, 106 SubscriptionStatus theExistingStatus) { 107 SubscriptionChannelType channelType = 108 theSubscription.getChannel().getTypeElement().getValue(); 109 110 if (channelType == null) { 111 throw new UnprocessableEntityException(Msg.code(808) + "Subscription.channel.type must be populated"); 112 } 113 114 if (channelType == SubscriptionChannelType.WEBSOCKET) { 115 return; 116 } 117 118 if (theExistingStatus != null) { 119 throw new UnprocessableEntityException(Msg.code(809) + "Subscription.status can not be changed from " 120 + describeStatus(theExistingStatus) + " to " + describeStatus(newStatus)); 121 } 122 123 if (theSubscription.getStatus() == null) { 124 throw new UnprocessableEntityException( 125 Msg.code(810) + "Can not " + theOperation.getCode().toLowerCase() 126 + " resource: Subscription.status must be populated on this server"); 127 } 128 129 throw new UnprocessableEntityException( 130 Msg.code(811) + "Subscription.status must be '" + SubscriptionStatus.OFF.toCode() + "' or '" 131 + SubscriptionStatus.REQUESTED.toCode() + "' on a newly created subscription"); 132 } 133 134 private String describeStatus(SubscriptionStatus existingStatus) { 135 String existingStatusString; 136 if (existingStatus != null) { 137 existingStatusString = '\'' + existingStatus.toCode() + '\''; 138 } else { 139 existingStatusString = "null"; 140 } 141 return existingStatusString; 142 } 143}