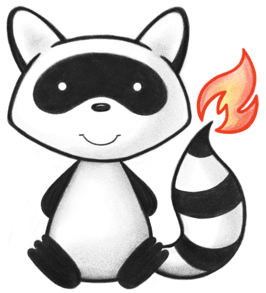
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.validation; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.support.IValidationSupport; 024import ca.uhn.fhir.jpa.config.JpaConfig; 025import ca.uhn.fhir.jpa.packages.NpmJpaValidationSupport; 026import ca.uhn.fhir.jpa.term.api.ITermConceptMappingSvc; 027import ca.uhn.fhir.jpa.term.api.ITermReadSvc; 028import jakarta.annotation.PostConstruct; 029import jakarta.annotation.PreDestroy; 030import org.hl7.fhir.common.hapi.validation.support.CommonCodeSystemsTerminologyService; 031import org.hl7.fhir.common.hapi.validation.support.InMemoryTerminologyServerValidationSupport; 032import org.hl7.fhir.common.hapi.validation.support.SnapshotGeneratingValidationSupport; 033import org.hl7.fhir.common.hapi.validation.support.UnknownCodeSystemWarningValidationSupport; 034import org.hl7.fhir.common.hapi.validation.support.ValidationSupportChain; 035import org.springframework.beans.factory.annotation.Autowired; 036import org.springframework.beans.factory.annotation.Qualifier; 037 038public class JpaValidationSupportChain extends ValidationSupportChain { 039 040 private final FhirContext myFhirContext; 041 042 @Autowired 043 @Qualifier(JpaConfig.JPA_VALIDATION_SUPPORT) 044 public IValidationSupport myJpaValidationSupport; 045 046 @Qualifier("myDefaultProfileValidationSupport") 047 @Autowired 048 private IValidationSupport myDefaultProfileValidationSupport; 049 050 @Autowired 051 private ITermReadSvc myTerminologyService; 052 053 @Autowired 054 private NpmJpaValidationSupport myNpmJpaValidationSupport; 055 056 @Autowired 057 private ITermConceptMappingSvc myConceptMappingSvc; 058 059 @Autowired 060 private UnknownCodeSystemWarningValidationSupport myUnknownCodeSystemWarningValidationSupport; 061 062 @Autowired 063 private InMemoryTerminologyServerValidationSupport myInMemoryTerminologyServerValidationSupport; 064 065 /** 066 * Constructor 067 */ 068 public JpaValidationSupportChain( 069 FhirContext theFhirContext, ValidationSupportChain.CacheConfiguration theCacheConfiguration) { 070 super(theCacheConfiguration); 071 072 assert theFhirContext != null; 073 assert theCacheConfiguration != null; 074 075 myFhirContext = theFhirContext; 076 } 077 078 @Override 079 public FhirContext getFhirContext() { 080 return myFhirContext; 081 } 082 083 @PreDestroy 084 public void flush() { 085 invalidateCaches(); 086 } 087 088 @PostConstruct 089 public void postConstruct() { 090 addValidationSupport(myDefaultProfileValidationSupport); 091 addValidationSupport(myJpaValidationSupport); 092 addValidationSupport(myTerminologyService); 093 addValidationSupport(new SnapshotGeneratingValidationSupport(myFhirContext)); 094 addValidationSupport(myInMemoryTerminologyServerValidationSupport); 095 addValidationSupport(myNpmJpaValidationSupport); 096 addValidationSupport(new CommonCodeSystemsTerminologyService(myFhirContext)); 097 addValidationSupport(myConceptMappingSvc); 098 099 // This needs to be last in the chain, it was designed for that 100 addValidationSupport(myUnknownCodeSystemWarningValidationSupport); 101 } 102}